Optimizing Performance in Distributed System Development
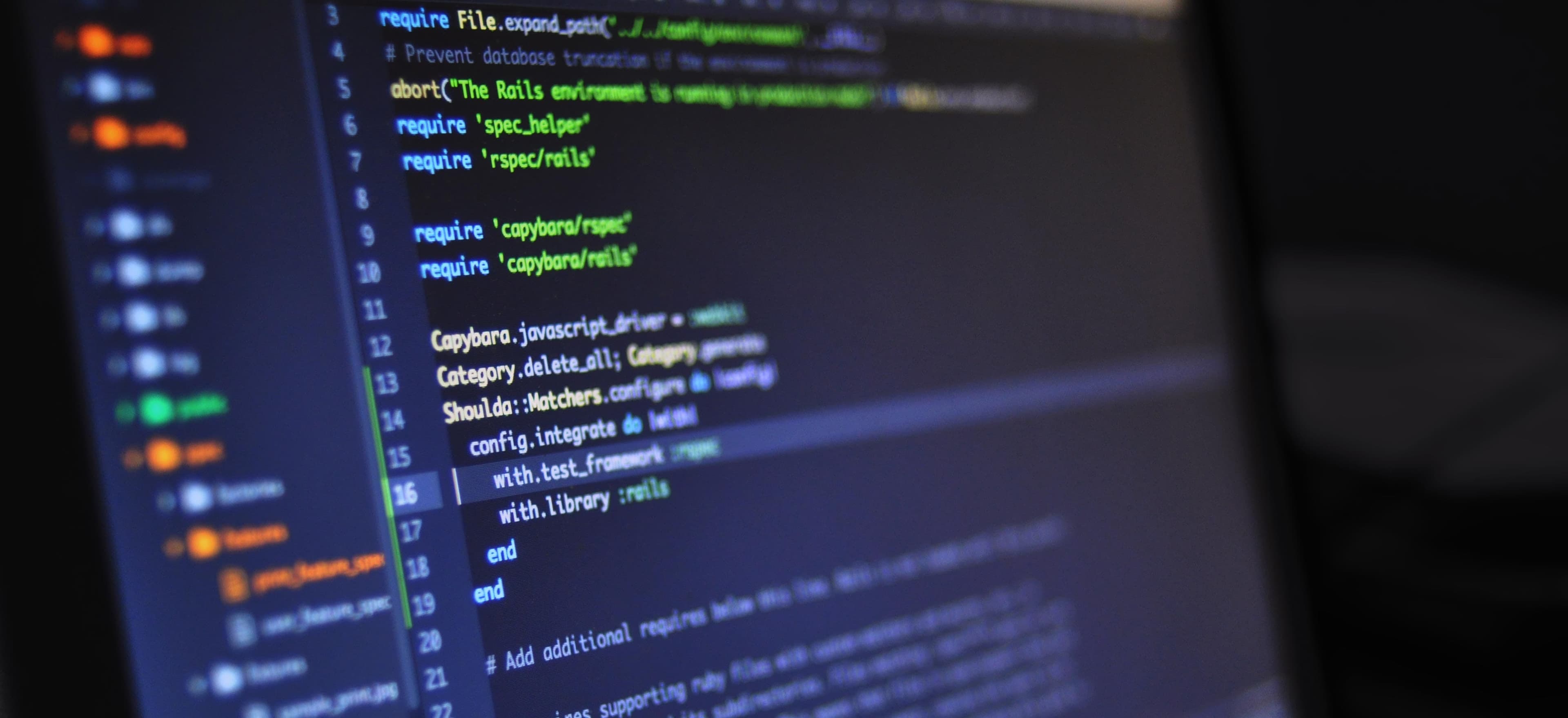
- Published on
Optimizing Performance in Distributed System Development
When it comes to optimizing performance in distributed system development, Java plays a crucial role. Java is a versatile programming language that is widely used for developing distributed systems due to its platform independence, strong networking capabilities, and extensive ecosystem of libraries and tools. In this blog post, we will explore some best practices for optimizing performance in distributed system development using Java.
