Explicit vs. Default Constructors: Which to Choose?
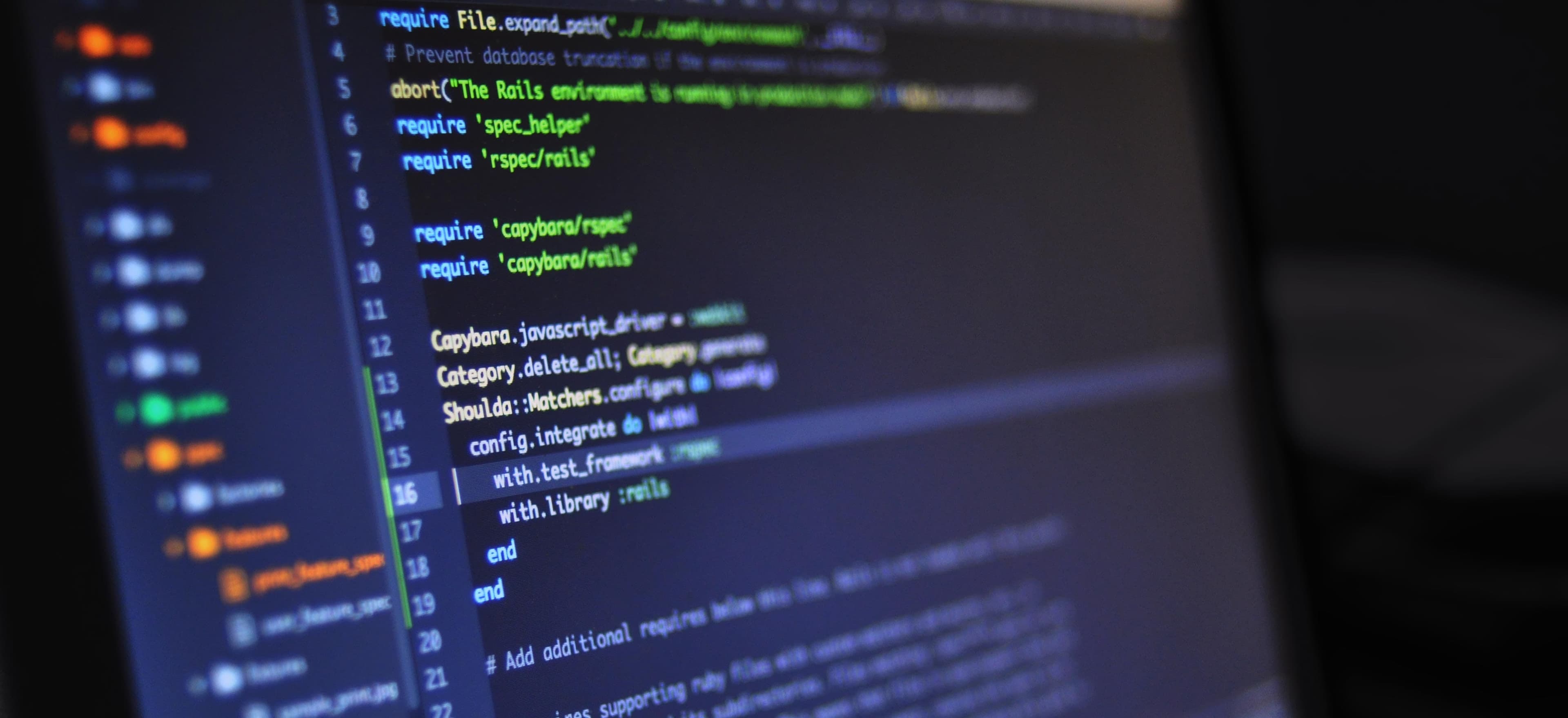
- Published on
Explicit vs. Default Constructors: Which to Choose?
In Java, constructors play a critical role in initializing objects. When designing a class, one of the fundamental decisions you'll make is whether to utilize an explicit constructor or a default constructor. Each type has its own unique benefits and use cases, and understanding these differences is essential for writing effective and maintainable code.
What Is a Constructor?
A constructor is a special method that is invoked when an object of a class is created. The primary purpose of a constructor is to initialize the object's attributes with specific values.
Types of Constructors in Java
There are two main types of constructors in Java:
-
Default Constructor: This is automatically generated by Java if no constructors are explicitly defined in your class. It does not require any parameters and initializes object attributes with their default values (e.g.,
0
for integers,null
for objects). -
Explicit Constructor: This constructor is defined by a programmer and can have parameters. It allows for customization of the object’s state upon creation.
Understanding Default Constructors
Basics of Default Constructors
Here’s a simple code snippet demonstrating a default constructor:
public class Person {
String name;
int age;
// Default constructor
public Person() {
this.name = "Unknown";
this.age = 0;
}
}
Why Use a Default Constructor?
- Simplicity: When a class does not require specific initial values, a default constructor can streamline object creation.
- Flexibility: If you plan to create instances where attributes are filled later (e.g., from user input), a default constructor is a suitable choice.
When to Avoid Default Constructors
In classes where certain attributes must always have a value, a default constructor can lead to incorrect states. For instance, if you want to ensure that a Person
always has a defined name, using a default constructor without parameters might not be appropriate:
public class Person {
String name;
int age;
// This approach is not ideal if 'name' shouldn't stay 'Unknown'.
public Person() {
this.name = "Unknown";
this.age = 0; // Default age is set but may not be valid
}
}
Exploring Explicit Constructors
Basics of Explicit Constructors
Explicit constructors allow for tailored initialization. Here’s a snippet showcasing an explicit constructor:
public class Person {
String name;
int age;
// Explicit constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
Why Use an Explicit Constructor?
- Controlled Initialization: Explicit constructors need parameters for creation, ensuring that the important attributes are always initialized.
- Enhanced Flexibility: You can create multiple overloaded constructors to accommodate various initialization options.
Example of Overloading Constructors
public class Person {
String name;
int age;
// Default constructor
public Person() {
this.name = "Unknown";
this.age = 0;
}
// Explicit constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Constructor for only name, setting age to 18 by default
public Person(String name) {
this.name = name;
this.age = 18;
}
}
In this example, the Person
class has three constructors:
- A default constructor
- An explicit constructor requiring both a name and age
- An overloaded constructor that requires just a name, with a default age of 18.
Performance Considerations
While the choice between explicit and default constructors may not significantly impact performance, it can affect readability and maintainability. When initializing complex objects, consider whether an explicit constructor might reduce the chances of creating an object in an invalid state.
Best Practices: When to Use Each Type
Use a Default Constructor When:
- You want to provide flexibility for later initialization.
- You’re working with mutable classes where initial states are not crucial.
Use an Explicit Constructor When:
- Specific attributes are mandatory for the functioning of your object.
- You need to enforce certain validation and conditions upon object creation.
- Overloading is beneficial for providing multiple options for constructing objects.
Final Thoughts
Ultimately, the choice between using an explicit constructor or a default constructor depends on your specific use case. An explicit constructor offers more control and ensures objects are created in a valid state, while a default constructor provides simplicity and flexibility.
Understanding these distinctions can lead to better object-oriented design and more intuitive code. For those interested in delving deeper into advanced Java concepts, resources like GeeksforGeeks offer extensive explanations and examples on constructors.
By tailoring your decision to the needs of your application, you can write cleaner and more effective Java code. Remember, constructors are the first step in creating robust objects—choose wisely!