Unlock the Power of ConfigurationProperties in Spring
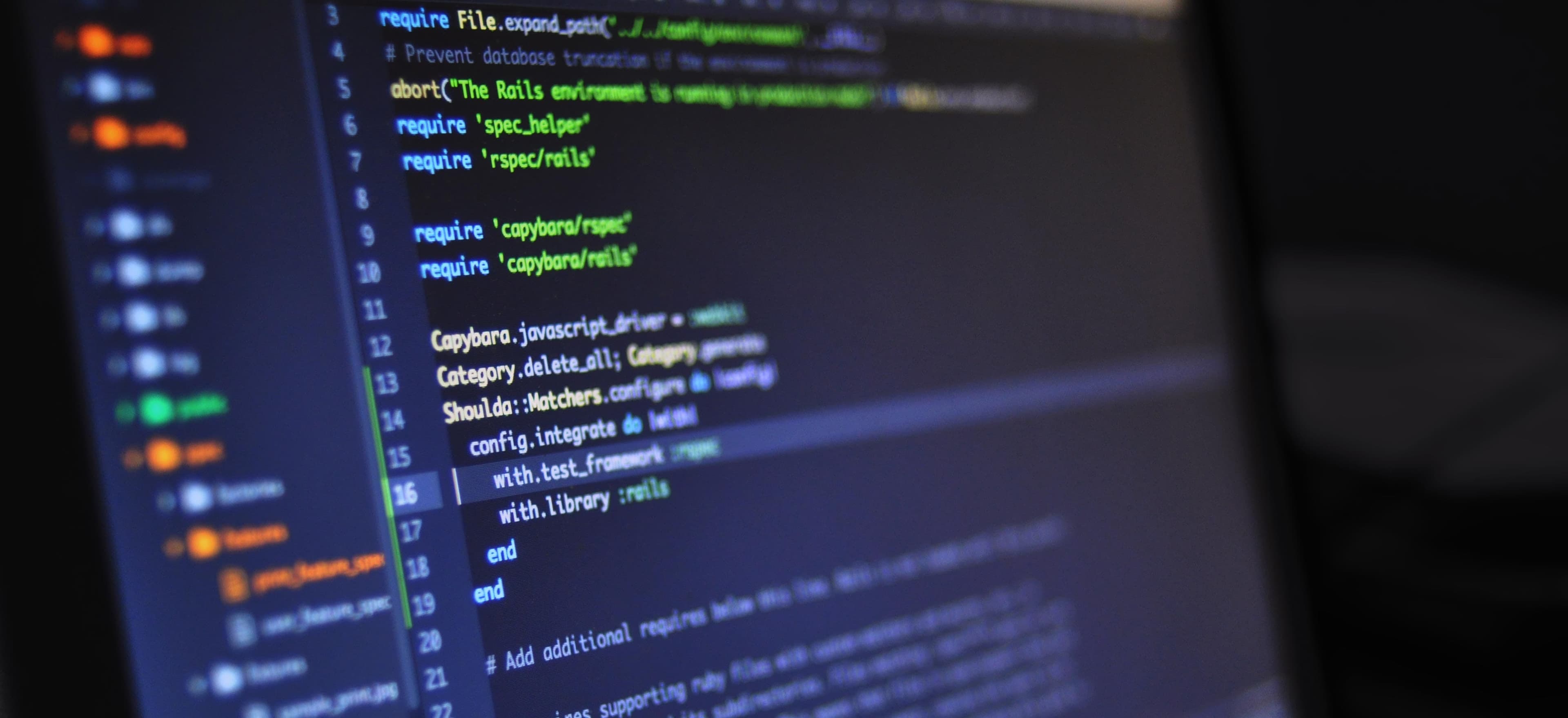
- Published on
Unlock the Power of @ConfigurationProperties
in Spring
When working with Spring applications, you often encounter the need to handle configuration properties. Whether it's database connection details, external service endpoints, or feature toggles, managing these properties efficiently is crucial. In this article, we'll explore how to leverage the @ConfigurationProperties
annotation in Spring to simplify the handling of configuration properties.
What are @ConfigurationProperties
?
