Optimizing Event Processing with Project Lombok
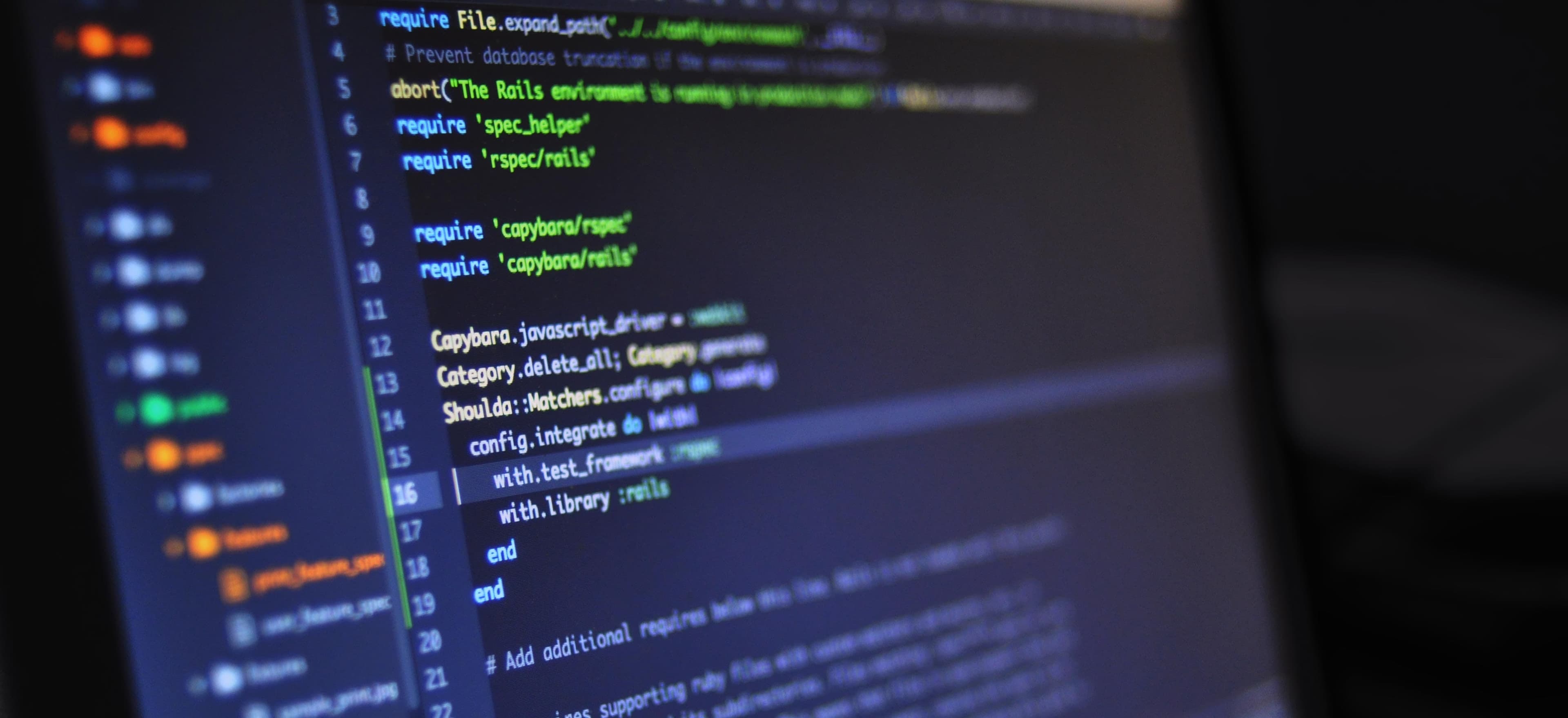
- Published on
Optimizing Event Processing with Project Lombok
Event processing is a crucial aspect of modern software development, especially in the world of distributed systems and microservices. Efficient event processing can significantly impact the performance and scalability of an application. In Java, Project Lombok is a powerful tool that can be used to optimize event processing by reducing boilerplate code and improving code readability. In this post, we will explore how Project Lombok can be leveraged to streamline event processing code and improve overall application performance.
Understanding Event Processing
Before diving into the optimization techniques, it's important to understand the basics of event processing. In a typical application, events represent occurrences or interactions within the system. These events can range from user actions, such as clicking a button, to system-generated events, like data updates or notifications.
In a distributed system, events are often used for communication between services, enabling asynchronous and decoupled interactions. Event processing involves capturing, handling, and responding to these events, often in real-time or near real-time.
Challenges in Event Processing
Efficient event processing comes with its own set of challenges. The codebase can quickly become cluttered with repetitive and verbose code, leading to decreased maintainability and readability. Additionally, handling immutable data and enforcing thread safety adds complexity to event processing code.
Project Lombok offers a solution to these challenges by reducing boilerplate code and providing annotations that automate the generation of repetitive code, thereby improving the overall code quality and maintainability.
Leveraging Project Lombok for Event Processing
Project Lombok provides a set of annotations and utilities to simplify the creation of Java classes by generating boilerplate code during compilation. Let's explore how Project Lombok can be used to optimize event processing.
Immutable Data with @Value
In event processing, immutable data objects are preferred to ensure data consistency and thread safety. Manually creating immutable classes involves writing constructors, getters, and other related methods, which can be time-consuming and error-prone.
Project Lombok's @Value
annotation generates immutable classes, including the required constructors, accessors, and equals
/ hashCode
methods. Here's an example of using @Value
for an event class:
import lombok.Value;
@Value
public class Event {
String id;
String type;
long timestamp;
// Other fields and methods
}
The @Value
annotation eliminates the need for writing boilerplate code for immutable classes, allowing developers to focus on the actual event handling logic.
Simplifying Builders with @Builder
In event processing, it's common to use builder patterns to create complex event objects with optional and mandatory attributes. Writing a traditional builder class involves creating a constructor with numerous parameters and setter methods for each attribute, which can clutter the code and reduce readability.
Project Lombok's @Builder
annotation simplifies the creation of builder classes by automatically generating the builder pattern code. Here's an example of using @Builder
for an event builder:
import lombok.Builder;
import lombok.Singular;
@Builder
public class Event {
String id;
String type;
long timestamp;
@Singular
List<String> attributes;
// Other fields and methods
}
The @Builder
annotation streamlines the builder pattern implementation, making it easier to create and initialize complex event objects.
Simplifying Logging with @Slf4j
Logging plays a crucial role in event processing for tracking the flow of events, debugging, and monitoring application behavior. Manually creating logger instances in each class can lead to code duplication and decreased maintainability.
Project Lombok's @Slf4j
annotation simplifies logging by generating a logger field in the class. Here's an example of using @Slf4j
for event processing:
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class EventProcessor {
public void processEvent(Event event) {
log.info("Processing event: {}", event.getType());
// Event processing logic
}
}
The @Slf4j
annotation eliminates the need for explicitly defining the logger instance and provides a standardized approach to logging across classes.
Encapsulating Data with @Getter and @Setter
In event processing, encapsulating the event data through getter and setter methods is essential to control access and modification of the event attributes. Manually writing these methods for each attribute can result in code redundancy and decreased maintainability.
Project Lombok's @Getter
and @Setter
annotations automate the generation of getter and setter methods for class attributes. Here's an example of using @Getter
and @Setter
for event attributes:
import lombok.Getter;
import lombok.Setter;
public class Event {
@Getter @Setter
private String id;
@Getter @Setter
private String type;
@Getter @Setter
private long timestamp;
// Other fields and methods
}
The @Getter
and @Setter
annotations reduce the boilerplate code for getter/setter methods, allowing for cleaner and more readable code.
Final Considerations
Project Lombok provides a robust set of features and annotations that can significantly optimize event processing in Java applications. By leveraging annotations such as @Value
, @Builder
, @Slf4j
, @Getter
, and @Setter
, developers can streamline event processing code, reduce boilerplate code, and improve overall code readability and maintainability.
Optimizing event processing with Project Lombok not only enhances the developer experience but also contributes to the performance and scalability of the application. As event-driven architectures continue to gain popularity, tools like Project Lombok play a vital role in simplifying and enhancing event processing in Java applications.
Incorporating Project Lombok into event processing code can lead to more efficient and maintainable applications, ultimately benefiting both developers and end-users alike. So why wait? Start optimizing your event processing code with Project Lombok today!
Remember, optimizing event processing code with Project Lombok is just one step towards building robust and scalable event-driven applications in Java!
If you are interested in learning more about event-driven architecture, consider checking out this detailed guide by Martin Fowler on Event-Driven Architecture for an in-depth understanding of the concepts and best practices.
Until next time, happy coding!