Choosing the Right Data Structure for Your Project
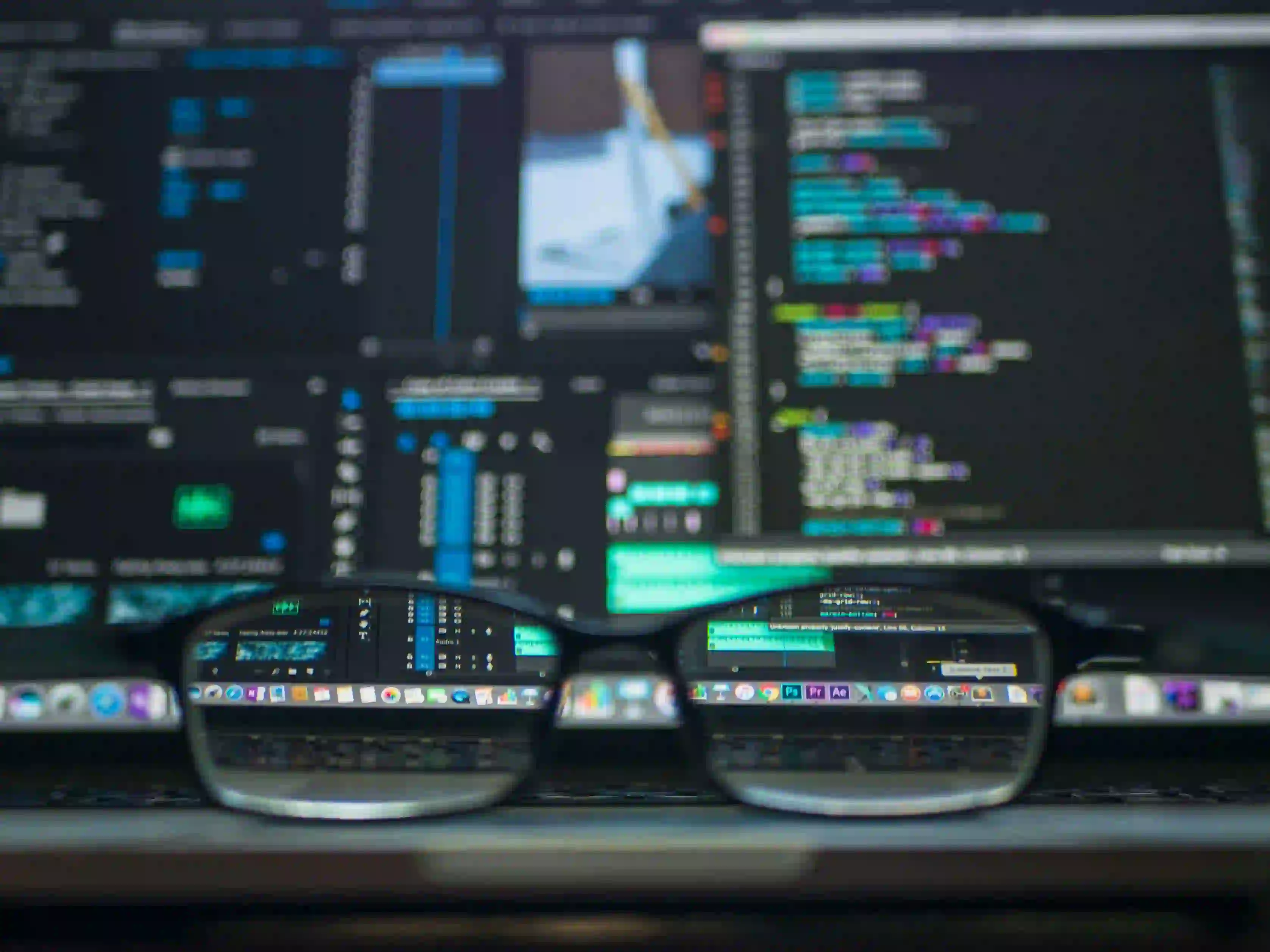
In the world of programming, data structures play a crucial role in organizing and storing data. The choice of a data structure can significantly impact the performance, scalability, and maintainability of your application. Understanding the different types of data structures and knowing when to use each one is essential for every Java developer.
Why Data Structures Matter
Data structures are at the core of computer science and are used in various domains, including database management, network protocols, and algorithm design. They provide a way to store and organize data so that it can be accessed and manipulated efficiently. Choosing the right data structure is vital because it can impact the overall performance and efficiency of your code.
The Java Collections Framework
Java provides a comprehensive Collections Framework that offers a wide range of data structures to choose from. This framework includes interfaces and classes for lists, sets, queues, and maps, along with various utility classes to manipulate collections. Understanding these data structures and their characteristics is essential for making an informed decision when writing Java applications.
Lists
Lists are ordered collections that allow duplicate elements. The ArrayList
and LinkedList
classes are commonly used implementations of the List
interface in Java.
The ArrayList
is a dynamic array that can dynamically grow and shrink, making it suitable for situations where random access and iteration are frequent operations. On the other hand, the LinkedList
is a doubly linked list that provides fast insertion and deletion, especially when dealing with large data sets.
Knowing when to use each type of list is crucial. For example, if your application requires frequent random access and traversal, ArrayList
might be a better choice due to its fast indexing capabilities. Conversely, if your application involves frequent insertion and deletion operations, especially with large data sets, LinkedList
may offer better performance.
Let's consider an example of selecting a list data structure to store a collection of user objects:
List<User> userList = new ArrayList<>(); // Choosing ArrayList for fast random access
In this example, the ArrayList
is chosen for its efficiency in random access scenarios.
Sets
Sets are collections that do not allow duplicate elements. The HashSet
and TreeSet
classes are commonly used implementations of the Set
interface in Java.
The HashSet
is implemented using a hash table and is the best-performing implementation for storing and retrieving elements. On the other hand, the TreeSet
is implemented using a red-black tree, providing ordered iteration of elements.
Choosing between HashSet
and TreeSet
depends on the specific requirements of your application. If you need constant-time performance for basic operations, such as add, remove, and contains, HashSet
is the go-to choice. However, if you require the elements to be ordered, TreeSet
is the appropriate option.
Consider the following example of using a set to store a collection of unique values:
Set<String> uniqueNames = new HashSet<>(); // Choosing HashSet for constant-time performance
In this scenario, HashSet
is preferred for its constant-time performance in ensuring uniqueness.
Maps
Maps are key-value pairs that do not allow duplicate keys. The HashMap
and TreeMap
classes are commonly used implementations of the Map
interface in Java.
The HashMap
provides constant-time performance for basic operations, such as get and put, making it suitable for scenarios where key-based operations are frequent. On the other hand, the TreeMap
provides a sorted order of the keys, useful for scenarios where iteration over the keys is required in a specific order.
Choosing between HashMap
and TreeMap
depends on the specific requirements of your application. If you need constant-time performance for key-based operations, HashMap
is the preferred choice. However, if you require the keys to be sorted, TreeMap
is the appropriate option.
Let's look at an example of using a map to store key-value pairs:
Map<String, Integer> ageMap = new HashMap<>(); // Choosing HashMap for constant-time key-based operations
In this example, HashMap
is chosen for its constant-time performance in key-based operations.
Custom Data Structures
While the Java Collections Framework provides a wide range of data structures, there may be specific scenarios where a custom data structure is needed to meet the unique requirements of an application.
For instance, if you are working on a specialized algorithm or data processing task that does not fit the standard collections, creating a custom data structure might be the most effective solution. This could involve designing a specific data structure tailored to the problem domain, thereby optimizing the performance and memory utilization for that particular use case.
Creating a custom data structure requires a deep understanding of data organization and manipulation. It involves defining the underlying data storage, determining the operations that can be performed on the structure, and ensuring the efficiency of these operations.
Below is an example of a simplified custom data structure CustomStack
that implements a stack using an array:
public class CustomStack {
private int[] array;
private int top;
public CustomStack(int capacity) {
array = new int[capacity];
top = -1;
}
public void push(int value) {
// Implementing the push operation
}
public int pop() {
// Implementing the pop operation
}
// Other operations
}
In this example, we have defined a custom data structure CustomStack
to implement a stack using an array. The choice to create a custom data structure in this scenario is driven by the need for a highly efficient stack tailored to the specific application requirements.
A Final Look
Choosing the right data structure is a critical decision that can significantly impact the performance and efficiency of a Java application. Understanding the characteristics and use cases of different data structures, whether from the Java Collections Framework or custom implementations, is essential for making informed choices.
When deciding on a data structure, consider the specific requirements of your application, such as the frequency of operations, the nature of data manipulation, and the desired performance characteristics. By selecting the most appropriate data structure, you can ensure that your application performs optimally and scales effectively as the data grows.
By diving deeper into the details and nuances of each data structure, you can elevate your programming skills and make better-informed decisions in your Java projects.