Improving Code Quality Through Efficient Code Reviews
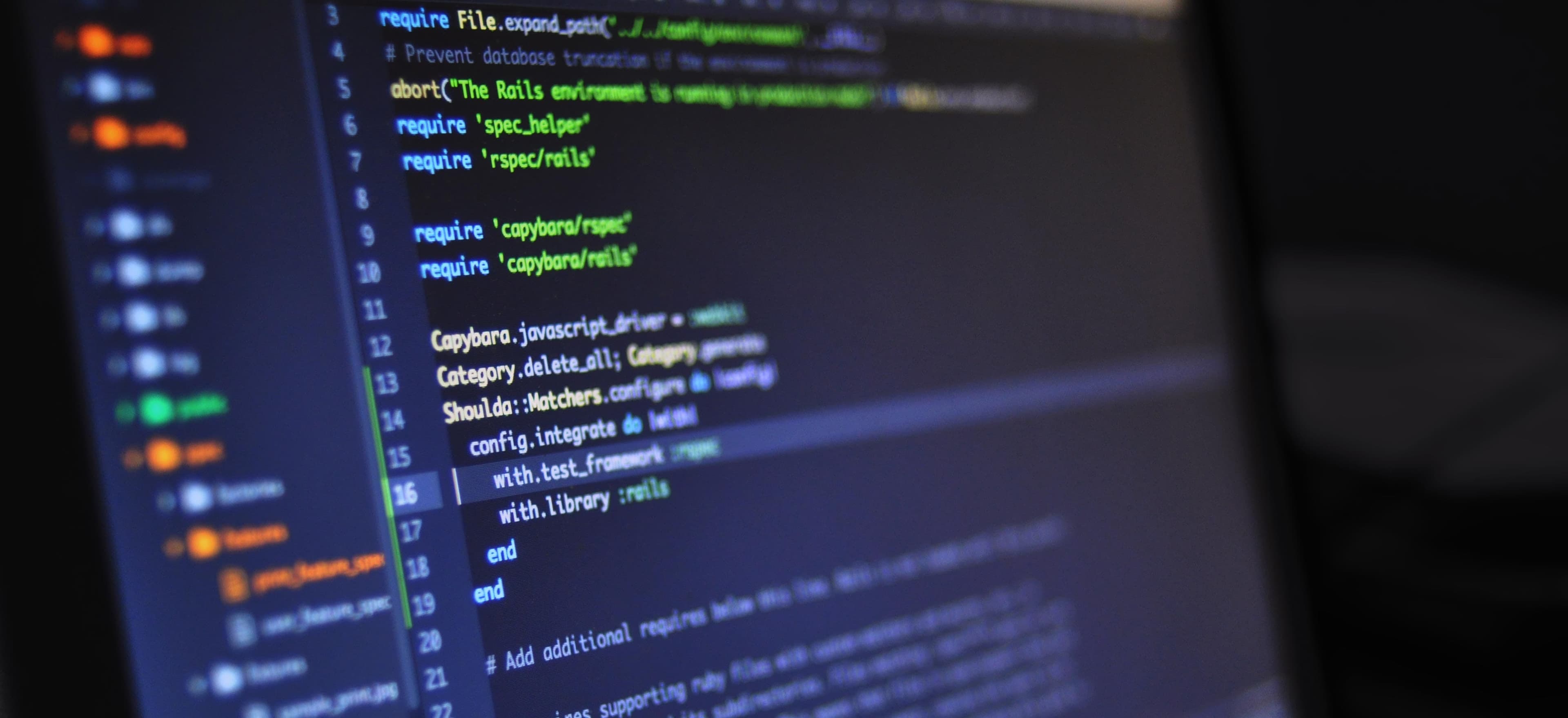
- Published on
Improving Code Quality Through Efficient Code Reviews
As a Java developer, one of the key processes for ensuring high-quality code is through efficient code reviews. Code reviews not only identify bugs and defects but also help in sharing knowledge, maintaining coding standards, and improving overall code quality.
In this article, we'll explore the importance of code reviews in the context of Java development and discuss techniques to conduct efficient code reviews that yield tangible benefits. We will also provide actionable tips for both reviewers and authors to make the most out of the code review process.
The Importance of Code Reviews in Java Development
Code reviews play a crucial role in the development lifecycle by providing an opportunity for developers to critique each other's work. They facilitate knowledge sharing and learning from peers, which is particularly important in Java, given its vast ecosystem and constantly evolving best practices.
In Java, code reviews are essential for ensuring adherence to language-specific guidelines, identifying potential memory leaks, discussing thread safety, and evaluating the proper use of Java APIs and libraries.
