Max-age directive not working for HTTP caching
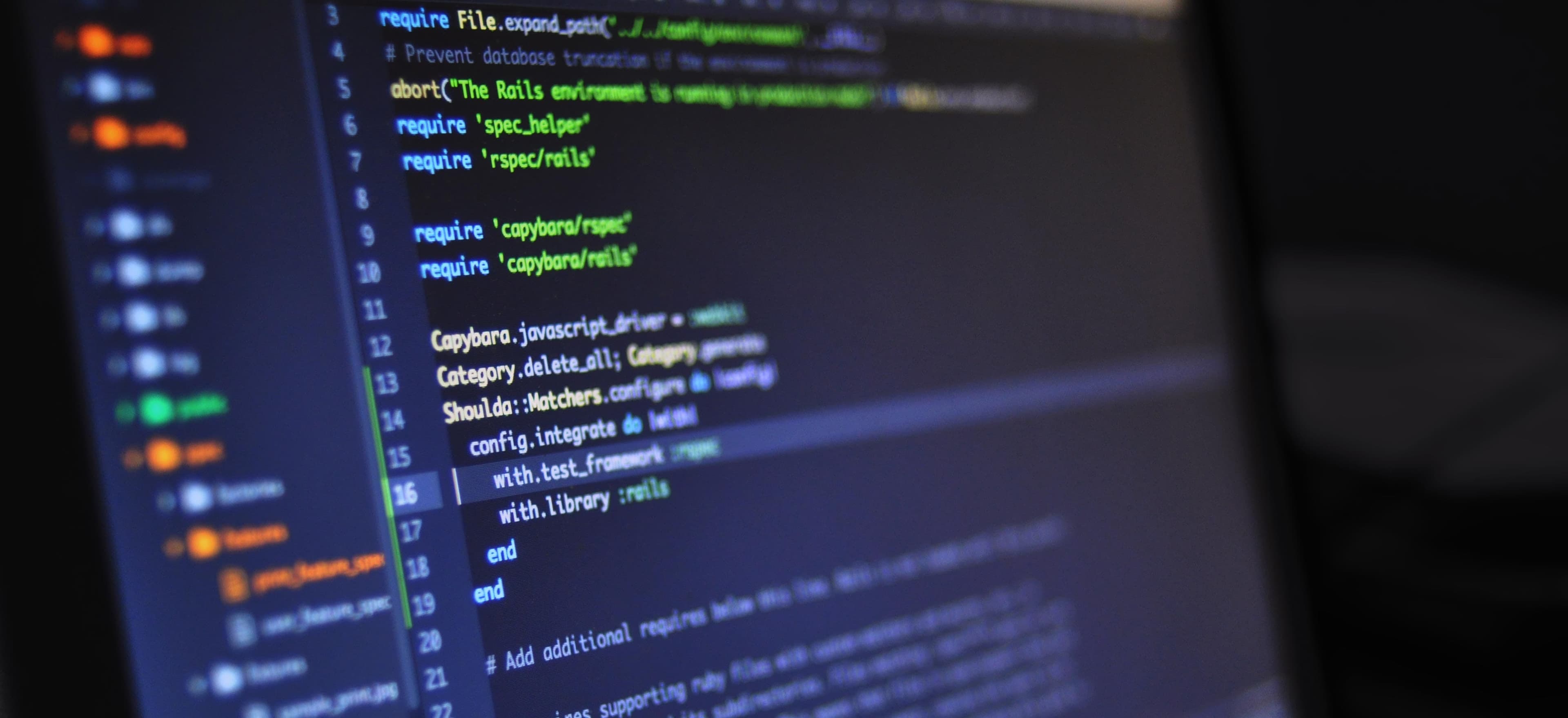
- Published on
Understanding the Issue with max-age Directive in HTTP Caching
When it comes to optimizing website performance and load times, caching is a crucial aspect. It allows for the efficient reusing of previously fetched resources, ultimately reducing the need for redundant requests to the server. However, it's not uncommon to encounter issues with caching directives. One such problem that developers often face is the misconfiguration or misinterpretation of the max-age
directive in HTTP caching. In this article, we'll delve into the nuances of the max-age
directive, explore common pitfalls, and provide solutions to ensure effective caching in your Java web applications.