In Spring, the @ConfigurationProperties
annotation is used to bind external configuration properties to a Java object. This powerful feature eliminates the need to manually retrieve and parse individual properties from the environment. By encapsulating related properties within a dedicated class, you can neatly organize and access them throughout your application.
Setting up @ConfigurationProperties
To start using @ConfigurationProperties
, let’s create a simple Spring Boot application. Assuming you have a Spring Boot project set up, you can follow these steps to set up @ConfigurationProperties
.
- Create a Configuration Class: Create a new Java class and annotate it with
@Configuration
and@ConfigurationProperties
. Let's name itAppConfig
.
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Configuration;
@Configuration
@ConfigurationProperties(prefix = "app")
public class AppConfig {
private String name;
private String version;
// Getters and setters
}
In this example, we've defined an AppConfig
class that binds properties with the prefix "app" to corresponding fields.
- Enable Configuration Properties: Ensure that the
@ComponentScan
annotation is present in your Spring Boot application main class or any configuration class.
@SpringBootApplication
@ComponentScan(basePackages = "com.example")
public class Application {
// Application setup
}
By including @ComponentScan
, Spring Boot will scan for components, including our AppConfig
.
Binding Properties
After setting up the configuration class, we can now bind properties from various sources, such as application.properties
, application.yml
, environment variables, and command-line arguments. Let’s consider the following application.properties
file:
app.name=MyApp
app.version=1.0
Now, when the Spring application starts, the properties app.name
and app.version
will be automatically mapped to the fields in the AppConfig
class.
Leveraging @Value
vs @ConfigurationProperties
While @Value
can be used to inject individual properties, @ConfigurationProperties
is more suitable for binding an entire group of related properties to a single object. This provides a clearer structure and makes it easier to manage and access configuration properties.
@Value("${app.name}")
private String appName;
Using @Value
for each property can clutter the codebase and make it harder to maintain, especially as the number of properties grows.
Validating Properties
One of the key advantages of @ConfigurationProperties
is the built-in support for validating properties using standard JSR-303 javax.validation
annotations.
import javax.validation.constraints.NotBlank;
@Configuration
@ConfigurationProperties(prefix = "app")
public class AppConfig {
@NotBlank
private String name;
private String version;
// Getters and setters
}
In this example, the @NotBlank
annotation ensures that the name
property is not empty or null. Spring Boot will automatically validate the properties during application startup and report any validation errors.
Reloading Properties
In certain scenarios, you may need to reload configuration properties without restarting the entire application. Spring Boot provides a convenient way to achieve this by using @RefreshScope
in combination with @ConfigurationProperties
.
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.stereotype.Component;
@Component
@RefreshScope
@ConfigurationProperties(prefix = "app")
public class AppConfig {
// Configuration properties
}
By annotating the AppConfig
class with @RefreshScope
, Spring Boot will refresh the annotated beans when a @RefreshScope
refresh event occurs, allowing the properties to be reloaded dynamically.
Usage in Spring MVC
Using @ConfigurationProperties
in Spring MVC REST controllers can significantly simplify the handling of incoming request payloads. By binding the request payload to a POJO using @ConfigurationProperties
, you can automatically map and validate the incoming data.
@RestController
@RequestMapping("/api/users")
public class UserController {
@PostMapping
public ResponseEntity createUser(@Valid @RequestBody UserRequest userRequest) {
// Process the user request
}
}
@Component
@ConfigurationProperties(prefix = "app.user")
public class UserRequest {
@NotBlank
private String username;
@Email
private String email;
// Getters and setters
}
Here, the UserRequest
class represents the incoming request payload, and by annotating it with @ConfigurationProperties
, we can conveniently handle and validate the request data in the REST controller.
Lessons Learned
In conclusion, utilizing the @ConfigurationProperties
annotation in Spring provides a clean and efficient way to manage configuration properties, promote code reusability, and ensure type safety. By encapsulating related properties into dedicated classes and leveraging validation and reloading capabilities, you can streamline your application’s configuration management. Embrace the power of @ConfigurationProperties
to take your Spring applications to the next level of robustness and maintainability.
Take your Spring application to the next level by mastering @ConfigurationProperties
, and delve deeper into advanced topics with comprehensive courses from Spring University and expert-led tutorials on Spring Configuration.