Optimizing Unity3D Simulation Performance
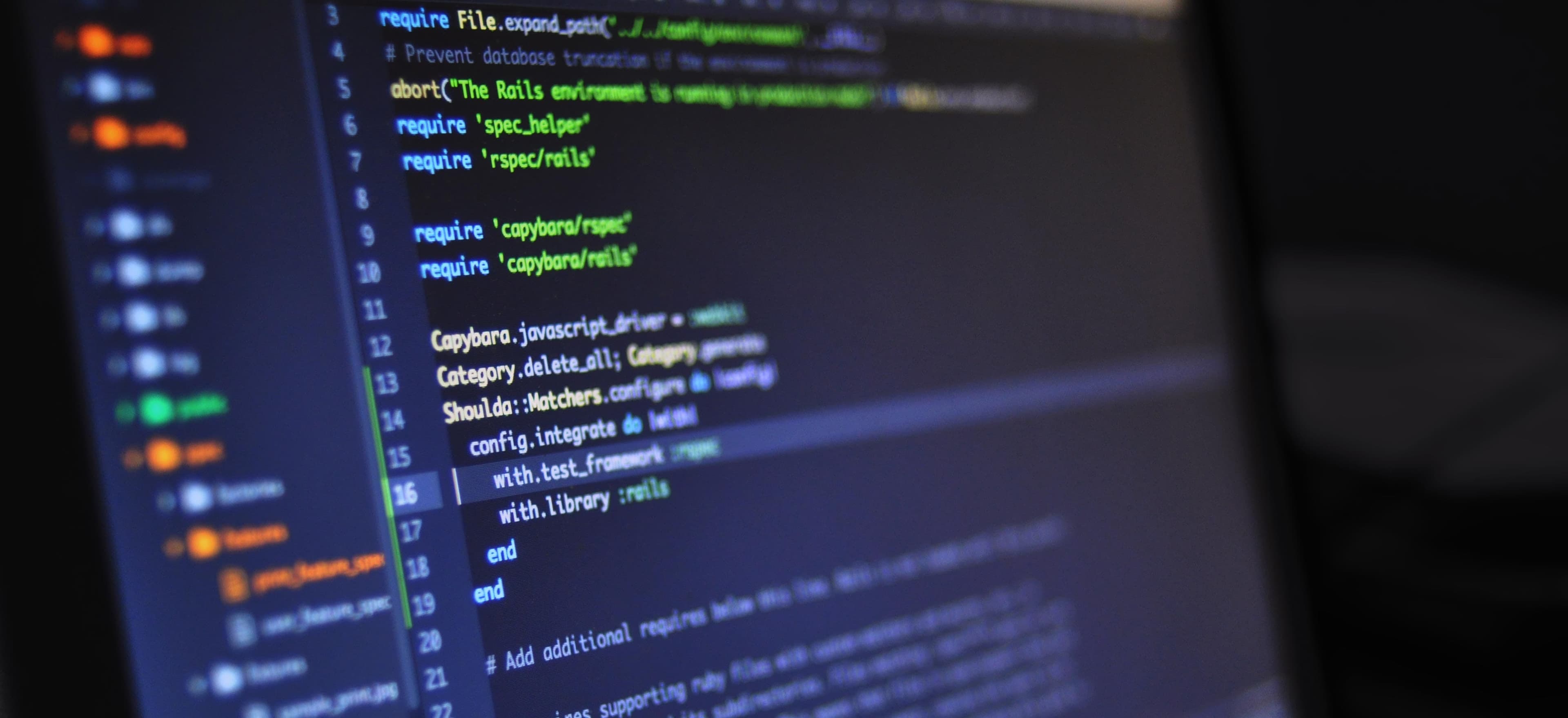
- Published on
Optimizing Unity3D Simulation Performance
Unity3D is a powerful game development platform that provides developers with a robust set of tools for creating immersive simulations and games. However, as simulations and games become more complex, optimizing performance becomes increasingly important. In this blog post, we will explore some key strategies for optimizing the performance of Unity3D simulations, focusing on Java-specific optimizations.
