Maximizing Productivity: The Kanban Approach
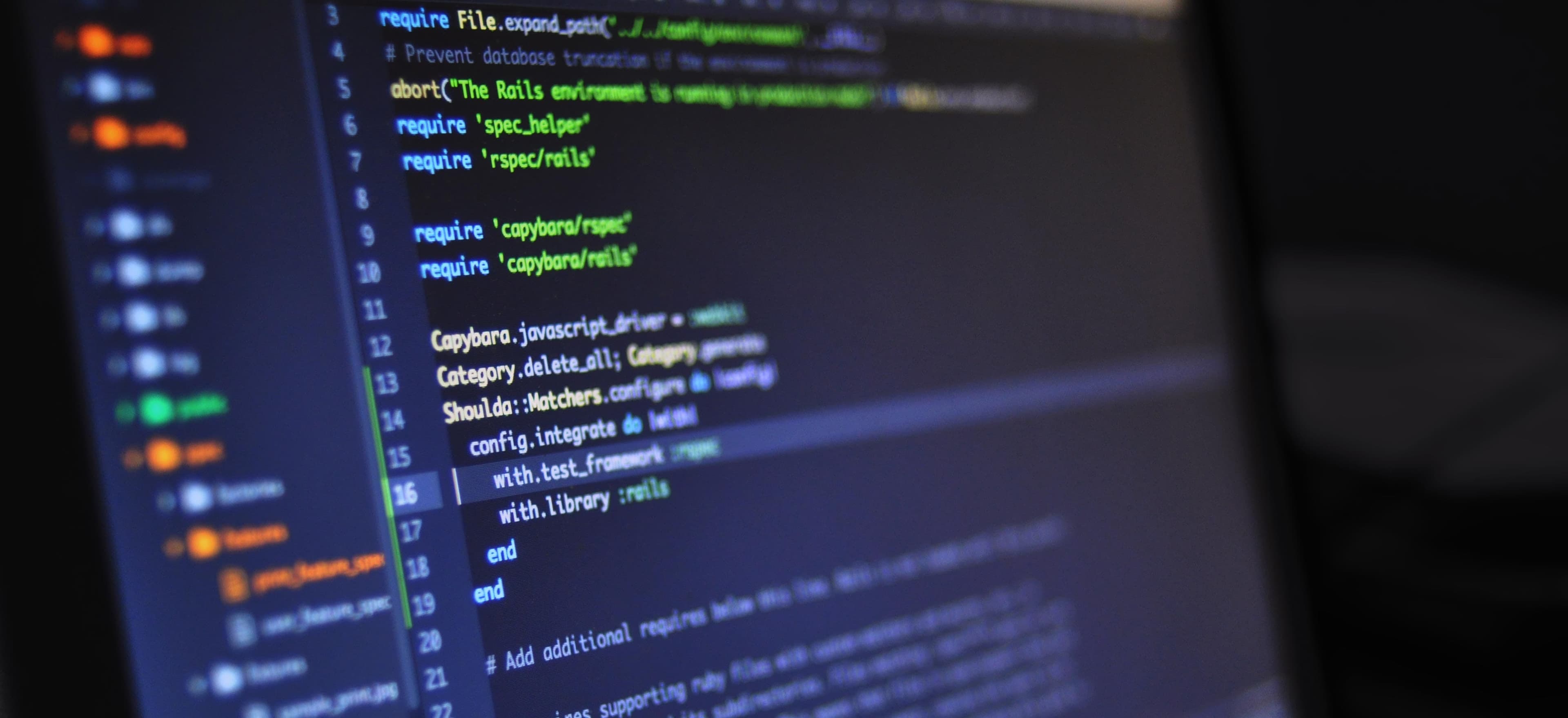
- Published on
Maximizing Productivity: The Kanban Approach
In today's fast-paced software development industry, maximizing productivity is essential for success. One methodology that has gained popularity for its effectiveness in improving workflow efficiency is Kanban. Originally developed by Toyota in the 1940s, Kanban has since been adapted and adopted by numerous industries, including software development.
What is Kanban?
Kanban, which means "visual signal" or "card" in Japanese, is a lean management system designed to optimize workflow processes. At its core, Kanban emphasizes visualization of work, limiting work in progress (WIP), and continuous improvement. These principles make it an ideal approach for software development teams seeking to streamline their processes and enhance productivity.
The Basics of Kanban
1. Visualization of Work
Kanban utilizes a Kanban board, which is a visual representation of the workflow. The board is divided into columns that represent different stages of the workflow, such as "Backlog," "In Progress," and "Done." Each task or user story is represented by a card that moves across the board as it progresses through the workflow.
2. Limiting Work in Progress (WIP)
One of the key principles of Kanban is limiting the amount of work that can be in progress at any given time. By setting WIP limits for each stage of the workflow, teams can prevent overloading and focus on completing tasks before pulling in new work.
3. Continuous Improvement
Kanban encourages a culture of continuous improvement. Team members are empowered to identify bottlenecks, implement changes, and regularly reflect on their processes to make incremental improvements over time.
Implementing Kanban with Java
Now, let's explore how the principles of Kanban can be applied in the context of Java development.
1. Visualization with a Kanban Board
To implement a Kanban board for Java development, we can use a variety of tools and frameworks. One popular choice is to leverage the capabilities of JIRA, a versatile project management tool. JIRA provides customizable Kanban boards that allow teams to visualize their Java development workflow efficiently.
// Example of creating a new JIRA Kanban board for Java development
public class KanbanBoard {
public static void main(String[] args) {
JiraClient jiraClient = new JiraClient("https://yourcompany.atlassian.net", "username", "password");
KanbanBoard javaKanbanBoard = new KanbanBoard("Java Development", jiraClient);
javaKanbanBoard.createBoard();
}
}
In this example, we demonstrate the creation of a new Kanban board specifically tailored for Java development using JIRA's API. This board can then be customized to reflect the stages of the Java development workflow, providing clear visualization for the team.
2. Limiting Work in Progress (WIP) with Java
In Java development, we can enforce WIP limits programmatically to prevent excessive multitasking and maintain focus on completing tasks. Let's consider a simplified example where we use a queue to represent the work in progress, and we enforce a WIP limit of 3 tasks.
import java.util.Queue;
import java.util.LinkedList;
public class WipLimitExample {
private static final int WIP_LIMIT = 3;
private Queue<String> workInProgress = new LinkedList<>();
public void addToWip(String task) {
if (workInProgress.size() < WIP_LIMIT) {
workInProgress.add(task);
System.out.println("Task added to WIP: " + task);
} else {
System.out.println("WIP limit reached. Cannot add more tasks at the moment.");
}
}
public void removeFromWip(String task) {
if (workInProgress.remove(task)) {
System.out.println("Task removed from WIP: " + task);
} else {
System.out.println("Task not found in WIP.");
}
}
}
In this code snippet, we illustrate a simple mechanism for enforcing a WIP limit in a Java program. By maintaining a queue and checking the WIP limit before adding new tasks, we can emulate WIP constraints within our development process.
3. Continuous Improvement in Java Development
Continuous improvement is pivotal in Kanban, and the same holds true for Java development. Tools such as JUnit and Mockito can be utilized to create comprehensive test suites and perform test-driven development (TDD) to ensure code quality and maintainability.
Moreover, adopting a version control system like Git enables seamless collaboration and the ability to review and reflect on code changes. By embracing agile practices such as regular retrospectives, Java development teams can iteratively improve their processes and deliver higher-quality software.
A Final Look
By embracing the principles of Kanban in Java development, teams can effectively visualize their workflow, limit work in progress, and continuously improve their processes. The result is increased productivity, better focus, and a streamlined development cycle.
In a competitive industry where time to market and quality are paramount, implementing Kanban principles in Java development can be a game-changer for teams striving to deliver exceptional software efficiently and effectively.
Incorporating Kanban principles into Java development is not just about organizing work—it's about fostering a culture of efficiency and continual improvement. With the right mindset and the tools tailored to support this approach, teams can maximize their productivity and deliver exceptional software with confidence.