Techniques for Efficient Code Reviews
1. Setting Clear Objectives
Before diving into a code review, it's vital to establish clear objectives for the review process. This includes identifying the specific aspects of the code to be examined, such as functionality, performance, security, and adherence to best practices. Setting clear objectives helps reviewers focus on the most critical aspects of the code.
2. Utilizing Code Analysis Tools
Utilize static code analysis tools such as FindBugs, PMD, and Checkstyle to automate the detection of common code issues. Integrating these tools into the code review process allows for a more efficient and thorough analysis of the codebase. For example, using FindBugs for identifying potential bugs and Checkstyle for enforcing coding conventions can significantly improve the overall code quality.
3. Collaborative Review Process
Engage in a collaborative review process where both the author and the reviewer actively participate in discussions. As a reviewer, ask questions and provide constructive feedback, while as an author, be open to receiving input and explaining the reasoning behind design choices. This collaboration fosters a culture of continuous improvement and knowledge sharing within the development team.
4. Automated Testing Integration
Ensure that the code under review is accompanied by relevant automated tests. This not only validates the functionality of the code but also provides a safety net for future code modifications. By integrating automated testing into the code review process, potential regression issues can be caught early, contributing to the overall robustness of the codebase.
5. Establishing Coding Standards
Define and adhere to clear coding standards within the codebase. This includes formatting conventions, naming conventions, and best practices specific to Java development. By maintaining consistent coding standards, the codebase becomes more readable and maintainable, leading to improved overall code quality.
Best Practices for Reviewers
1. Understanding the Context
Before delving into the code, reviewers should strive to understand the broader context of the changes. This includes understanding the user stories, feature requirements, and any relevant design or architectural decisions. Contextual understanding enables more meaningful feedback and helps in identifying potential edge cases that the code should cater to.
2. Focus on High-Impact Areas
Instead of nitpicking minor style issues, focus on high-impact areas such as algorithmic complexity, error handling, potential resource leaks, and security vulnerabilities. By prioritizing high-impact areas, reviewers can maximize the value they provide and avoid getting caught up in trivial details that may not significantly affect the code's functionality or maintainability.
Example:
// Bad practice: Unnecessary complex logic
if (flag == true) {
return true;
} else {
return false;
}
// Good practice: Simplify the logic
return flag;
In the above example, replacing the condition with the boolean flag directly simplifies the logic and improves readability.
3. Provide Actionable Feedback
When providing feedback, ensure that it is actionable and specific. Vague comments like "This code is unclear" are less helpful than specific suggestions such as "Consider extracting this logic into a separate method for improved readability." Actionable feedback guides authors towards concrete improvements and fosters a culture of continuous learning and enhancement.
4. Balance Objectivity and Empathy
Maintain a balance between objectivity and empathy during code reviews. While it's crucial to objectively point out areas for improvement, it's equally important to acknowledge the author's efforts and provide feedback in a respectful manner. Effective code reviews focus on improving the code, not criticizing the author.
Best Practices for Authors
1. Self-Review Before Submission
Before initiating a code review, authors should conduct a self-review of their code. This includes reviewing the changes for adherence to coding standards, identifying potential code smells, and ensuring that the code aligns with the established design and architectural patterns. Self-reviewing allows authors to catch and address obvious issues before involving the reviewer.
2. Provide Contextual Information
When submitting code for review, authors should provide contextual information such as the purpose of the changes, any relevant design considerations, and any potential trade-offs made during implementation. Contextual information aids reviewers in understanding the rationale behind the code, leading to more insightful feedback.
3. Be Open to Feedback
Approach code reviews with an open mind and be receptive to feedback. Instead of being defensive about the code, use the feedback as an opportunity to learn and improve. Engage in constructive discussions with reviewers to gain a better understanding of their suggestions and incorporate valuable feedback into the codebase.
4. Continuous Learning and Improvement
Use code reviews as a learning opportunity to expand your knowledge and skillset. Actively seek feedback on your code, ask questions, and engage in discussions to understand alternative approaches and best practices. Embracing a mindset of continuous learning and improvement fosters personal and collective growth within the development team.
Closing Remarks
Efficient code reviews are instrumental in ensuring the overall quality and maintainability of Java codebases. By establishing clear objectives, leveraging code analysis tools, fostering a collaborative review process, and adhering to best practices for reviewers and authors, development teams can derive significant value from the code review process.
Remember, the goal of code reviews is not just to find defects but to enhance the collective knowledge and expertise of the team. Through effective code reviews, Java developers can elevate their coding standards, share insights, and collectively strive for excellence in software development.
Incorporating these techniques and best practices into your code review process can lead to more efficient reviews, higher-quality code, and a more cohesive and knowledgeable development team.
So, embrace code reviews as a valuable practice and make them an integral part of your Java development workflow for sustained code quality and continuous improvement.
Happy coding!
*References: