Choosing the Best Free Weather API for Your Weather App
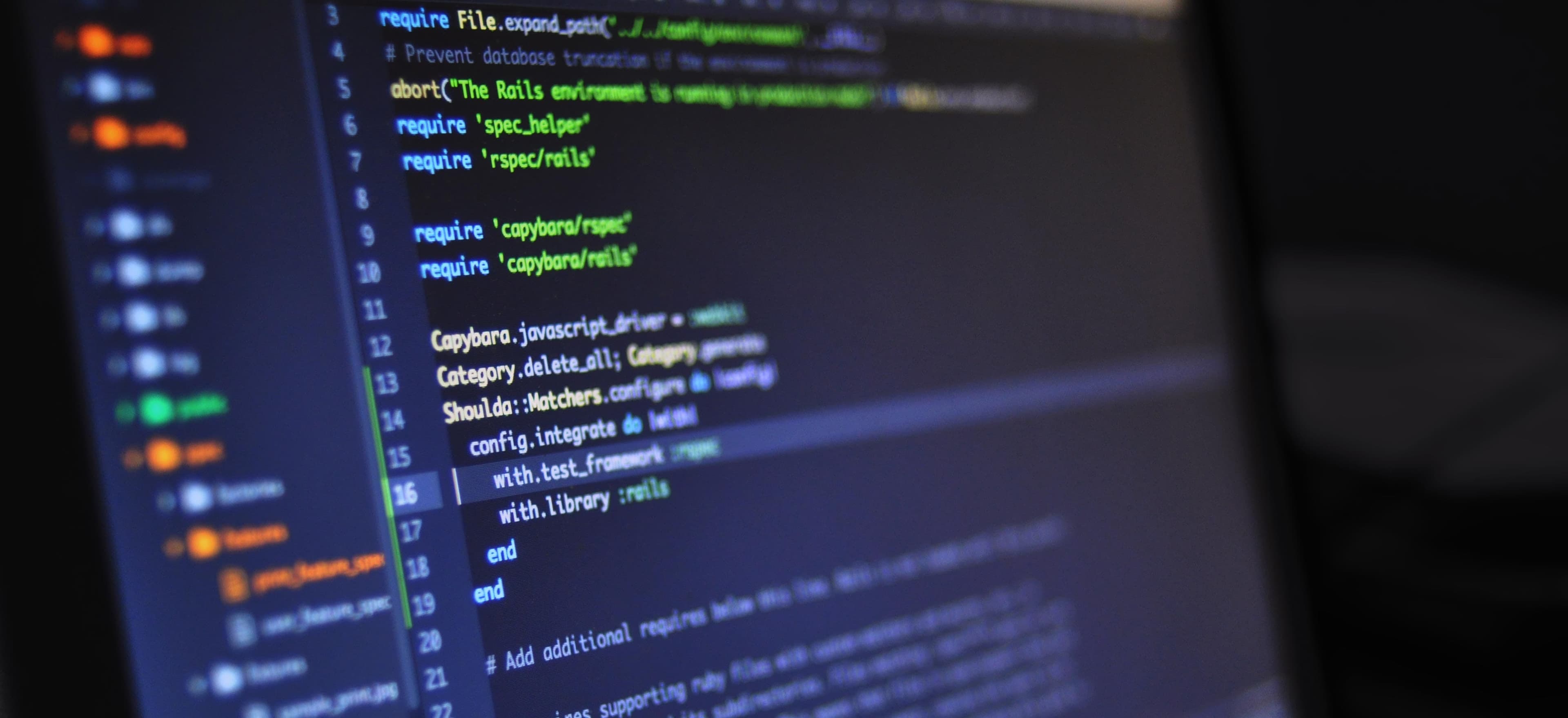
- Published on
Choosing the Best Free Weather API for Your Weather App
Weather APIs are crucial for any weather app, as they provide the necessary data to keep users informed about the current and forecasted weather conditions. When developing a weather app, it's important to select the right weather API to ensure reliable and accurate information for your users.
In this article, we will explore some of the best free weather APIs available, and discuss their features, limitations, and how to utilize them in your Java weather app.
1. OpenWeatherMap API
OpenWeatherMap is one of the most popular and widely used weather APIs, providing current weather data, forecasts, and historical data for any location. It offers a generous free tier that includes access to current weather data and 5-day forecasts with 3-hour granularity.
Usage Example with Java
Here's how you can make a simple API call to retrieve the current weather data using OpenWeatherMap API in Java:
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class OpenWeatherMapExample {
public static void main(String[] args) {
String apiKey = "YOUR_API_KEY";
String city = "London";
String apiUrl = "http://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=" + apiKey;
try {
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we make an HTTP request to OpenWeatherMap's API endpoint to retrieve current weather data for the city of London. Replace YOUR_API_KEY
with your actual API key obtained from OpenWeatherMap.
2. Weatherbit API
Weatherbit is another powerful weather API that provides current weather, forecasts, and historical weather data. The free tier allows for 16-day forecasts, current weather, and hourly forecasts with limited API calls per day.
Usage Example with Java
Let's see how we can fetch the current weather for a specific location using the Weatherbit API in Java:
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class WeatherbitExample {
public static void main(String[] args) {
String apiKey = "YOUR_API_KEY";
String city = "New York";
String apiUrl = "https://api.weatherbit.io/v2.0/current?city=" + city + "&key=" + apiKey;
try {
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we construct the API endpoint URL with the required parameters and make an HTTP request to fetch the current weather data for New York. Don't forget to replace YOUR_API_KEY
with your actual Weatherbit API key.
Final Considerations
When choosing a free weather API for your weather app, it's essential to consider the accuracy and reliability of the data, the features provided in the free tier, and the ease of integration with your Java application. Both OpenWeatherMap and Weatherbit offer robust APIs with free tiers suitable for small-scale weather apps.
It's crucial to explore the documentation and understand the API's capabilities and limitations before integrating it into your weather app. Additionally, consider the frequency of API calls allowed in the free tier, as this can impact the app's performance and user experience.
In conclusion, by carefully evaluating the available free weather APIs and understanding their usage in a Java environment, you can choose the best option for your weather app and provide users with up-to-date and reliable weather information.