Optimizing Event Processing in JBoss BRMS Deployments
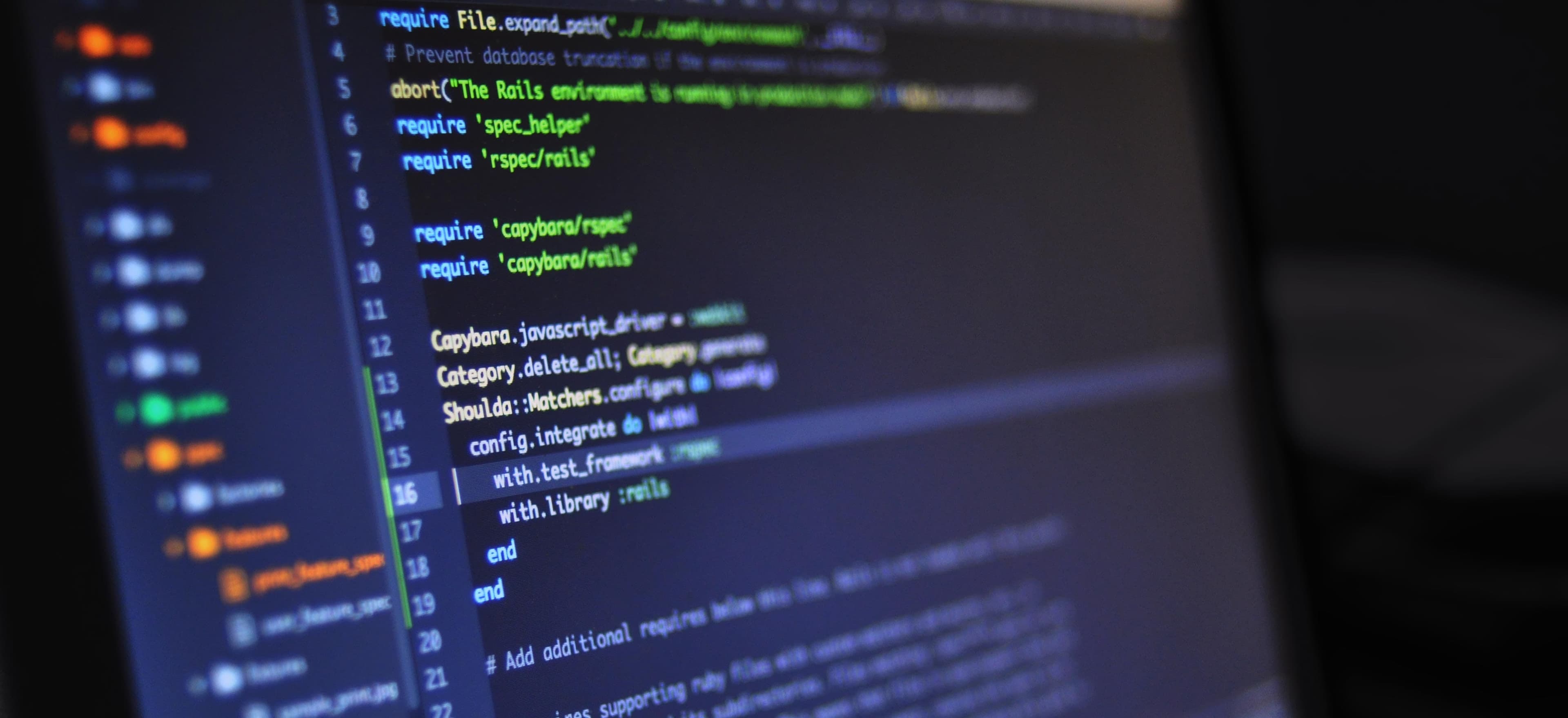
- Published on
Optimizing Event Processing in JBoss BRMS Deployments
In today's fast-paced software landscape, businesses are constantly looking for ways to make their applications more efficient and agile. In particular, rule-based systems can significantly benefit from optimized event processing. JBoss Business Rules Management System (BRMS) provides a potent framework for managing business rules, and ensuring that event processing is optimized is crucial for harnessing its full potential.
In this blog post, we will delve into strategies for optimizing event processing when working with JBoss BRMS. We will cover everything from configuring your environment correctly to implementing best practices in your code. Let's dive in!
Understanding JBoss BRMS and Event Processing
Before we can optimize event processing in JBoss BRMS, it's important to understand how it operates. JBoss BRMS is based on the Drools rule engine, which allows you to define complex business rules that can infer actions based on incoming events. The main goal is to create a responsive system that reacts intelligently to changes.