1. Use Object Pooling for Efficient Memory Management
In Java, object instantiation can be an expensive operation due to the overhead of memory allocation and garbage collection. In Unity3D, this can lead to performance hiccups and potential frame rate drops. Object pooling is a technique where a set of pre-initialized objects are kept ready for use, thus avoiding the overhead of creating and destroying objects frequently.
public class ObjectPool : MonoBehaviour
{
public GameObject prefab;
public int poolSize = 10;
private List<GameObject> pool;
void Start()
{
pool = new List<GameObject>();
for (int i = 0; i < poolSize; i++)
{
GameObject obj = Instantiate(prefab);
obj.SetActive(false);
pool.Add(obj);
}
}
public GameObject GetObjectFromPool()
{
for (int i = 0; i < pool.Count; i++)
{
if (!pool[i].activeInHierarchy)
{
pool[i].SetActive(true);
return pool[i];
}
}
return null;
}
}
In this example, we create an object pool that instantiates a set number of objects and keeps them ready for use. When an object is needed, it is retrieved from the pool rather than instantiating a new one. This can significantly reduce memory allocation and improve performance.
2. Efficient Data Structures and Algorithms
In Java, using the right data structure and algorithm can have a significant impact on performance. The same holds true for Unity3D simulations. For example, when dealing with a large number of game objects, using the most efficient data structure such as spatial partitioning (e.g., Quadtree or Octree) can greatly enhance performance.
3. Minimize Garbage Collection
Excessive garbage collection can cause performance spikes and hiccups in Unity3D simulations. To minimize garbage collection, it's important to avoid unnecessary object allocations and deallocations. Caching frequently used objects and reusing them can help reduce the frequency of garbage collection.
String result = "";
for (int i = 0; i < 1000; i++) {
result += "data"; // Avoid string concatenation in loops
}
In the above example, using a StringBuilder
instead of string concatenation in a loop can reduce memory overhead and unnecessary object allocations, thus minimizing garbage collection.
4. Use Asynchronous Processing
In situations where heavy computations or operations are required, using asynchronous processing can prevent the simulation from locking up. In Unity3D, the AsyncOperation
class can be used for tasks that need to be processed in the background.
IEnumerator LoadLevelAsync(int levelIndex)
{
AsyncOperation asyncLoad = SceneManager.LoadSceneAsync(levelIndex);
while (!asyncLoad.isDone)
{
float progress = Mathf.Clamp01(asyncLoad.progress / 0.9f);
// Update progress bar or perform other tasks
yield return null;
}
}
By using asynchronous processing for tasks such as level loading, resource loading, or data processing, the main simulation thread remains responsive, thus improving overall performance.
5. Optimizing Rendering
Efficient rendering is crucial for achieving good performance in Unity3D simulations. Techniques such as occlusion culling, level of detail (LOD) management, and minimizing the number of draw calls can significantly improve rendering performance.
void Start()
{
// Enable occlusion culling
Camera.main.useOcclusionCulling = true;
// Manage LOD settings
QualitySettings.SetQualityLevel(2);
// Batch draw calls where possible
// ...
}
By enabling occlusion culling, managing LOD settings, and batching draw calls, we can reduce the rendering workload and optimize performance.
In Conclusion, Here is What Matters
Optimizing performance in Unity3D simulations is crucial for delivering a smooth and immersive user experience. By leveraging Java-specific optimizations such as object pooling, efficient data structures, minimizing garbage collection, asynchronous processing, and optimizing rendering, developers can ensure that their Unity3D simulations run smoothly and efficiently.
For more in-depth information on Unity3D optimization techniques, Unity3D's official performance optimization guide provides additional insights and best practices.
Implementing these optimizations requires careful consideration of the specific requirements and constraints of each simulation, but with the right approach, developers can create high-performance Unity3D simulations that push the boundaries of what is possible in the realm of virtual experiences.
Remember, the key to successful optimization lies in profiling, identifying bottlenecks, and strategically applying the most suitable optimization techniques.
Happy optimizing!