Handling Testing of Email Code in Spring Boot
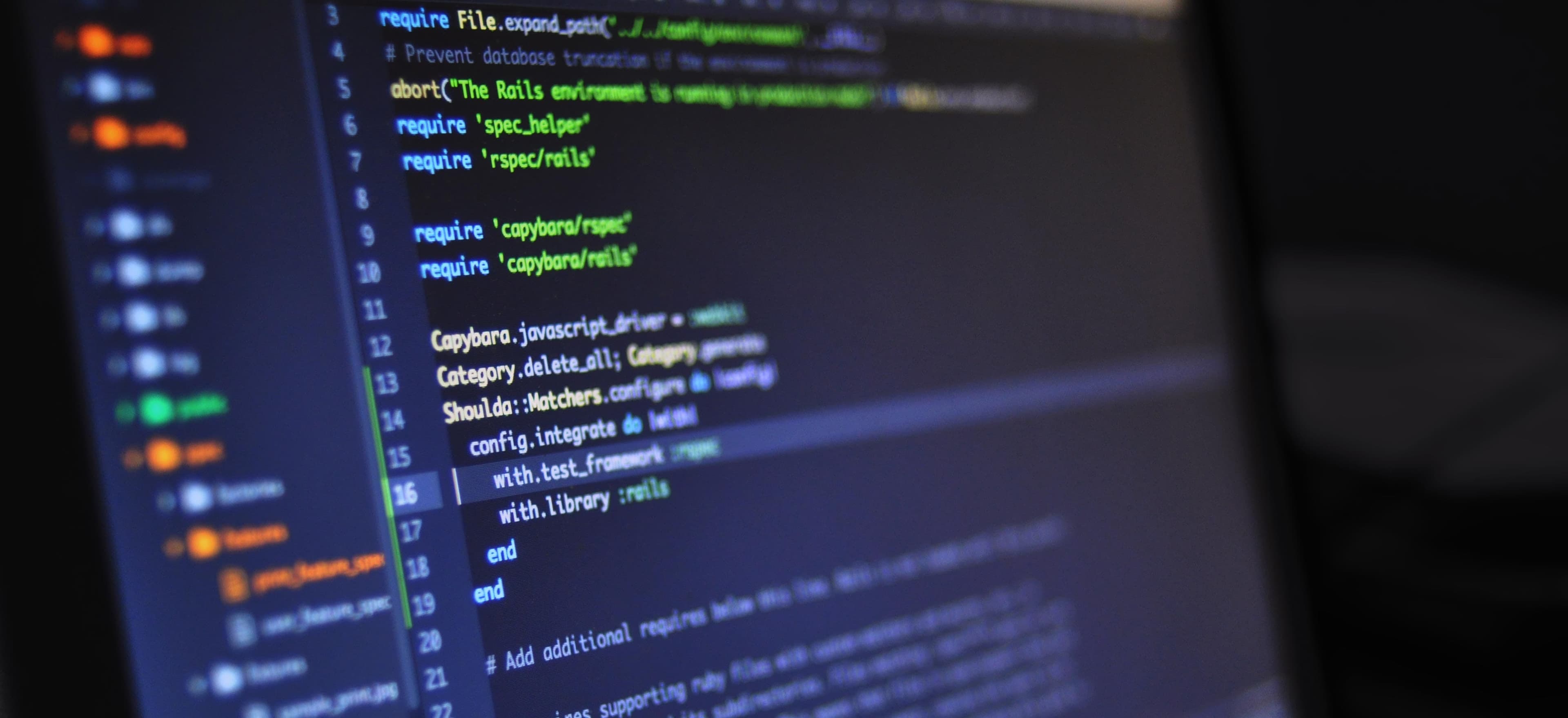
- Published on
Testing Email Functionality in Spring Boot
Testing email functionality is an essential part of ensuring the reliability of a Spring Boot application. In this blog post, we will discuss how to effectively test email functionality in a Spring Boot application. We will explore various strategies and best practices for testing email functionality, including unit testing and integration testing. By the end of this post, you will have a clear understanding of how to test email functionality in your Spring Boot application, ensuring that your email-related code is robust and reliable.
