Building Scalable Microservices with Quarkus and Qute Templates
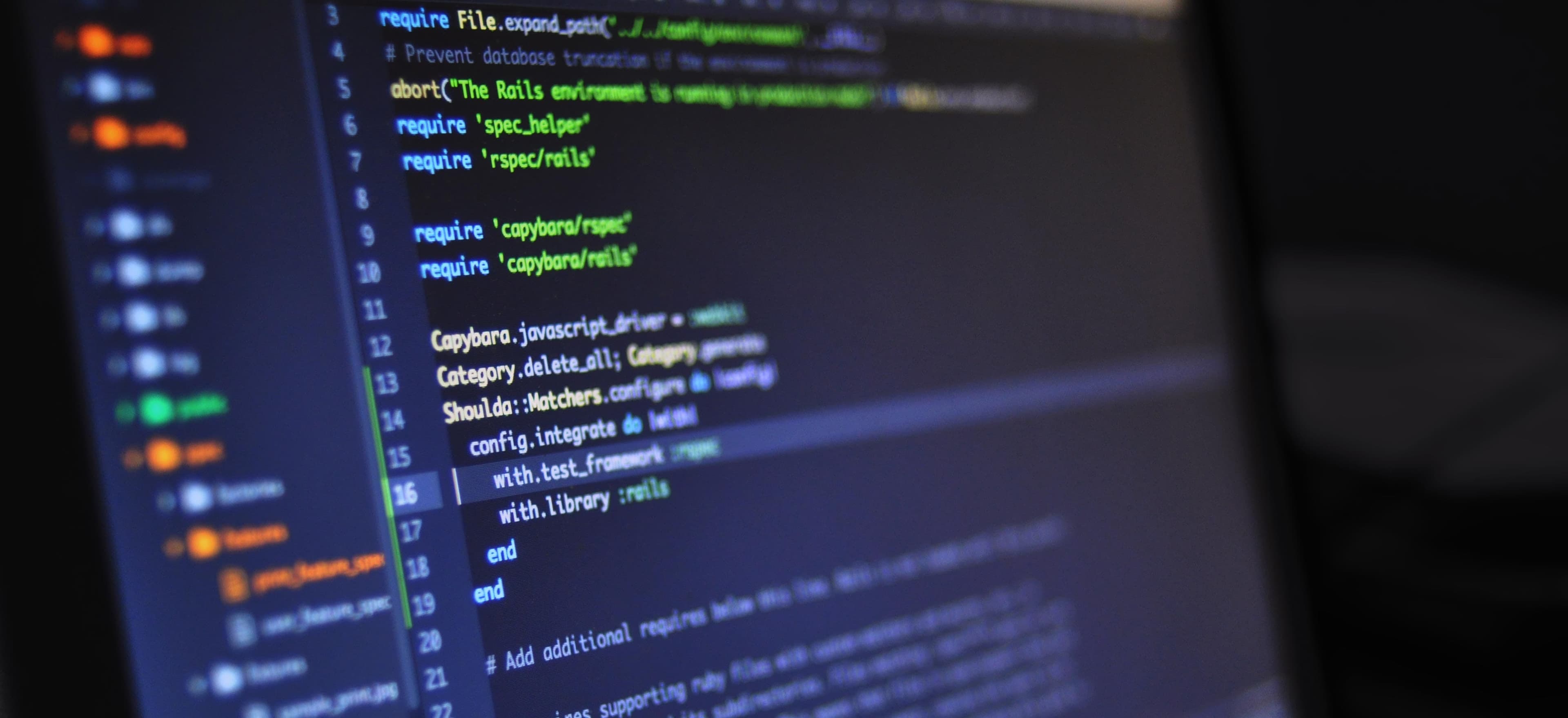
- Published on
Building Scalable Microservices with Quarkus and Qute Templates
In the world of microservices, scalability and performance are key considerations. The ability to efficiently handle an increasing number of requests and effectively manage resources is crucial for any microservice architecture. In this blog post, we will explore how to build scalable microservices using Quarkus, a supersonic subatomic Java framework, and Qute templates, a high-performance templating engine.