The Basics of HTTP Caching
HTTP caching is a mechanism that allows the browser to store web resources locally. When a user revisits a page, the browser can check if the resources are still valid and use the locally stored copies instead of requesting them from the server again. This reduces latency and improves overall performance.
Caching directives are sent as part of the HTTP headers, instructing the browser on how to cache and reuse resources. One of the most widely used directives is Cache-Control
, which contains various directives including max-age
.
Understanding the max-age Directive
The max-age
directive specifies the maximum amount of time, in seconds, that a resource is considered fresh. When a cached resource's age exceeds the max-age
value, it is deemed stale, and a new request for the resource is made to the server. In essence, max-age
dictates for how long the cached resource can be used without revalidating it from the server.
Potential Issues with max-age
Incorrect Syntax or Placement
One common issue with the max-age
directive is incorrect syntax or placement within the HTTP headers. It's crucial to ensure that the Cache-Control
header is correctly formatted, and the max-age
value is specified appropriately.
Server-Side Configuration
Another potential issue lies in the server-side configuration. If the server does not send the max-age
directive or sends conflicting caching directives, the client's caching behavior may not align with the intended settings.
Dynamic Content
Caching dynamic content presents another challenge. Content that frequently changes should not have a long max-age
, as it may lead to serving outdated content to the users.
Overriding Caching Directives
Sometimes, a downstream proxy server or a CDN may override the caching directives set by the origin server, leading to unexpected caching behavior.
An Example in Java
Let's take a look at how the max-age
directive can be set in a Java web application using the Spring Framework. In this example, we'll use Spring Boot to create a simple REST endpoint and set the caching directives for the response.
@RestController
public class MyController {
@GetMapping("/data")
public ResponseEntity<String> getData() {
HttpHeaders headers = new HttpHeaders();
headers.setCacheControl(CacheControl.maxAge(3600, TimeUnit.SECONDS).getHeaderValue());
return ResponseEntity.ok()
.headers(headers)
.body("This is some data to be cached for 1 hour");
}
}
In the above code snippet, we have a MyController
class with a getData
method that returns a simple string. We set the Cache-Control
header with a max-age
of 3600 seconds (1 hour) using the CacheControl.maxAge
method provided by Spring Framework.
Troubleshooting and Solutions
Verify Response Headers
To troubleshoot issues with the max-age
directive, start by inspecting the response headers using developer tools in the browser. Ensure that the Cache-Control
header contains the max-age
directive with the intended value.
Server-Side Configuration
Review the server-side configuration to guarantee that caching directives are being set correctly. If using frameworks such as Spring Boot, ensure that the caching directives are appropriately configured in the responses.
Dynamic Content Considerations
If dealing with dynamic content, carefully assess the caching strategy. Consider using a combination of max-age
and validation-based caching (e.g., ETag
or Last-Modified
) to handle dynamic content caching effectively.
Content Delivery Networks (CDNs) and Proxies
When using CDNs or proxies, be mindful of their caching behavior. They may override origin server caching headers, necessitating coordination between the origin server and these intermediary systems.
Final Considerations
Effective caching plays a pivotal role in enhancing the performance and user experience of web applications. The max-age
directive, as part of the HTTP caching headers, dictates the duration for which a cached resource can be considered fresh. By understanding common issues and employing best practices, such as proper syntax, dynamic content considerations, and server-side configurations, developers can ensure that the max-age
directive works seamlessly to optimize caching in their Java web applications.
Remember, proper caching not only boosts performance but also conserves server resources, making it a fundamental aspect of web development that should not be overlooked.
Checkout our other articles