Scala: Overcoming Type Safety Challenges
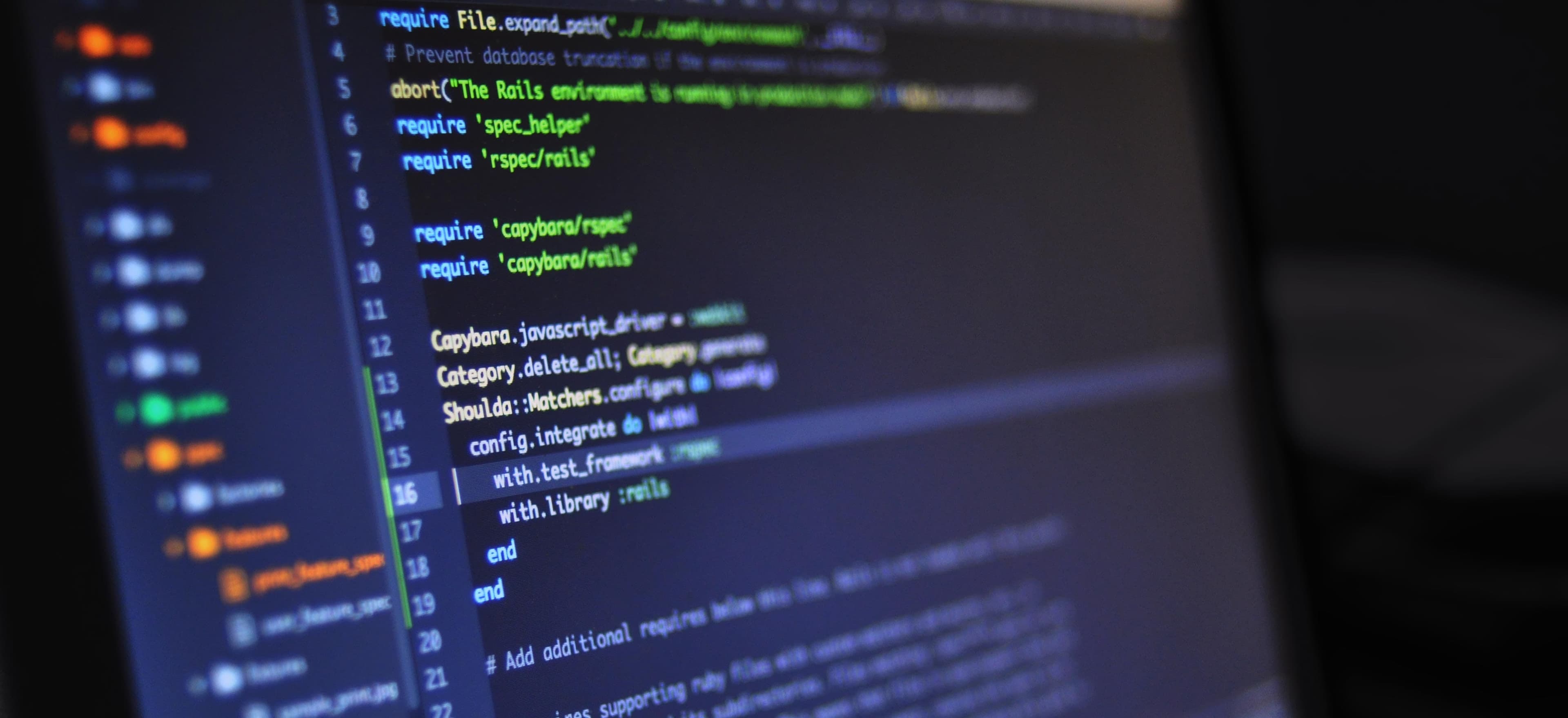
- Published on
Scala: Overcoming Type Safety Challenges
When it comes to building robust and maintainable software systems, type safety is a crucial element. In the realm of Java programming, Scala has emerged as a powerful language that provides a rich set of features for ensuring type safety. In this post, we'll explore the various challenges related to type safety in Java and how Scala offers solutions to overcome them.
Understanding Type Safety in Java
In Java, type safety is enforced by the compiler, ensuring that operations are performed only on compatible types. While Java's type system is robust, there are still certain challenges that developers encounter when dealing with complex data structures and dynamic behaviors.
One of the main issues in Java is the verbosity of defining generic types, which can lead to code duplication and reduced readability. Additionally, Java's type erasure mechanism can result in runtime errors when working with generic types.
Scala's Approach to Type Safety
Scala, being a hybrid functional and object-oriented language, provides a more expressive type system compared to Java. It offers advanced features such as type inference, variance annotations, and pattern matching, which contribute to higher levels of type safety and code conciseness.
Let's delve into some of the ways Scala addresses the type safety challenges prevalent in Java.
Type Inference
Scala's powerful type inference mechanism allows developers to omit explicit type annotations in many cases, reducing boilerplate code and enhancing readability. This feature not only streamlines the development process but also minimizes the chances of type-related errors.
// Scala type inference in action
val number = 42 // Compiler infers 'number' as Int
val greeting = "Hello, Scala!" // Compiler infers 'greeting' as String
Variance Annotations
In Java, dealing with covariance and contravariance can be cumbersome, often leading to code complexity. Scala's variance annotations (+
for covariance and -
for contravariance) provide a concise way of defining type relationships, leading to safer and more intuitive APIs.
// Scala variance annotations for type safety
trait Animal
class Dog extends Animal
class Cat extends Animal
// Covariance example
class CovariantList[+A]
val animalList: CovariantList[Animal] = new CovariantList[Dog]
// Contravariance example
class ContravariantList[-A]
val dogList: ContravariantList[Dog] = new ContravariantList[Animal]
Pattern Matching
Scala's pattern matching offers a powerful way to perform type-safe, exhaustive checks on data. This feature can significantly reduce the occurrence of runtime type errors and enhance code robustness.
// Scala pattern matching for type safety
def matchAnimal(animal: Animal): String = animal match {
case _: Dog => "It's a dog"
case _: Cat => "It's a cat"
}
Leveraging Functional Programming for Type Safety
Scala's functional programming capabilities further contribute to type safety by promoting immutable data structures, referential transparency, and algebraic data types. These principles help in creating more predictable and error-resistant code, reducing the likelihood of type-related bugs.
Algebraic Data Types
Algebraic data types, including sealed traits
and case classes
, are fundamental to Scala's type safety model. They allow developers to define comprehensive and exhaustive data structures in a type-safe manner, enabling compiler assistance in pattern matching.
// Scala algebraic data types for type safety
sealed trait Shape
case class Circle(radius: Double) extends Shape
case class Square(sideLength: Double) extends Shape
Immutability and Referential Transparency
By default, Scala encourages immutability and pure functions, which eliminates the risk of unexpected type mutations and side effects. This approach fosters a more predictable and understandable codebase, contributing to overall system reliability.
// Scala immutability for type safety
val immutableList = List(1, 2, 3) // 'immutableList' cannot be mutated
def add(x: Int, y: Int): Int = x + y // 'add' is referentially transparent
Embracing Advanced Type System Features
Scala's advanced type system empowers developers to express complex relationships between types, thereby fortifying the code against many type-related pitfalls. Features such as path-dependent types, type classes, and self-types play a pivotal role in achieving a higher degree of type safety.
Path-Dependent Types
Path-dependent types in Scala allow for type projection based on the path of an instance, enabling fine-grained control over the relationships between types. This feature aids in constructing more precise and type-safe abstractions.
// Scala path-dependent types for type safety
class Container {
class Content
}
def processContent(container: Container)(content: container.Content): Unit = {
// Process 'content' in a type-safe manner
}
Type Classes
Type classes in Scala enable ad-hoc polymorphism, providing a powerful mechanism to define behaviors for types in a type-safe and extensible manner. This approach allows for the implementation of generic algorithms without compromising type safety.
// Scala type classes for type safety
trait Printable[T] {
def print(value: T): String
}
object PrintableInstances {
implicit val printableString: Printable[String] = (value: String) => value
implicit val printableInt: Printable[Int] = (value: Int) => value.toString
}
Self-Types
Self-types in Scala allow a class to declare its dependencies explicitly, ensuring the presence of required members from another type. This feature enforces type safety by specifying dependencies in a clear and concise manner.
// Scala self-types for type safety
trait UserService {
def getUserById(id: Long): User
}
trait UserRepository {
this: UserService =>
// Implementations with access to 'getUserById' method
}
A Final Look
In the realm of type safety, Scala stands out as a language that offers a myriad of features and techniques to mitigate common challenges encountered in Java. By leveraging advanced type system features, functional programming principles, and concise syntax, Scala empowers developers to build robust and type-safe systems with confidence.
Incorporating Scala's type safety best practices not only leads to fewer runtime errors but also promotes code clarity, maintainability, and extensibility. As the software industry continues to embrace type-safe paradigms, Scala emerges as a compelling choice for tackling the evolving demands of modern software development.
In conclusion, Scala's comprehensive approach to type safety serves as a testament to its prowess in addressing the complexities of type-related challenges, making it a formidable language for building resilient and dependable software systems.
For more in-depth insights into Scala and its type safety features, explore Scala Documentation.
Remember, when it comes to type safety, Scala is not just a language; it's a paradigm shift towards robust and maintainable software development.