Understanding the Challenges
Developing distributed systems presents unique challenges, such as network latency, concurrency control, and data consistency. These challenges become more pronounced as the system scales. In a distributed environment, it is essential to minimize latency, maximize throughput, and ensure fault tolerance.
Use of Asynchronous Programming
Asynchronous programming is crucial for optimizing performance in distributed systems. Java provides robust support for asynchronous programming through libraries like CompletableFuture and RxJava. By leveraging asynchronous programming, developers can design systems that efficiently utilize resources and handle concurrent requests without blocking threads.
Example of Using CompletableFuture in Java
CompletableFuture.supplyAsync(() -> {
// Asynchronous task implementation
return result;
}).thenAcceptAsync(result -> {
// Handle the result
});
In the above example, we use CompletableFuture
to perform an asynchronous task and then handle the result asynchronously. This approach allows the system to continue processing other tasks while waiting for the asynchronous operation to complete.
Efficient Network Communication
Efficient network communication is vital for the performance of distributed systems. Java provides robust support for network communication through its networking APIs. When designing network communication protocols, it is essential to minimize the number of round trips and reduce the amount of data transmitted.
Example of Efficient Network Communication in Java
// Create a socket and establish a connection
Socket socket = new Socket("hostname", port);
// Get the input and output streams for the socket
InputStream inputStream = socket.getInputStream();
OutputStream outputStream = socket.getOutputStream();
// Write data to the output stream
outputStream.write(data);
// Read data from the input stream
byte[] buffer = new byte[1024];
int bytesRead = inputStream.read(buffer);
In the above example, we demonstrate efficient network communication using Java's Socket
class. By minimizing the number of round trips and efficiently transmitting data, we can enhance the performance of the distributed system.
Effective Resource Management
Efficient resource management is critical in distributed system development. Managing resources such as threads, database connections, and file handles can have a significant impact on system performance. Java provides powerful tools for resource management, such as the Executor framework for thread management and connection pooling libraries for database connections.
Example of Using Executor Framework in Java
// Create an executor with a fixed thread pool
ExecutorService executor = Executors.newFixedThreadPool(10);
// Execute tasks using the executor
executor.execute(() -> {
// Task implementation
});
In the above example, we utilize the Executor framework to manage a pool of threads, ensuring efficient utilization of resources and improved system performance.
Effective Caching Strategies
Caching plays a crucial role in optimizing the performance of distributed systems. By caching frequently accessed data, we can reduce the load on backend systems and minimize latency. Java provides robust caching libraries such as Ehcache and Caffeine, which offer features like expiration policies, eviction strategies, and automatic loading of cached data.
Example of Using Ehcache in Java
// Create a cache manager
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder().build();
cacheManager.init();
// Create a cache with specific settings
Cache<String, String> cache = cacheManager.createCache("myCache",
CacheConfigurationBuilder.newCacheConfigurationBuilder(String.class, String.class,
ResourcePoolsBuilder.heap(100)).build());
// Put data into the cache
cache.put("key", "value");
// Get data from the cache
String value = cache.get("key");
In the above example, we demonstrate the use of Ehcache to create and utilize a cache for storing and retrieving data. By implementing effective caching strategies, we can significantly enhance the performance of distributed systems.
Key Takeaways
Optimizing performance in distributed system development requires careful consideration of various factors such as asynchronous programming, network communication, resource management, and caching strategies. Java provides a rich set of tools and libraries that empower developers to address these challenges effectively. By incorporating best practices and leveraging Java's capabilities, developers can build high-performing distributed systems that meet the demands of modern applications.
In conclusion, optimizing performance in distributed system development is an ongoing effort that requires a deep understanding of the underlying principles and a proactive approach to performance tuning. With the right strategies and tools, developers can build distributed systems that deliver exceptional performance and reliability.
Remember, performance optimization is not a one-time task but an ongoing process that should be integrated into the development lifecycle. By continually monitoring and improving the performance of distributed systems, developers can ensure that their applications meet the demands of today's dynamic and highly distributed computing environments.
For further insights on optimizing Java performance in distributed system development, check out this comprehensive guide on effective caching strategies in Java.
Happy optimizing!