Event processing in JBoss BRMS is typically asynchronous. Events generated within a system are pushed to the rule engine, where they are evaluated against predefined rules. Efficient event processing can enhance application performance and responsiveness, making it critical to implement optimization strategies.
Key Factors Impacting Event Processing Efficiency
- Event Volume: High volumes of events can overwhelm the rule engine, causing delays in rule execution.
- Complexity of Rules: More complicated rules require more processing power.
- Resource Availability: The hardware and configuration of your server can impact performance.
- Rule Configuration: Improperly configured rules can lead to unnecessary checks and processing.
Setting Up Your Environment
Choosing the Right Hardware
Before you even start with code, ensure that your environment is robust enough to handle your anticipated load. A multi-core CPU and adequate memory will keep your event processing fast.
Configuration Tuning in JBoss
JBoss provides multiple configuration options for fine-tuning your BRMS deployment. Below are some key parameters you might want to consider adjusting:
- Drools Session Configuration: Configure session types and limits to optimize rule processing.
<rules>
<rule-session id="default" type="stateless">
<max-activations>1000</max-activations>
<job-executor>
<max-threads>10</max-threads>
</job-executor>
</rule-session>
</rules>
- Memory Management: Configure the JVM memory settings adequately to handle large volumes of events without running out of heap space.
JAVA_OPTS="-Xms512m -Xmx2048m -XX:MaxPermSize=256m"
Designing Efficient Business Rules
Creating efficient rules is critical for optimized event processing. Here are some best practices:
Keep Rules Simple
Complex rules increase processing time. Aim for clarity and maintainability.
rule "Simple age check"
when
$person: Person(age > 18)
then
System.out.println("Person is an adult: " + $person.getName());
end
The above rule is straightforward and processes quickly. When creating rules, think about splitting complex rules into simpler, smaller rules.
Use Rule Activation Groups
If you have related rules, consider using activation groups. This allows only one rule in the group to trigger, reducing unnecessary processing.
rule "Grant discount"
activation-group "discounts"
when
$customer: Customer(type == "VIP")
then
// Grant discount
end
rule "Notify about discounts"
activation-group "discounts"
when
$customer: Customer(type == "Regular")
then
// Notify customer
end
Minimize Rule Interdependencies
Overlapping rules can interact negatively. Reducing interdependencies allows for more efficient evaluation.
Managing Event Streams
Use Bulk Event Processing
Batch processing events can significantly reduce the overhead of context switching seen with individual event processing. This approach collects several events and processes them at once, leading to reduced CPU cycles and improved performance.
List<Event> eventList = new ArrayList<>();
eventList.add(new Event(...));
eventList.add(new Event(...));
// Add more events
kSession.insertAll(eventList);
kSession.fireAllRules();
Implement Throttling
In high-load scenarios, using throttling can help prevent system overloads. JBoss allows you to set limits for incoming events, ensuring your processing can keep pace without overwhelming resources.
public void processEvent(Event event) {
if (eventQueue.size() > MAX_QUEUE_SIZE) {
throw new EventQueueFullException();
}
eventQueue.add(event);
}
By establishing a maximum queue size, you can prevent resource exhaustion.
Asynchronous Processing
Leveraging asynchronous processing capabilities can significantly enhance performance. This involves integrating message queues such as Apache Kafka or ActiveMQ when dealing with a high volume of events.
Using Message Queues
Here's an example of how to publish events to a message queue, decoupling the event generation from processing.
public void publishEvent(Event event) {
// Assuming you have set up a connection to a message broker
producer.send(new ProducerRecord<>("events", event));
}
Consumers can then process these events at their own pace, leading to more efficient resource usage.
Monitoring and Optimization
Performance Monitoring Tools
Regular monitoring is essential to identify bottlenecks in event processing. Consider tools like Prometheus and Grafana for real-time monitoring of your JBoss applications, including memory usage and processing times.
Profiling Your Application
Java Profilers such as VisualVM or YourKit can help in identifying slow parts of your code and understanding memory usage patterns.
The Last Word
Optimizing event processing in JBoss BRMS involves a multi-faceted approach, focusing on environment setup, efficient rule design, and robust event management practices. By understanding the underlying principles and employing these best practices, you can significantly enhance the performance and responsiveness of your applications.
For more in-depth insights, check out the official JBoss BRMS documentation and the Drools documentation for additional tips and advanced configurations.
Optimizing event processing is not a one-time task; it requires constant evaluation, monitoring, and adjustment as workload demands change. Keep your rules simple, leverage asynchronous processing, and design a robust event management strategy to fully utilize the capabilities of JBoss BRMS.
Happy coding!