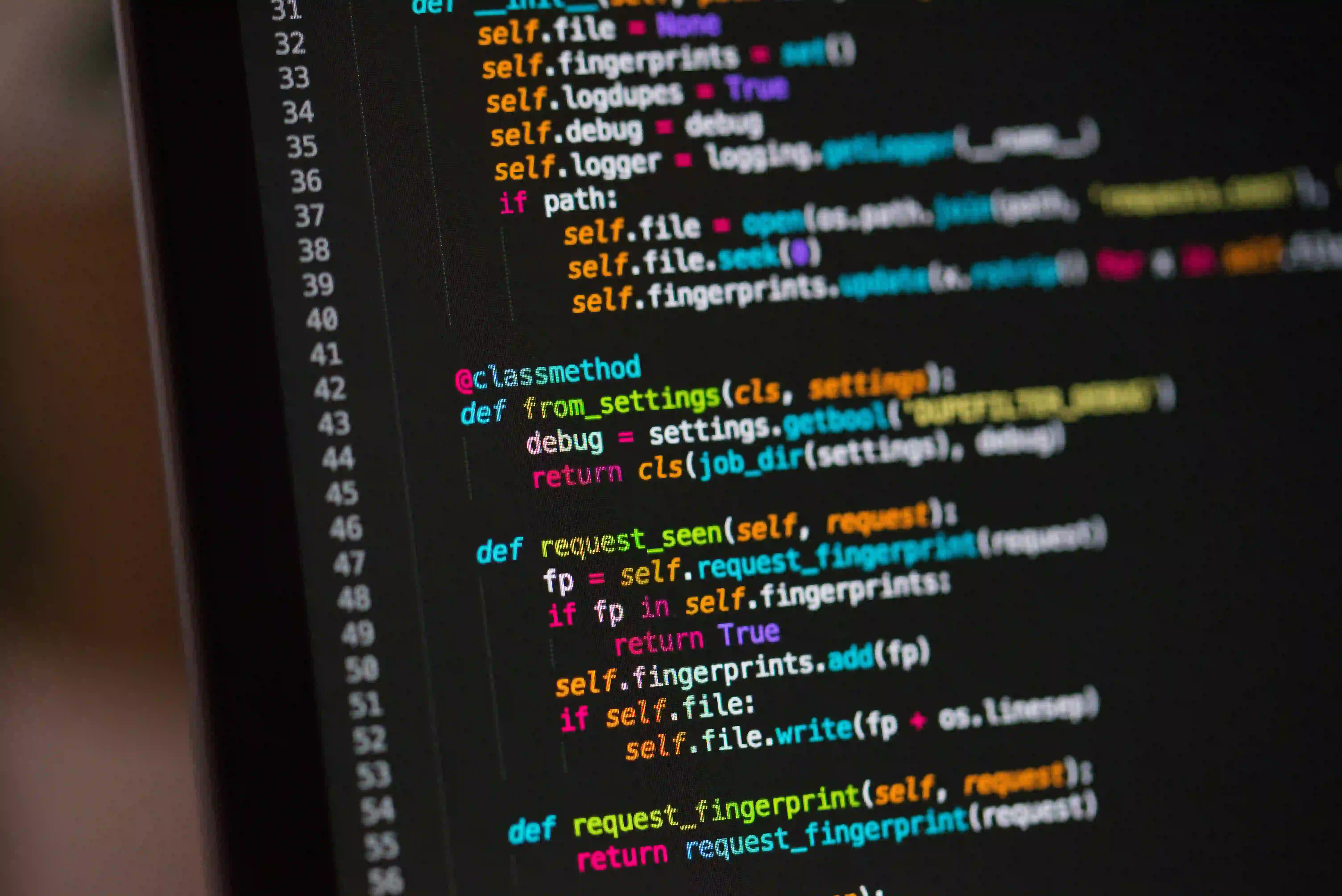
How to Secure Web Applications with JAAS
Securing web applications is a critical aspect of modern software development. The Java Authentication and Authorization Service (JAAS) provides a flexible and powerful framework for securing Java applications, including web applications. In this blog post, we will explore how to use JAAS to secure web applications, including implementing authentication and authorization.
