Optimizing Spring Thread Pool for Efficient Service Execution
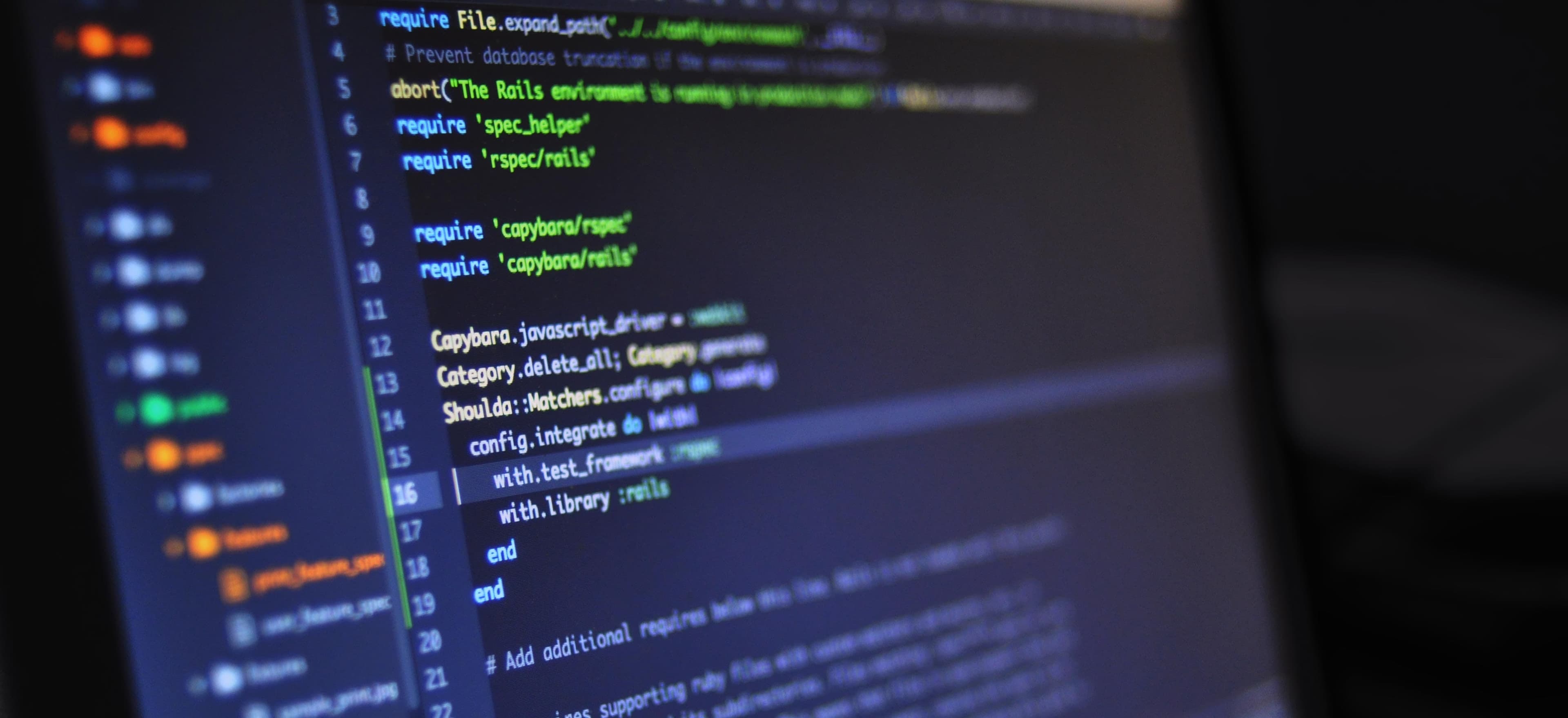
- Published on
Optimizing Spring Thread Pool for Efficient Service Execution
In a multi-threaded environment, configuring the thread pool is crucial for achieving efficient service execution. Spring Framework provides a powerful thread pool abstraction that allows developers to manage thread pools effectively. In this blog post, we will discuss how to optimize the Spring thread pool for efficient service execution.
Understanding the Basics of ThreadPoolTaskExecutor
In Spring Framework, the ThreadPoolTaskExecutor
class provides a convenient way to set up and manage a thread pool. It allows you to customize core pool size, maximum pool size, queue capacity, and various other settings to control the behavior of the thread pool.
