Troubleshooting Common Java Programming Errors
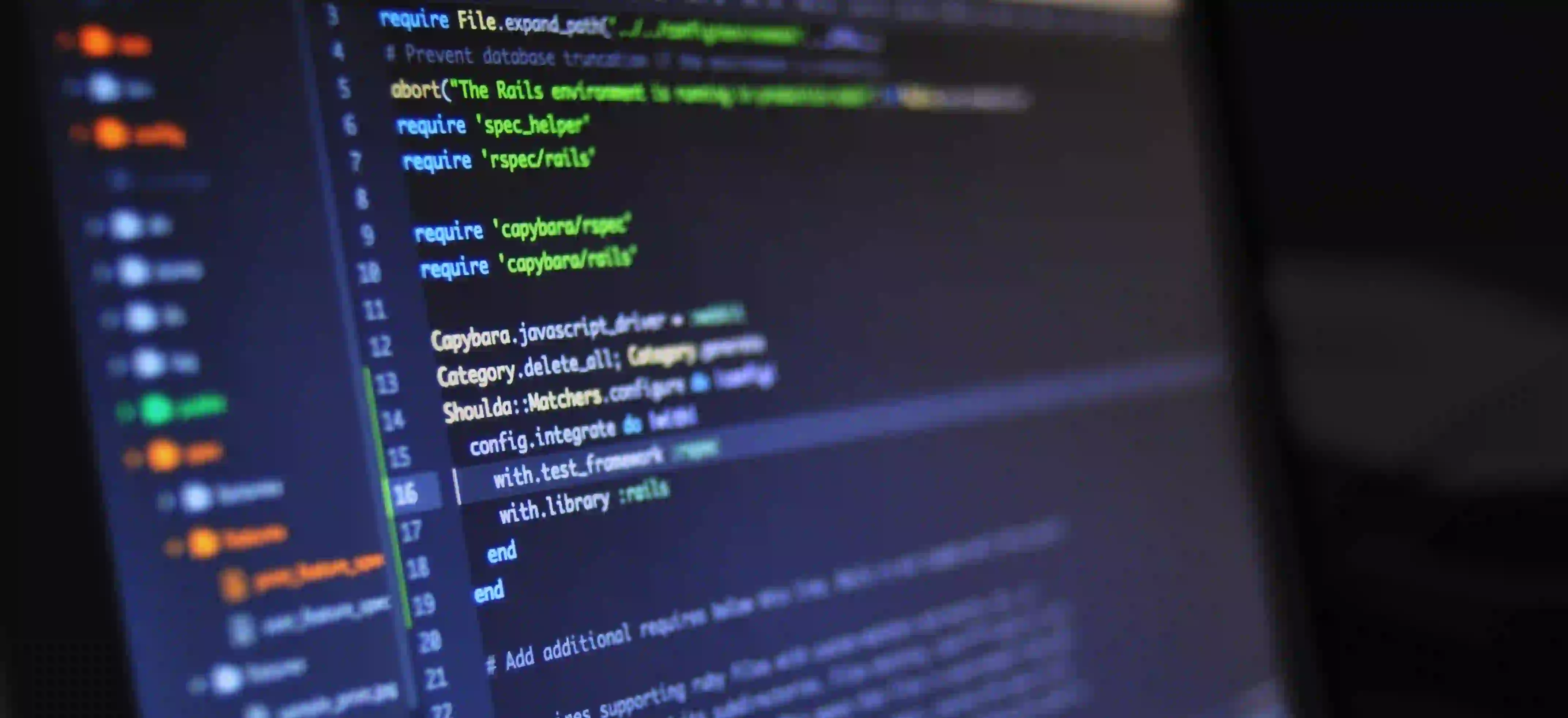
Troubleshooting Common Java Programming Errors
As a Java developer, it's inevitable that you'll encounter errors in your code. While they can be frustrating, understanding how to troubleshoot and resolve these errors is essential for your growth as a programmer. In this post, we'll explore some common Java programming errors and how to approach troubleshooting them.
1. NullPointerException
The dreaded NullPointerException
is one of the most common runtime exceptions in Java. It occurs when you try to access or modify an object reference that is null
. Let's take a look at an example:
public class NullPointerExceptionExample {
public static void main(String[] args) {
String name = null;
System.out.println(name.length());
}
}
In this example, when we try to access the length()
method on the name
variable, a NullPointerException
will be thrown.
To troubleshoot this error, you need to carefully examine your code and identify which object reference is null
. Then, you can add null checks or ensure that the object is properly initialized before using it.
2. ArrayIndexOutOfBoundsException
Another common error in Java is the ArrayIndexOutOfBoundsException
, which occurs when you try to access an index that is outside the bounds of an array. Here's an example:
public class ArrayIndexOutOfBoundsExceptionExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]);
}
}
In this case, trying to access numbers[3]
will result in an ArrayIndexOutOfBoundsException
.
To troubleshoot this error, you should review your array access patterns and ensure that you stay within the bounds of the array. Using loops and conditional statements can help prevent this error from occurring.
3. ClassNotFoundException
The ClassNotFoundException
is thrown when the JVM tries to load a class at runtime using the forName
method in Class
, but the specified class is not found. Here's an example:
public class ClassNotFoundExceptionExample {
public static void main(String[] args) {
try {
Class.forName("com.example.NonExistentClass");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
In this example, the ClassNotFoundException
will be thrown because the NonExistentClass
does not exist.
To troubleshoot this error, double-check the class name and ensure that the class is in the classpath. If the class is from an external library, make sure the library is properly included in your project.
4. Syntax Errors
Syntax errors occur during the compilation of your Java code when the syntax of your program is incorrect. These errors are caught by the compiler, and the code will not be executed until the syntax errors are fixed. Here's an example:
public class SyntaxErrorExample {
public static void main(String[] args) {
System.out.println("Hello, world!")
}
}
In this case, a missing semicolon at the end of the println
statement will result in a syntax error.
To troubleshoot syntax errors, carefully review your code for any typos, missing punctuation, or incorrect keywords. Most modern IDEs provide real-time syntax error highlighting, which can help you identify and fix these errors quickly.
5. Infinite Loop
Accidentally creating an infinite loop is a common logic error in Java. An infinite loop occurs when the condition of the loop always evaluates to true
, causing the loop to run indefinitely. Here's an example:
public class InfiniteLoopExample {
public static void main(String[] args) {
while (true) {
System.out.println("This is an infinite loop!");
}
}
}
In this example, the while (true)
condition will cause the loop to run forever, printing "This is an infinite loop!" repeatedly.
To troubleshoot this error, carefully review your loop conditions and ensure that they will eventually evaluate to false
. Using a loop counter or break statements can help prevent infinite loops.
Key Takeaways
In conclusion, understanding how to troubleshoot common Java programming errors is crucial for every Java developer. By carefully examining your code, understanding the nature of the errors, and employing best practices, you can effectively identify and resolve these errors in your Java programs.
Remember, encountering errors is a natural part of the coding process, and each error presents an opportunity to learn and improve your skills as a Java programmer.
Now that you have a better understanding of common Java programming errors and how to troubleshoot them, continue to expand your knowledge and keep honing your skills. Happy coding!
For more in-depth understanding of Java troubleshooting, refer to the official Java documentation and Java Tutorials.