What is JAAS?
JAAS, or Java Authentication and Authorization Service, is a Java security framework that allows applications to implement user-based security. JAAS provides a way for applications to authenticate and authorize users, and it can be used in a variety of Java applications, including web applications.
Setting Up JAAS
To begin using JAAS to secure your web application, you'll need to set up the JAAS configuration. This involves creating a jaas.conf
file that defines the login modules and their configurations. Here's an example of a jaas.conf
file:
WebApp {
com.example.security.CustomLoginModule required;
};
In this example, we define a WebApp
configuration with a custom login module called CustomLoginModule
. This login module will be responsible for authenticating users in our web application.
Implementing Authentication with JAAS
Once the JAAS configuration is set up, we can implement authentication in our web application. We can use the LoginContext
class to authenticate users using the configured login modules. Here's an example of how we can authenticate a user using JAAS:
import javax.security.auth.login.LoginContext;
import javax.security.auth.login.LoginException;
public class WebAppAuthenticator {
public boolean authenticateUser(String username, String password) {
try {
LoginContext lc = new LoginContext("WebApp", new CustomCallbackHandler(username, password));
lc.login();
return true;
} catch (LoginException e) {
return false;
}
}
}
In this example, we create a LoginContext
with the WebApp
configuration and attempt to log in the user using the CustomCallbackHandler
to provide the user's credentials.
Implementing Authorization with JAAS
Once authentication is in place, we can use JAAS to implement authorization in our web application. Authorization determines what actions authenticated users are allowed to perform. We can use the Subject
class to represent the authenticated user and make authorization decisions based on the user's roles and permissions. Here's an example of how we can implement authorization with JAAS:
import javax.security.auth.Subject;
public class WebAppAuthorizer {
public boolean isUserAuthorized(Subject subject, String requiredRole) {
return subject.getPrincipals().stream()
.anyMatch(principal -> principal.getName().equals(requiredRole));
}
}
In this example, we create a WebAppAuthorizer
class that checks if a user has a required role. We do this by inspecting the user's Subject
and checking if it contains a Principal
with the required role.
Integrating JAAS with a Web Framework
To integrate JAAS with a web framework, such as Java EE or Spring, we need to configure the framework to use JAAS for authentication and authorization. Each web framework provides a way to configure security settings, including the JAAS configuration. For example, in Java EE, we can configure the web.xml
deployment descriptor to specify the security realm and the login configuration. Here's an example of how we can configure JAAS in a Java EE web application:
<login-config>
<auth-method>FORM</auth-method>
<realm-name>WebAppRealm</realm-name>
<form-login-config>
<form-login-page>/login.jsp</form-login-page>
<form-error-page>/error.jsp</form-error-page>
</form-login-config>
</login-config>
<security-constraint>
<web-resource-collection>
<web-resource-name>Secured Resources</web-resource-name>
<url-pattern>/secured/*</url-pattern>
</web-resource-collection>
<auth-constraint>
<role-name>user</role-name>
</auth-constraint>
</security-constraint>
<security-role>
<role-name>user</role-name>
</security-role>
In this example, we configure the web.xml
file to use a FORM-based authentication method and specify the WebAppRealm
as the security realm. We also define a security constraint that requires users to have the user
role to access resources under the /secured/*
URL pattern.
To Wrap Things Up
In conclusion, JAAS provides a powerful framework for securing web applications in Java. By configuring JAAS, implementing authentication and authorization, and integrating it with a web framework, we can effectively secure our web applications and protect our users' data and resources. Understanding how to use JAAS is crucial for any Java developer building secure web applications.
By utilizing JAAS for secure web applications, developers can ensure that user data is protected, and access to sensitive resources is appropriately controlled. The flexibility and extensibility of JAAS make it a valuable tool for implementing robust security measures in Java web applications.