@Configuration
@EnableAsync
public class AppConfig implements AsyncConfigurer {
@Override
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(20);
executor.setQueueCapacity(30);
executor.setThreadNamePrefix("MyExecutor-");
executor.initialize();
return executor;
}
}
In the above code snippet, we are configuring a ThreadPoolTaskExecutor
with a core pool size of 10, a maximum pool size of 20, a queue capacity of 30, and a thread name prefix of "MyExecutor-".
Optimizing Core Pool Size
The core pool size represents the number of threads that will be kept alive in the thread pool, even if they are idle. It is crucial to set an appropriate core pool size based on the nature of the tasks being executed.
Setting the core pool size too low may result in the creation of new threads for every incoming task, leading to increased overhead due to thread creation and teardown. On the other hand, setting the core pool size too high may consume unnecessary resources.
executor.setCorePoolSize(10);
By carefully analyzing the nature of your tasks and the available system resources, you can set an optimal core pool size that allows the thread pool to efficiently handle the incoming workload without unnecessary resource consumption.
Optimizing Maximum Pool Size
The maximum pool size determines the upper limit of threads that can be created in the thread pool to handle incoming tasks. It is essential to set an appropriate maximum pool size to prevent the thread pool from creating an excessive number of threads under heavy load.
executor.setMaxPoolSize(20);
A high maximum pool size may lead to resource exhaustion under extreme load conditions, while a low maximum pool size may result in tasks being rejected when the pool is saturated. By understanding the characteristics of your workload and system resources, you can set an optimal maximum pool size to ensure efficient utilization of resources without risking exhaustion.
Optimizing Queue Capacity
The queue capacity determines the maximum number of tasks that can be held in the queue waiting for execution when all threads are busy. It is crucial to choose an appropriate queue capacity to balance the load on the thread pool and prevent task rejection.
executor.setQueueCapacity(30);
A small queue capacity may lead to task rejection under heavy load, while a large queue capacity may cause excessive memory consumption and potential OutOfMemoryError. By understanding the workload characteristics and memory constraints, you can set an optimal queue capacity to ensure efficient task management without risking memory exhaustion.
Throttling Task Submission
In a highly concurrent system, it is essential to consider throttling task submission to prevent overwhelming the thread pool with a large number of incoming tasks. By introducing a submission throttle mechanism, such as a bounded queue or a rate limiter, you can control the rate of task submission to the thread pool.
@Service
public class TaskService {
@Autowired
private ThreadPoolTaskExecutor taskExecutor;
public void submitTask(Runnable task) {
taskExecutor.execute(task);
}
}
In the above code snippet, the TaskService
class utilizes a ThreadPoolTaskExecutor
to execute tasks. By implementing a submission throttle mechanism in the submitTask
method, you can control the rate at which tasks are submitted to the thread pool, preventing it from being overwhelmed.
Monitoring and Tuning
Monitoring the performance of the thread pool is essential for identifying potential bottlenecks and optimizing its configuration. You can utilize tools like JVisualVM, Java Mission Control, or Spring Boot Actuator to monitor the thread pool's behavior, including thread count, task throughput, and queue usage.
Based on the monitoring insights, you can fine-tune the thread pool configuration to align with the dynamically changing workload and system characteristics. It is essential to regularly review and adjust the thread pool settings to ensure optimal performance and resource utilization.
Bringing It All Together
Optimizing the Spring thread pool for efficient service execution is crucial for achieving high performance and resource utilization in a multi-threaded application. By carefully configuring core pool size, maximum pool size, queue capacity, and implementing submission throttling, you can ensure that the thread pool effectively handles the incoming workload without risking resource exhaustion or task rejection.
Remember, it's essential to continuously monitor the thread pool's performance and fine-tune its configuration based on the dynamic nature of the workload and system resources.
In conclusion, by optimizing the Spring thread pool, you can achieve efficient service execution and maintain high-performance standards in your multi-threaded applications.
Continue to refine your understanding of thread pool optimization by exploring more about Spring's Task Execution and Scheduling Documentation.
Would you consider implementing these optimizations in your Spring application to boost performance?