Struggling with Tess4J? Common OCR Errors and Fixes
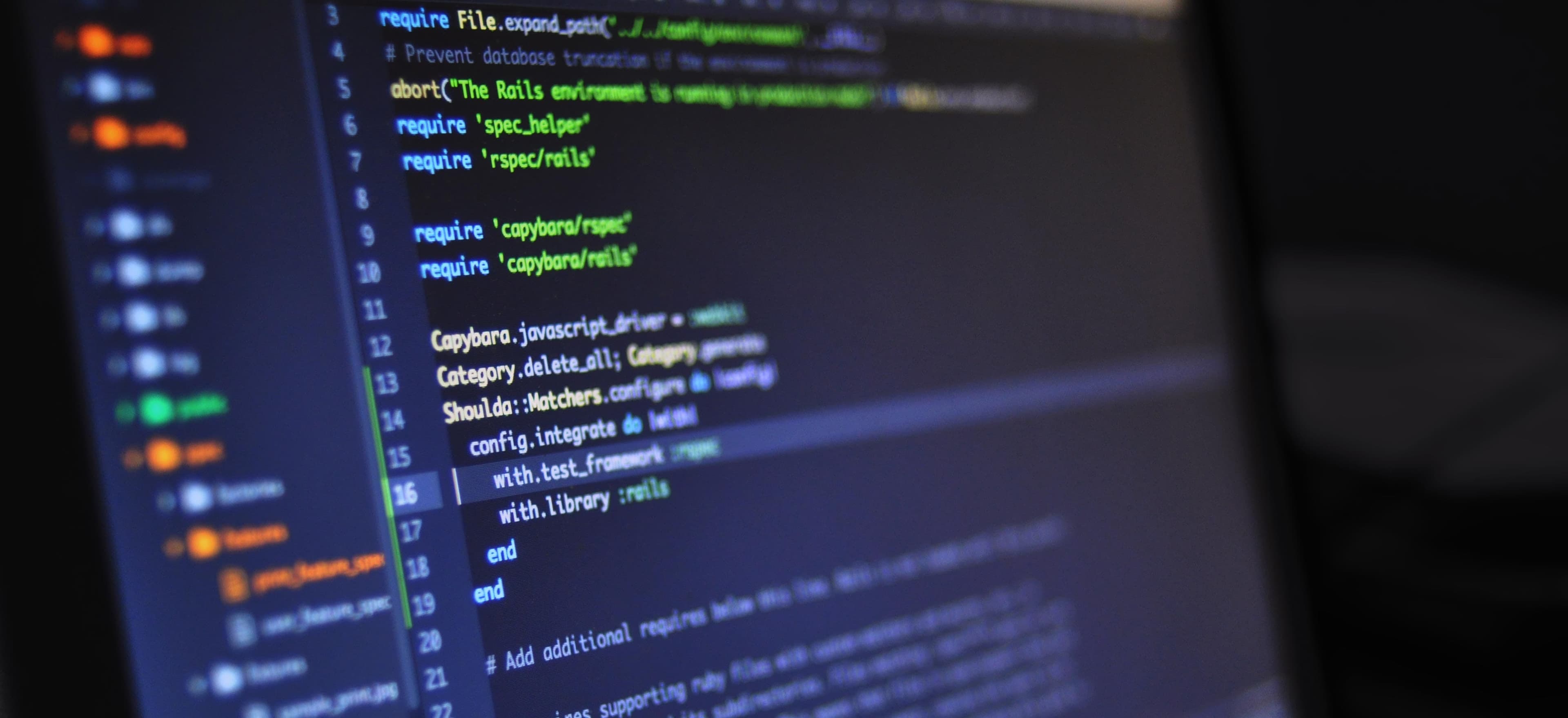
- Published on
Struggling with Tess4J? Common OCR Errors and Fixes
Optical Character Recognition (OCR) technology has significantly transformed how we process printed text, making it easier to digitize documents, automate data extraction, and facilitate numerous applications in software development. Among the various OCR libraries available, Tess4J stands out for Java developers seeking a wrapper around the powerful Tesseract OCR engine.
Despite its robustness, Tess4J isn't without its quirks. New users often encounter common issues, which can be frustrating. This blog post addresses common OCR errors you may face when using Tess4J and how to overcome them.
What is Tess4J?
Tess4J is a Java-based wrapper for Tesseract OCR, which provides an easy interface for accessing Tesseract's capabilities from Java applications. It enables Java applications to perform OCR on images and PDF files, converting complex visual information into editable text.
To kick things off and to follow along, ensure you have Java installed on your machine as well as Tess4J properly set up.