Introducing Quarkus
Quarkus is a Kubernetes Native Java stack tailored for GraalVM and HotSpot, crafted from best-of-breed Java libraries and standards. It is designed to significantly reduce the memory footprint and boot time of Java applications and offers seamless integration with popular Java standards and frameworks.
Why Quarkus?
- Quarkus boasts remarkable startup time and low memory usage, making it an ideal choice for microservices where rapid scaling and resource efficiency are paramount.
- Its support for compile-time boot, live coding, and Just-in-Time (JIT) compilation enables seamless development and testing of microservices.
Leveraging Qute Templates for Scalability
Qute is a high-performance templating engine designed for Quarkus. It brings the concept of reactive templating to Java, allowing developers to build scalable and efficient user interfaces.
Why Qute Templates?
- Qute templates offer a responsive, asynchronous, and non-blocking approach to building user interfaces, which aligns perfectly with the requirements of scalable microservices.
- With its lightweight and high-performance nature, Qute templates ensure minimal impact on the overall performance and scalability of microservices.
Getting Started with Quarkus and Qute Templates
To demonstrate the power of Quarkus and Qute templates in building scalable microservices, let's dive into a practical example of building a simple yet scalable web application.
Setting up the Project
First, we need to set up a new Quarkus project using the Maven quarkus-maven-plugin
. We can achieve this by executing the following command:
mvn io.quarkus:quarkus-maven-plugin:2.7.6.Final:create \
-DprojectGroupId=com.example \
-DprojectArtifactId=quarkus-qute-demo \
-DclassName="com.example.GreetingResource" \
-Dpath="/hello"
This command creates a new Quarkus project with the necessary directory structure and basic configuration.
Integrating Qute Templates
Next, we'll integrate Qute templates into our project to handle the UI rendering. We can achieve this by adding the Qute dependency to the pom.xml
file:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-qute</artifactId>
</dependency>
Creating a Qute Template
We'll create a Qute template for rendering a simple HTML page. Within the src/main/resources/templates
directory, create a file named index.html
with the following content:
<!DOCTYPE html>
<html>
<head>
<title>Quarkus Qute Demo</title>
</head>
<body>
<h1>Hello, {name}!</h1>
</body>
</html>
Implementing the Resource
Now, let's implement a REST endpoint using Quarkus to serve the Qute template with dynamic data. Below is an example of how the GreetingResource
class can be implemented:
import io.quarkus.qute.TemplateInstance;
import io.quarkus.qute.Template;
import io.quarkus.qute.TemplateLocator;
import javax.inject.Inject;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
import java.util.HashMap;
import java.util.Map;
@Path("/hello")
public class GreetingResource {
@Inject
Template index; // Inject the Qute template
@GET
@Produces(MediaType.TEXT_HTML)
public TemplateInstance get() {
Map<String, String> data = new HashMap<>();
data.put("name", "Quarkus");
return index.data("name", data.get("name")); // Pass dynamic data to the template
}
}
In the above code, we inject the Qute template index
and define a REST endpoint that returns the rendered template with the dynamic data.
Running the Application
With the project set up and the necessary code written, we can now run our Quarkus application using the following Maven command:
./mvnw compile quarkus:dev
This command starts the Quarkus development mode, enabling live coding and real-time application updates as we make changes to the code.
Closing Remarks
In this post, we explored the potential of building scalable microservices using Quarkus and Qute templates. With Quarkus' unmatched performance and low footprint, coupled with Qute templates' reactive and high-performance nature, we can craft microservices that are not only efficient but also easily scalable. By leveraging these technologies, developers can ensure that their microservices have the agility and responsiveness needed to handle increasing workloads, making them well-prepared for the demands of the modern digital landscape.
Now that we have an understanding of how Quarkus and Qute templates can be utilized to build scalable microservices, it's time to delve deeper into the limitless possibilities and optimizations these technologies offer. Whether it's integrating with other microservices, leveraging advanced Quarkus extensions, or fine-tuning Qute templates for performance, there's always more to explore in the realm of scalable microservices.
So, embrace the power of Quarkus and the reactivity of Qute templates, and embark on a journey to create highly scalable microservices that are primed for the challenges of tomorrow's digital ecosystem. Happy coding!
For further reading on Quarkus and Qute templates, refer to the official documentation: