Optimizing Spring Data Project with Apache Cassandra
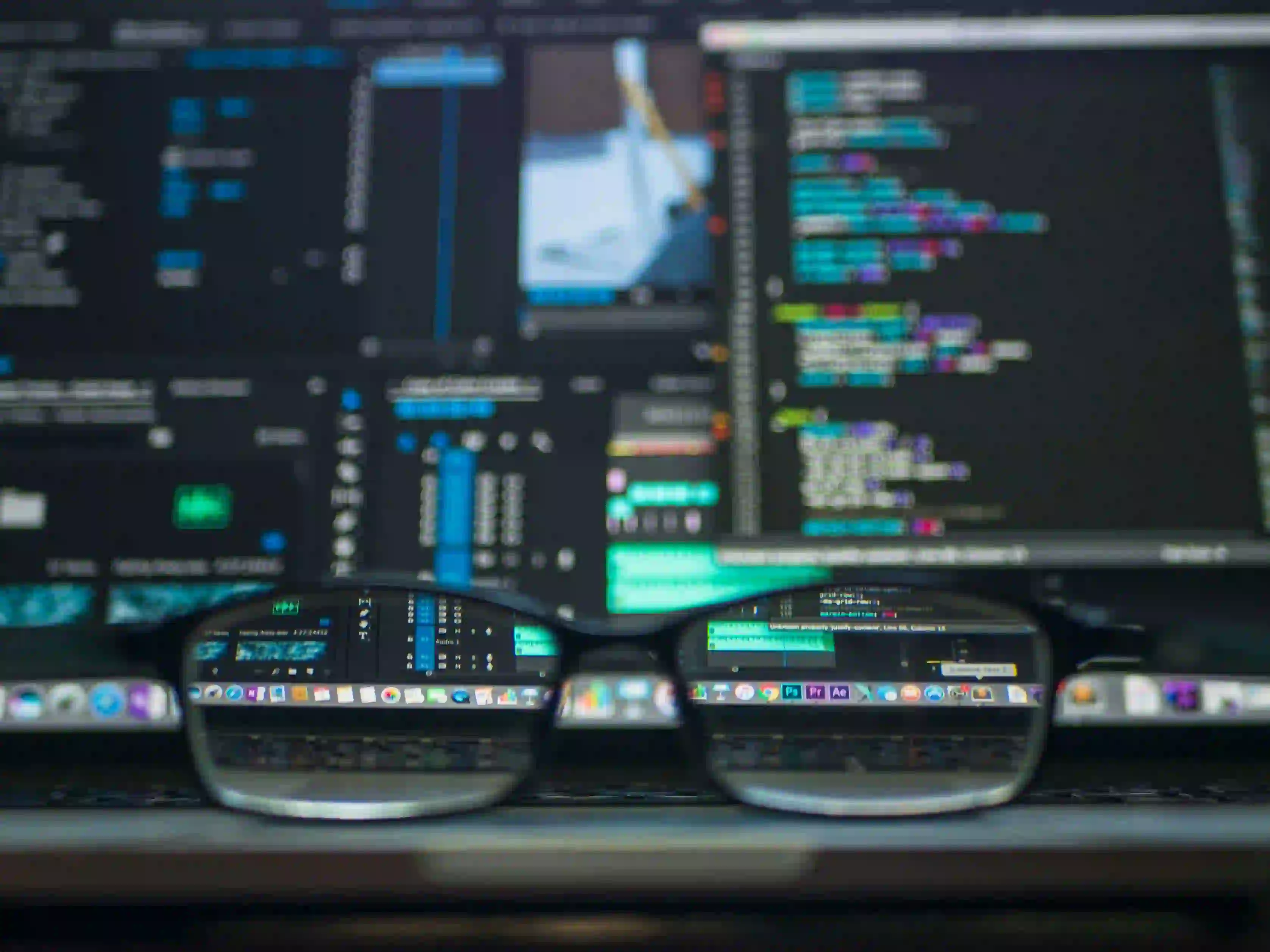
Optimizing Spring Data Project with Apache Cassandra
In today's data-driven world, managing large volumes of data efficiently is crucial for the success of any application. When it comes to handling big data, Apache Cassandra is a popular choice due to its distributed architecture and scalability. Integrating Apache Cassandra with a Spring Data project can further enhance its performance and reliability.
In this article, we will explore how to optimize a Spring Data project by leveraging the capabilities of Apache Cassandra. We'll delve into various techniques and best practices to improve the performance of a Spring Data application using Apache Cassandra as the data store.
Why Apache Cassandra?
Apache Cassandra is a highly scalable and fault-tolerant NoSQL database that is well-suited for managing large amounts of data across multiple nodes. It provides high availability and can handle petabytes of information with ease. Cassandra's decentralized architecture ensures that there is no single point of failure, making it a robust choice for distributed applications.
When integrated with a Spring Data project, Apache Cassandra can bring its strengths to the table, offering fast read and write operations, support for large datasets, and seamless scalability.
Setting Up Apache Cassandra with Spring Data
To get started, you'll need to have Apache Cassandra installed and running. You can refer to the official Apache Cassandra documentation for installation instructions.
Once Cassandra is up and running, you can begin integrating it with your Spring Data project. Spring Data provides seamless integration with Cassandra through the spring-data-cassandra
module. You can add the necessary dependencies to your project's pom.xml
(if using Maven) or build.gradle
(if using Gradle) file.
Maven Dependency
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-cassandra</artifactId>
</dependency>
Gradle Dependency
implementation 'org.springframework.boot:spring-boot-starter-data-cassandra'
After adding the dependency, you can configure the connection to the Cassandra database in your Spring Boot application properties. Specify the Cassandra host, port, keyspace, and any other required configurations.
spring:
data:
cassandra:
contact-points: localhost
port: 9042
keyspace-name: mykeyspace
With the setup in place, you can start leveraging the features of Apache Cassandra within your Spring Data project.
