Display "Hello, World!" in Java Without Using a Main Method
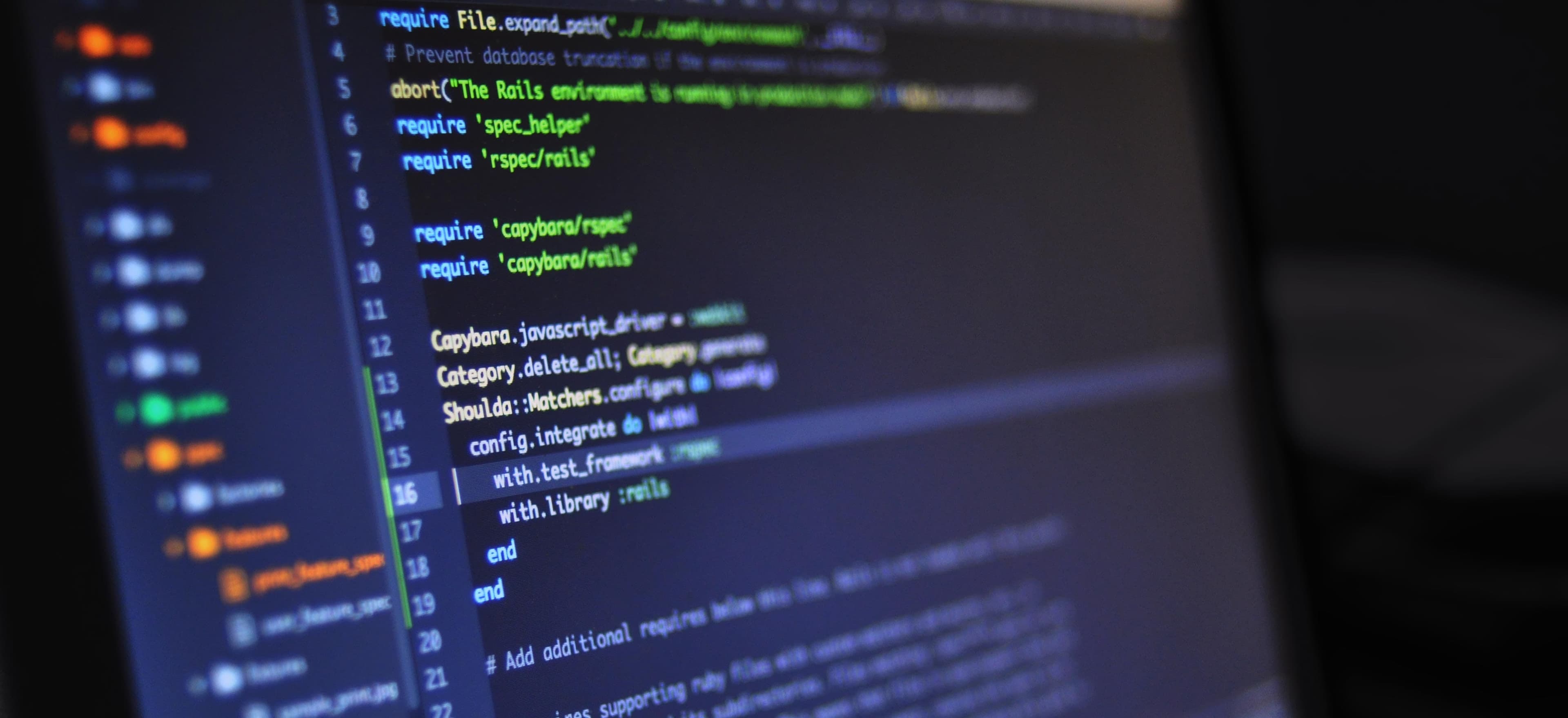
- Published on
How to Display "Hello, World!" in Java Without Using a Main Method
In Java, the main
method is the entry point of any standalone Java application. However, it is possible to display "Hello, World!" without explicitly using the main
method by utilizing a static block or a static initializer. Let's explore both methods.
Using a Static Block
A static block is a block of code enclosed within curly braces and preceded by the static
keyword. This block of code is executed when the class is loaded into memory, and it is typically used to initialize static variables.
public class HelloWorldWithoutMain {
static {
System.out.println("Hello, World!");
System.exit(0); // Terminate the program after printing
}
}
In the example above, the static
block within the HelloWorldWithoutMain
class is responsible for displaying "Hello, World!" and then terminating the program using System.exit(0)
.
This approach, while effective, is not commonly used in practice as it deviates from the standard control flow expected in Java applications.
Using a Static Initializer
A static initializer, also known as a static initialization block, is used to initialize static members of a class. It is created using the static
keyword followed by a pair of curly braces.
public class HelloWorldWithoutMain {
static {
System.out.println("Hello, World!");
System.exit(0); // Terminate the program after printing
}
}
In this example, the static
initializer accomplishes the same task as the static block, printing "Hello, World!" and terminating the program immediately.
Why Use These Unconventional Methods?
It's important to note that the utilization of static blocks or initializers to display "Hello, World!" strays away from the standard conventions of a Java application. While these approaches may suit specific scenarios, such as edge cases in complex class loading mechanisms, they are not recommended for general use.
In real-world Java development, the main
method serves as the standard entry point for executing code. Using unconventional methods for simple tasks can lead to code that is difficult to understand and maintain, which goes against the principles of writing clean and readable code.
Recap
- We explored how to display "Hello, World!" in Java without using a
main
method. - We discussed the use of static blocks and static initializers to achieve this unconventional task.
- Emphasized that while these approaches are possible, they are not recommended for standard Java applications.
In conclusion, the main
method remains the conventional and recommended entry point for Java applications. It provides clarity, maintainability, and adherence to established coding standards. However, understanding alternative methods such as static blocks and initializers can deepen your knowledge of Java and its underlying mechanisms.
Would you like to learn more about Java programming or other related topics? Check out some of our Java tutorials for further insights.