Setting Up Email Functionality in Spring Boot
Before diving into testing email functionality, let's first set up email functionality in a Spring Boot application. To send emails in a Spring Boot application, we typically use the JavaMailSender interface provided by Spring. Here's a simple example of configuring and sending an email using JavaMailSender:
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
public class EmailService {
private JavaMailSender javaMailSender;
public EmailService(JavaMailSender javaMailSender) {
this.javaMailSender = javaMailSender;
}
public void sendEmail(String to, String subject, String body) throws MessagingException {
MimeMessage message = javaMailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setTo(to);
helper.setSubject(subject);
helper.setText(body, true);
javaMailSender.send(message);
}
}
In the above code, we have a simple EmailService class that uses JavaMailSender to send an email. We inject an instance of JavaMailSender into the EmailService class and use it to create and send a MimeMessage with the help of MimeMessageHelper.
Unit Testing Email Functionality
Unit testing is the practice of testing individual units or components of a software application in isolation. When it comes to testing email functionality, we can write unit tests to verify the behavior of the EmailService class. We want to ensure that the sendEmail method functions as expected and sends an email with the correct content.
Let's write a unit test for the EmailService class using JUnit and Mockito. We will mock the JavaMailSender interface to avoid sending actual emails during the test.
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.Mockito;
import org.mockito.junit.jupiter.MockitoExtension;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
@ExtendWith(MockitoExtension.class)
public class EmailServiceTest {
@Mock
private JavaMailSender javaMailSender;
@InjectMocks
private EmailService emailService;
@Test
public void testSendEmail() throws MessagingException {
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, true);
Mockito.when(javaMailSender.createMimeMessage()).thenReturn(mimeMessage);
Mockito.when(new MimeMessageHelper(mimeMessage, true)).thenReturn(mimeMessageHelper);
String to = "recipient@example.com";
String subject = "Test Subject";
String body = "Test Body";
emailService.sendEmail(to, subject, body);
Mockito.verify(javaMailSender).send(mimeMessage);
}
}
In this unit test, we use Mockito to mock the JavaMailSender interface and verify that the send method is called with the correct MimeMessage. We also verify that the MimeMessageHelper is instantiated with the correct parameters.
Integration Testing Email Functionality
While unit tests are valuable for testing individual components in isolation, integration tests are necessary to validate the interactions between different components. When it comes to testing email functionality in a Spring Boot application, we can write integration tests to ensure that emails are sent successfully using a real mail server.
For integration testing of email functionality, we can utilize the Spring Boot Test framework and an embedded SMTP server such as GreenMail. The following example demonstrates how to write an integration test for the EmailService class using GreenMail as an embedded SMTP server.
import com.icegreen.greenmail.util.GreenMail;
import com.icegreen.greenmail.util.ServerSetup;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.mock.mockito.MockBean;
import org.springframework.mail.javamail.JavaMailSender;
import javax.mail.internet.MimeMessage;
@SpringBootTest
public class EmailServiceIntegrationTest {
@Autowired
private EmailService emailService;
@Autowired
private JavaMailSender javaMailSender;
private static GreenMail smtpServer;
@BeforeAll
public static void setupSmtpServer() {
ServerSetup serverSetup = new ServerSetup(2525, "localhost", "smtp");
smtpServer = new GreenMail(serverSetup);
smtpServer.start();
}
@AfterAll
public static void stopSmtpServer() {
smtpServer.stop();
}
@Test
public void testSendEmail() throws Exception {
String to = "recipient@example.com";
String subject = "Test Subject";
String body = "Test Body";
emailService.sendEmail(to, subject, body);
MimeMessage[] receivedMessages = smtpServer.getReceivedMessages();
// assert logic to verify the email is received
}
}
In this integration test, we use the @SpringBootTest annotation to load the application context, including the EmailService and JavaMailSender beans. We also start an embedded GreenMail SMTP server to capture the outgoing emails. After sending an email using the EmailService, we can verify the received emails using the GreenMail API.
Closing the Chapter
In this blog post, we covered the importance of testing email functionality in a Spring Boot application and explored different testing strategies. We learned how to write unit tests using Mockito to verify the behavior of the EmailService class. Additionally, we discussed how to write integration tests using an embedded SMTP server to validate the end-to-end email sending process.
Effective testing of email functionality is crucial for ensuring the reliability and robustness of a Spring Boot application. By following the best practices and strategies outlined in this post, you can confidently test the email-related code in your Spring Boot application, ultimately leading to a more stable and dependable email functionality.
Remember, thorough testing not only validates the correctness of your email code but also boosts the overall quality and resilience of your Spring Boot application.
For further exploration into testing Spring Boot applications, check out Spring Boot Testing - A Comprehensive Guide. Additionally, you can delve deeper into the nuances of email testing by referring to the official documentation on JavaMailSender.
Now, go forth and ensure the reliability of your email functionality through rigorous testing!