Optimizing Data Access with Spring Redis Cache
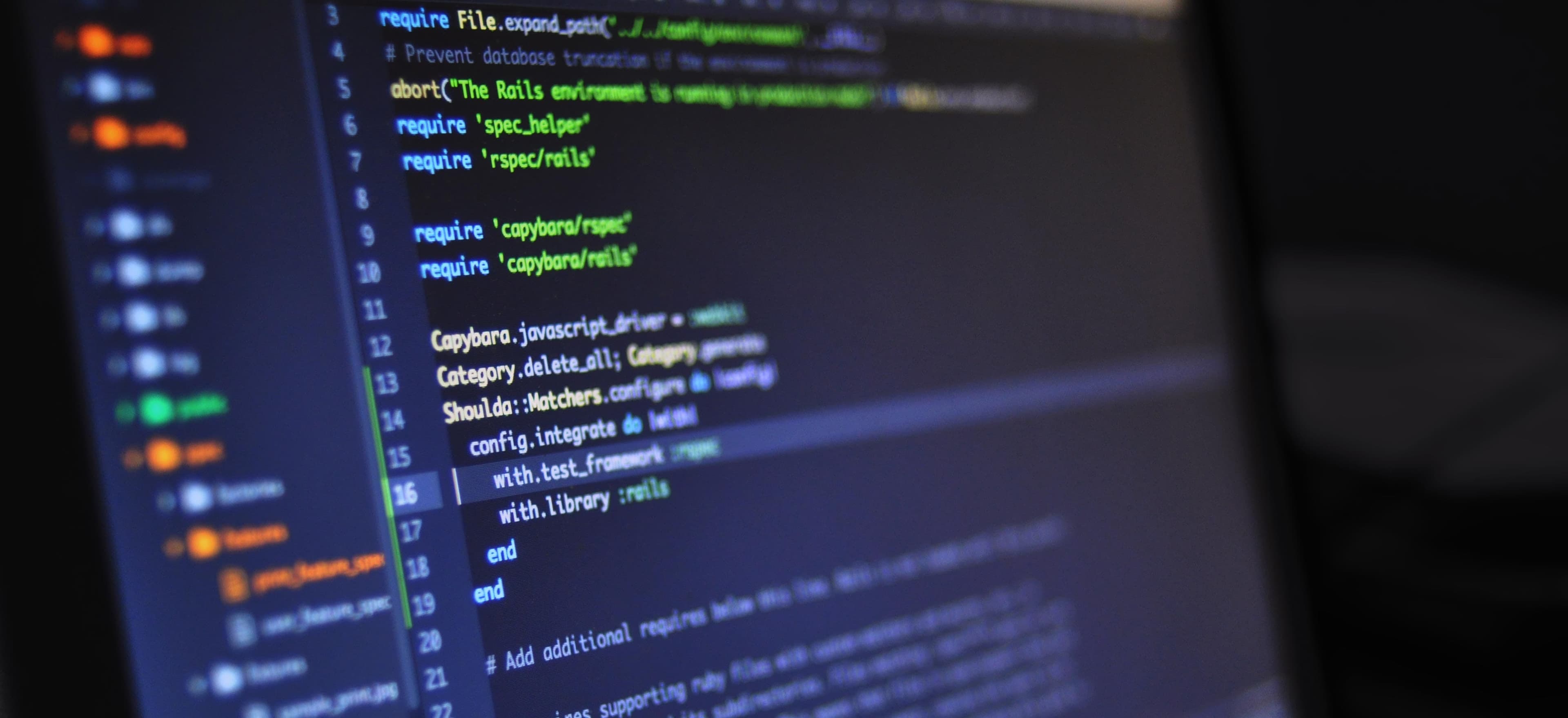
- Published on
Optimizing Data Access with Spring Redis Cache
When it comes to optimizing data access in a Java application, leveraging caching mechanisms can significantly improve performance. One popular caching solution is Redis, a powerful in-memory data store that can be seamlessly integrated with Spring applications. In this post, we will explore how to leverage Spring Redis Cache to optimize data access in a Java application.
What is Redis?
Redis is an open-source, in-memory data structure store known for its exceptional performance and versatility. It can be used as a database, cache, and message broker, making it a popular choice for high-performance applications. Redis supports various data structures such as strings, hashes, lists, sets, and sorted sets, and provides advanced features like transactions, pub/sub messaging, and Lua scripting.