Setting Up Tess4J
Before discussing common issues, let's set up Tess4J. Here is how you would begin:
- Add Tess4J to Your Project:
If you are using Maven, you can add Tess4J by including the following dependency in your pom.xml
file:
<dependency>
<groupId>net.sourceforge.tess4j</groupId>
<artifactId>tess4j</artifactId>
<version>5.5.0</version>
</dependency>
- Download Tesseract:
You must also install Tesseract itself. You can find installation instructions for various operating systems on the Tesseract GitHub Repository.
- Set up your Project:
import net.sourceforge.tess4j.*;
import java.io.File;
public class OCRExample {
public static void main(String[] args) {
File imageFile = new File("path/to/image.png");
ITesseract tesseract = new Tesseract();
tesseract.setDatapath("path/to/tessdata");
try {
String result = tesseract.doOCR(imageFile);
System.out.println(result);
} catch (TesseractException e) {
System.err.println(e.getMessage());
}
}
}
In the above code:
- We define the file path to the image we want to process.
- We specify the location of Tesseract's language data files through
setDatapath
. - We use
doOCR
to perform the recognition, catching any exceptions that occur.
Common Errors and Fixes
1. Missing tessdata
Directory
Error Message: TesseractException: Unable to open file <filename>. Check your parameters.
The issue often stems from the Tesseract OCR engine not locating the tessdata
directory that contains language files.
Fix: Ensure you set the correct path for tessdata
. The path can be set using:
tesseract.setDatapath("C:/Program Files/Tesseract-OCR/tessdata");
Adjust this path according to where you have installed Tesseract on your system.
2. Language Files Not Found
Error Message: TesseractException: Can’t load language ‘eng’
This error indicates that the specified language file is missing from the tessdata
folder.
Fix: Double-check that the required language files (like eng.traineddata
) exist in your tessdata
directory. You can download additional language files from the Tesseract tessdata repository.
3. Poor OCR Performance
Error Message: No specific error, but the output text is inaccurate or garbled.
Several factors can contribute to poor performance in OCR tasks:
- Image Quality: Low-resolution images will yield unreliable results.
- Text Configuration: Complex fonts, colors, or backgrounds can confuse the engine.
Fix: Pre-process the image to enhance OCR results. You can use libraries such as Java AWT or OpenCV for image processing. Below is an example code snippet to improve image sharpness:
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public static BufferedImage sharpenImage(File imageFile) throws IOException {
BufferedImage img = ImageIO.read(imageFile);
// Create and initialize a kernel for sharpening
float[] sharpeningKernel = {
0, -1, 0,
-1, 5, -1,
0, -1, 0
};
Kernel kernel = new Kernel(3, 3, sharpeningKernel);
ConvolveOp convolveOp = new ConvolveOp(kernel);
return convolveOp.filter(img, null);
}
Utilizing image pre-processing can significantly improve the accuracy of the OCR process.
4. Memory Limitations
Error Message: TesseractException: Insufficient memory
Large images can consume substantial memory, leading to exceptions in recognizing the text.
Fix: Downsize the images before processing them or increase the Java VM's heap size:
java -Xmx2048m -jar yourapp.jar
5. Jar File Dependencies
Error Message: NoClassDefFoundError: org.bytedeco.javacv
Sometimes, required dependencies may not be included in your project.
Fix: Include all necessary dependencies in your pom.xml
. For example, you might need to add:
<dependency>
<groupId>org.bytedeco</groupId>
<artifactId>javacv-platform</artifactId>
<version>1.5.4</version> <!-- Use the latest version -->
</dependency>
Advanced OCR Configuration
Setting Configuration Parameters
Tesseract allows you to tweak configurations for better OCR results. Here’s how you can set parameters in your Java code:
tesseract.setPageSegMode(ITesseract.PageSegMode.PSM_AUTO);
tesseract.setOcrEngineMode(ITesseract.OEM.DEFAULT);
- PageSegMode (PSM): Adjusting this setting can improve recognition based on the layout of the text.
- OcrEngineMode (OEM): Choose which engine mode to use for more specialized tasks.
For a deeper dive into configuration parameters, check the Tesseract documentation.
The Last Word
Tess4J provides a powerful yet approachable interface for using the Tesseract OCR engine in your Java applications. Although users may encounter a variety of issues ranging from configuration errors to performance challenges, many of these problems have straightforward solutions.
By understanding the common pitfalls and employing careful image processing techniques, you can unlock the full capabilities of OCR technology. Continue experimenting and refining your use of Tess4J to discover how you can integrate OCR into your projects effectively.
For further reading, you may want to check out:
Engage with the community, explore new features, and continue to enhance your applications with OCR capabilities!