Best Practices for Organizing Single-File Source Code in JDK 11
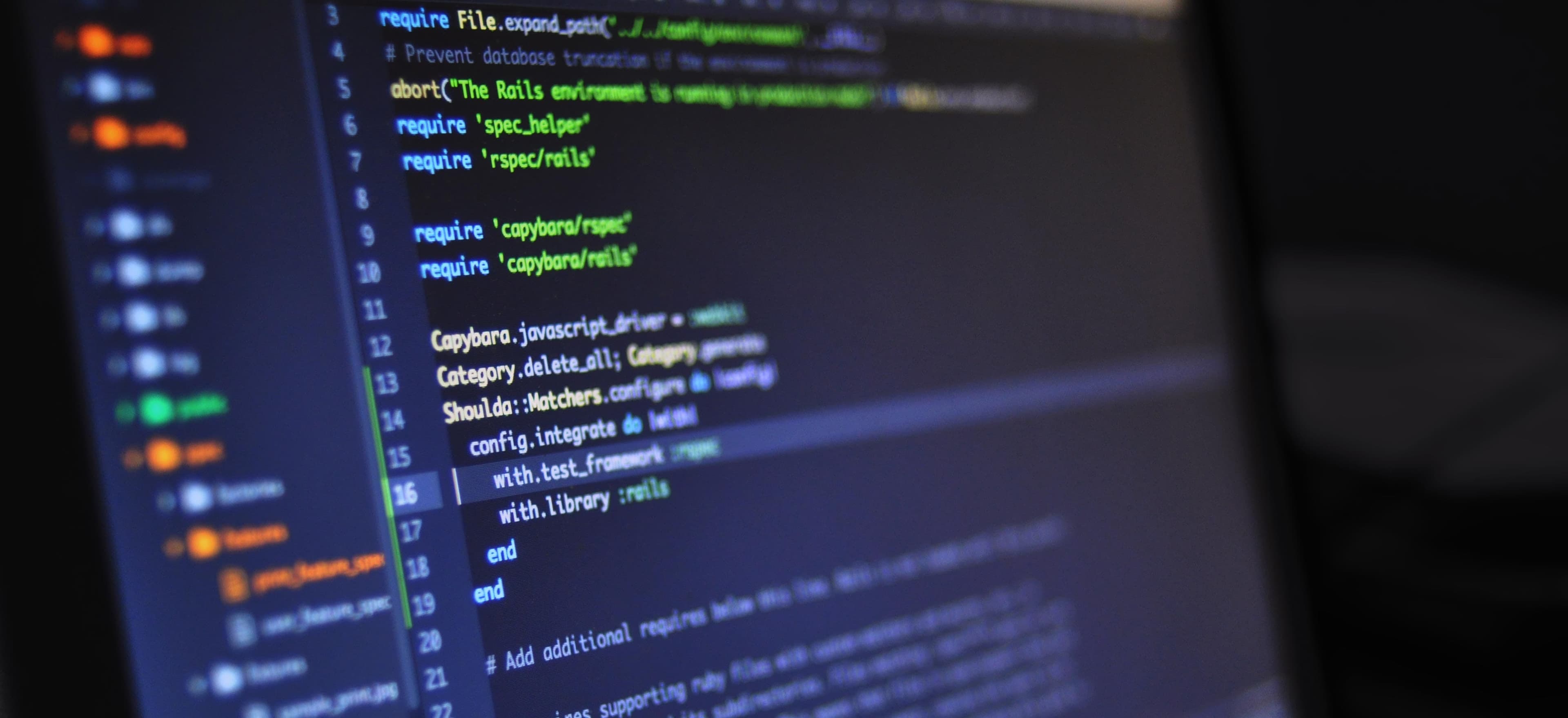
- Published on
Best Practices for Organizing Single-File Source Code in JDK 11
The introduction of JEP 330 in JDK 11 brought the support for running a single-file source-code program directly, without the need for a compilation step. While this can be convenient for quick scripts and small programs, it's important to maintain good organization and structure even in a single-file context. In this article, we'll explore some best practices for organizing single-file source code in JDK 11, covering naming conventions, code structure, and modularization.
Use a Meaningful Filename
When working with single-file source code, it's essential to give the file a meaningful name that reflects its purpose. This helps in quickly understanding the contents of the file without having to open it. For example, if you're writing a simple HTTP server in Java, you can name the file SimpleHttpServer.java
to clearly indicate its functionality.
Package Declaration
Even though the single-file does not require a package declaration, adding one is still good practice, especially if the code is part of a larger project. It helps prevent naming conflicts and ensures proper organization. For instance, if your single-file program relates to utility functions, you can declare it as part of a util
package:
package util;
public class StringUtils {
//...code here...
}
Imports
Organize your import
statements carefully. JDK 11 allows for static imports and wildcard imports, but excessive use of these can clutter the code and make it harder to understand. It's best to import only the classes that are actually used in the file, and avoid using wildcard imports. This not only reduces clutter but also prevents namespace collisions.
Class Structure
In single-file source code, the entire program resides in a single file. Therefore, it's crucial to keep the class structure simple and focused. If the program contains multiple classes or interfaces, ensure that they are logically related. For example, if you're creating a CRUD application, you can include the User
, UserService
, and UserRepository
classes in the same file, as they are closely related in functionality.
Use Comments Sparingly
Comments are essential for explaining complex algorithms or unconventional code, but in single-file source code, it’s important to keep the code as self-explanatory as possible. This means writing clean, expressive code and using comments only when necessary to prevent the clutter of unnecessary comments.
Modularize with Inline Classes
JEP 330 also introduced the concept of inline classes, which allows the definition of a helper class within a single file. This can help modularize the code and keep related classes together. For example, in a single-file program for processing CSV data, you can define an inline class for CsvParser
to encapsulate the parsing logic within the same file.
public class CsvProcessor {
// main code here
// Inline class for CSV parsing
class CsvParser {
// parsing logic here
}
}
Leverage Local Records
With JDK 14, the introduction of local records provided a convenient way to define small, immutable data-carrying classes. In the context of single-file source code, local records can be used to declare lightweight data structures specific to the program. For instance, if your program involves processing employee data, you can use a local record to define the Employee
data structure within the single file.
public class EmployeeProcessor {
record Employee(String name, int age) {
// record body
}
//...rest of the code...
}
Encapsulate Main Logic
In a single-file program, the main
method serves as the entry point. It's a good practice to encapsulate the main logic within a separate method or class to enhance readability and maintainability. This can also make the main
method concise and focused on program startup tasks.
public class DataProcessor {
public static void main(String[] args) {
DataProcessor processor = new DataProcessor();
processor.process();
}
private void process() {
// main logic here
}
}
Utilize Whitespace and Formatting
Just like in multi-file projects, maintain consistent and readable formatting in single-file source code. Use whitespace effectively to separate different sections of the code, and follow a consistent indentation style. This enhances code readability and makes it easier to understand the program flow.
Embrace Functional Programming
With the enhancements in recent Java versions, functional programming features, such as lambda expressions and streams, provide concise and expressive ways to handle data processing. In single-file source code, leveraging functional programming can make the code more elegant and maintainable.
List<String> filteredNames = names.stream()
.filter(name -> name.length() > 5)
.collect(Collectors.toList());
Bringing It All Together
While single-file source code in JDK 11 offers a convenient way to write and execute small Java programs, it's crucial to maintain good organization and structure. By adhering to the best practices discussed in this article – such as meaningful filenames, package declarations, organized imports, modularization, and clear formatting – developers can ensure that their single-file source code remains maintainable and easy to comprehend.
In conclusion, although single-file source code may seem small in scope, it is still important to apply best programming practices to ensure readability and maintainability.
Happy coding!
Start organizing your JDK 11 single-file source code today. And remember, well-organized code leads to well-crafted software!