Integrating Redis with Spring
Spring Data Redis provides a powerful abstraction for integrating Redis with Spring applications. It offers a set of convenient templates and abstractions for interacting with Redis, making it easy to incorporate Redis caching into a Spring application.
To get started, you'll need to include the spring-boot-starter-data-redis
dependency in your project. If you're using Maven, you can add the following dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
Configuring Redis Cache in Spring Boot
Once you have the necessary dependencies in place, you can configure Redis caching in your Spring Boot application. Start by annotating your Spring Boot main class with @EnableCaching
to enable Spring's caching support.
@SpringBootApplication
@EnableCaching
public class SpringRedisCacheDemoApplication {
public static void main(String[] args) {
SpringApplication.run(SpringRedisCacheDemoApplication.class, args);
}
}
Next, you'll need to configure a RedisCacheManager
bean to manage the caching behavior. You can do this by creating a @Bean
method in one of your configuration classes. Here's an example of configuring a RedisCacheManager
with a custom RedisTemplate
:
@Configuration
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
return new LettuceConnectionFactory("localhost", 6379);
}
@Bean
public RedisTemplate<String, Object> redisTemplate() {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory());
// Configure serializers, key and value classes, etc.
return template;
}
@Bean
public CacheManager cacheManager() {
RedisCacheConfiguration cacheConfiguration = RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofMinutes(5)); // Set cache expiration
return RedisCacheManager.builder(redisConnectionFactory())
.cacheDefaults(cacheConfiguration)
.build();
}
}
In the above code, we've configured a RedisCacheManager
with a custom RedisTemplate
and set a default expiration time for cached entries.
Using Redis Cache in Spring Service
Now that Redis caching is configured, let's see how we can use it in a Spring service. Consider a simple service that retrieves user data from a database:
@Service
public class UserService {
// Inject UserRepository or use JdbcTemplate to fetch data from the database
@Cacheable(value = "users", key = "#userId")
public User getUserById(Long userId) {
// Fetch user data from the database
return userRepository.findByUserId(userId);
}
@CacheEvict(value = "users", key = "#userId")
public void updateUser(Long userId, User updatedUser) {
// Update user data in the database
}
}
In the UserService
class, we've annotated the getUserById
method with @Cacheable
and the updateUser
method with @CacheEvict
. This tells Spring to cache the results of getUserById
based on the userId
, and to evict the cached entry when updateUser
is called.
By adding these caching annotations, we've effectively enabled caching for the getUserById
method. When the getUserById
method is called with a specific userId
, Spring will first check the cache. If the user data is found in the cache, it will be retrieved from Redis, avoiding the need to hit the database. If the data is not found in the cache, the method will proceed to fetch the data from the database and store it in the cache for future use.
Considerations and Best Practices
While Redis caching can greatly improve performance, it's important to consider certain aspects when using it in a production environment. Here are a few best practices to keep in mind:
Cache Eviction Policies
It's crucial to define appropriate cache eviction policies based on the data access patterns and business requirements. For frequently accessed data that rarely changes, a longer expiration time can be used. On the other hand, volatile data that frequently changes may require a shorter expiration time.
Serialization and Deserialization
When working with complex data structures, ensure that proper serialization and deserialization mechanisms are in place. This is important for maintaining data integrity and consistency when storing and retrieving objects from Redis.
Monitoring and Metrics
Monitor the Redis cache to gain insights into cache hit rates, eviction rates, and memory usage. Tools like RedisInsight and Redis commands such as INFO
can provide valuable metrics for optimizing cache performance.
Error Handling and Fallback Strategies
Implement error handling and fallback strategies to gracefully handle cache failures or Redis downtime. Consider fallback mechanisms to retrieve data from the database if the cache becomes unavailable.
By adhering to these best practices, you can ensure that Redis caching enhances the performance and scalability of your Spring application while maintaining data integrity and resilience.
Final Considerations
In this post, we've explored the integration of Redis caching with Spring applications to optimize data access. By leveraging Spring Data Redis and its caching abstractions, you can effectively improve the performance of your Java application by reducing database load and speeding up data retrieval.
Integrating Redis Cache with Spring involves configuring the RedisCacheManager and utilizing caching annotations in your service layer to cache and evict data based on method calls. Additionally, considering best practices such as cache eviction policies, serialization, monitoring, and error handling is crucial for successful implementation.
With its speed, versatility, and seamless integration with Spring, Redis caching is a valuable asset for optimizing data access and enhancing the overall performance of Java applications.
Give Redis caching a try in your Spring applications, and experience the performance benefits firsthand!