Optimizing Data Modeling
One of the key aspects of optimizing a Spring Data project with Apache Cassandra is designing an efficient data model. Cassandra's data modeling revolves around queries, where the schema is designed based on the application's query patterns rather than the normalized form used in traditional databases.
Denormalization
In Apache Cassandra, denormalization is a common practice where data is duplicated and stored in multiple tables to optimize read performance. By denormalizing the data, you can reduce the number of joins required for query execution, resulting in faster reads.
Let's consider an example where we have a users
table and a posts
table in a social media application. In a relational database, these might be separate tables with foreign key relationships. However, in Cassandra, we could denormalize the data and store the user information alongside each post to minimize the need for joins when fetching posts for a user.
@Table
public class User {
@PrimaryKey
private UUID userId;
private String username;
// other user data
}
@Table
public class Post {
@PrimaryKey
private UUID postId;
private UUID userId;
private String username; // denormalized from User table
private String content;
// other post data
}
In this example, the username
field from the User
table is denormalized and stored within the Post
table, allowing us to retrieve posts along with user information in a single query.
Composite Keys
In Cassandra, primary keys are crucial for data retrieval. Using composite keys, which are keys composed of multiple columns, can optimize query performance by allowing efficient retrieval based on multiple criteria.
@Table
public class SensorData {
@PrimaryKey
private String sensorId;
@PrimaryKey
private Date timestamp;
// sensor data fields
}
In this example, the SensorData
table uses a composite primary key consisting of sensorId
and timestamp
, enabling queries that filter data based on both the sensor ID and the timestamp, without the need for secondary indexes.
By carefully designing your data model to suit the application's query patterns, you can significantly enhance the performance of your Spring Data project with Apache Cassandra.
Query Optimization
Efficient querying is paramount for maximizing the benefits of Apache Cassandra. Understanding how queries are executed and optimizing them accordingly is crucial for achieving high performance.
Use of Secondary Indexes
While Cassandra discourages the use of secondary indexes due to their impact on performance, there are scenarios where they might be necessary. If you find the need to query based on non-primary key columns, consider using SASI (SSTable Attached Secondary Indexes) or DSE (DataStax Enterprise) search indexes, which offer improved index performance compared to traditional Cassandra secondary indexes.
@Indexed
private String email;
In this example, the email
field is annotated with @Indexed
, indicating that a secondary index should be created for efficient querying based on the email column.
Materialized Views
Materialized views in Cassandra allow you to precompute query results and store them as separate tables, thereby optimizing read performance for specific query patterns. By creating materialized views, you can minimize the need for ad-hoc querying and ensure faster data retrieval.
@MaterializedView
@Query("SELECT sensorId, timestamp, value FROM sensorData WHERE sensorId IS NOT NULL AND timestamp IS NOT NULL")
public class SensorDataBySensorIdAndTime { }
In this example, a materialized view is created to store sensor data based on the sensor ID and timestamp, facilitating efficient retrieval of sensor data without the need for complex querying.
Caching and Performance Tuning
Caching can play a significant role in optimizing the performance of a Spring Data project with Apache Cassandra. By caching frequently accessed data, you can reduce the load on the database and improve overall response times.
Use of Caching Providers
Integrating a caching provider such as Redis or Memcached can bring significant performance improvements by storing frequently accessed data in memory. Spring Data provides seamless integration with caching providers through its caching annotations.
@Cacheable("posts")
public Post getPostById(UUID postId) {
// fetch post from Cassandra
}
In this example, the @Cacheable
annotation instructs Spring Data to cache the result of the getPostById
method, reducing the need to fetch the same post repeatedly from the Cassandra database.
Tuning Consistency Levels
Cassandra offers tunable consistency levels, allowing you to balance between data consistency and performance. By carefully choosing the appropriate consistency level for read and write operations, you can optimize the performance of your Spring Data application.
QueryOptions queryOptions = new QueryOptions().setConsistencyLevel(ConsistencyLevel.LOCAL_QUORUM);
In this example, the LOCAL_QUORUM
consistency level is set for a query, ensuring strong consistency within the local data center while optimizing performance.
The Bottom Line
Optimizing a Spring Data project with Apache Cassandra involves a combination of efficient data modeling, query optimization, and performance tuning. By leveraging the strengths of Apache Cassandra and adhering to best practices, you can achieve high-performance data access and storage within your Spring Data application.
In this article, we've covered various aspects of optimizing a Spring Data project with Apache Cassandra, including data modeling techniques, query optimization strategies, and performance tuning measures. By implementing these optimizations, you can harness the full potential of Apache Cassandra and enhance the overall performance and scalability of your Spring Data application.
Apache Cassandra offers a plethora of features and optimizations, and its integration with Spring Data presents a compelling solution for building robust, high-performance applications in the modern data landscape.
Remember, achieving optimal performance is an iterative process, and continuous monitoring and refinement are essential for maintaining the efficiency of your Spring Data project with Apache Cassandra.
Now that you have a deeper understanding of optimizing Spring Data with Apache Cassandra, apply these techniques to your projects and witness the transformative impact on performance and scalability. Happy coding!
For further information and resources, feel free to explore the following references:
- Spring Data Cassandra Documentation
- Cassandra Query Language (CQL) Documentation
- DataStax Enterprise (DSE) Search Indexes
- Redis Documentation
Remember, the key to successful optimization lies in understanding your application's specific requirements and implementing tailored solutions to meet them effectively.