Maximizing API Security Efficiencies Without Hiring
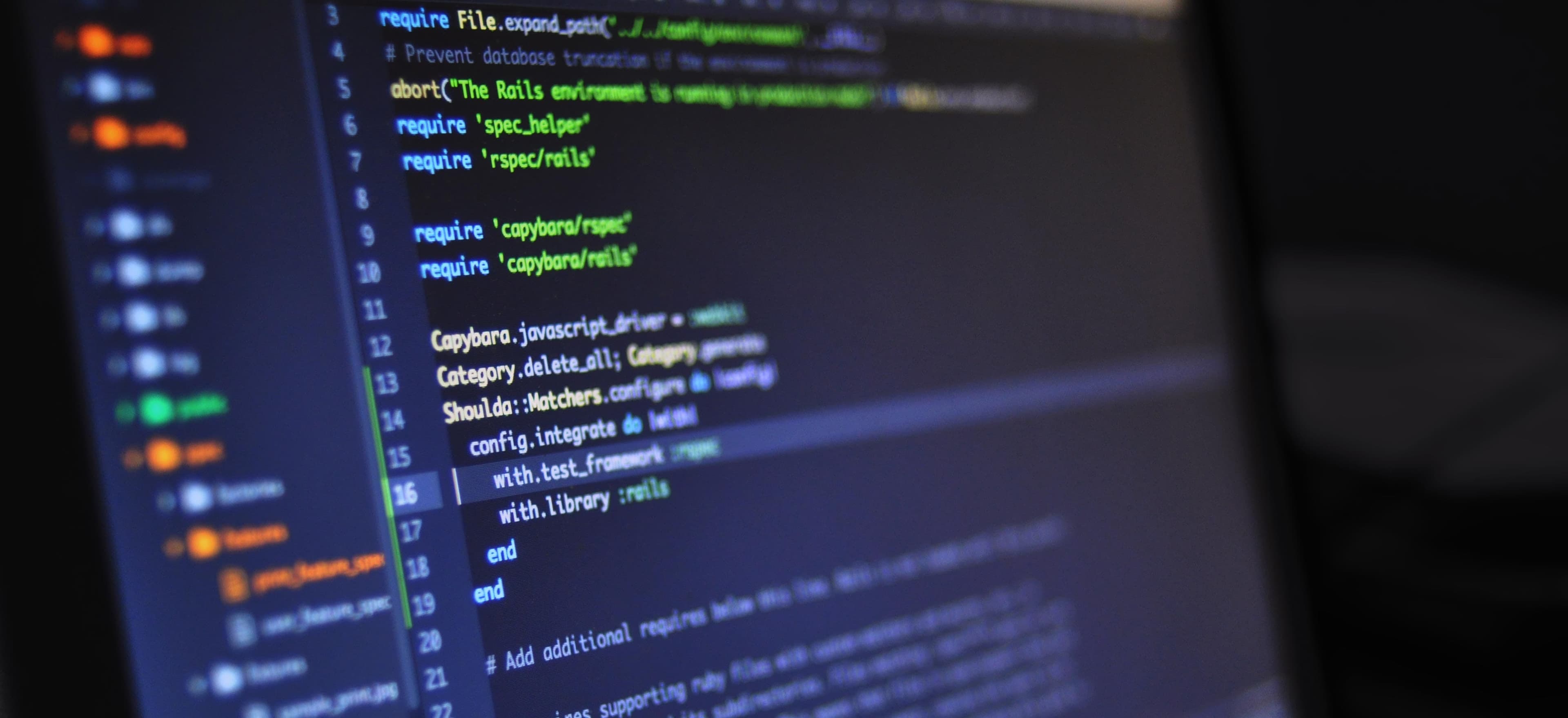
- Published on
In the fast-paced world of technology, APIs (Application Programming Interfaces) play a crucial role in enabling seamless communication and data exchange between disparate systems. As companies continue to leverage APIs for their applications, the need for robust API security has become more pressing than ever.
With the growing number of cyber threats and data breaches, it's essential for organizations to maximize API security efficiencies. This blog post will explore various strategies and best practices for enhancing API security in Java, without the need to hire additional resources.
Understanding the Importance of API Security
APIs act as a bridge between different software systems, allowing them to communicate and share data. However, this also makes them susceptible to security threats such as unauthorized access, data breaches, and manipulation of sensitive information. As a result, securing APIs has become a top priority for developers and organizations.
Implementing Robust Authentication
Authentication is the first line of defense in API security. By ensuring that only authorized users and systems have access to the API, the risk of unauthorized access and potential data breaches can be mitigated.
In Java, one of the most effective ways to implement authentication is by using JSON Web Tokens (JWT). JWT is a compact, URL-safe means of representing claims to be transferred between two parties. Leveraging libraries such as jjwt
makes it straightforward to handle JWT-based authentication within your Java API:
// Example JWT authentication using jjwt library
String token = Jwts.builder()
.setSubject("user123")
.setIssuedAt(new Date())
.signWith(SignatureAlgorithm.HS256, "secretKey")
.compact();
In this example, the JWT is signed with a secret key, ensuring that the token is tamper-proof. This straightforward approach provides a robust authentication mechanism for Java-based APIs.
Secure Communication with HTTPS
Secure communication between clients and servers is paramount in API security. Implementing HTTPS (HTTP over SSL/TLS) ensures that data transmitted between the client and server is encrypted, preventing unauthorized interception and tampering.
In Java, configuring HTTPS for your API can be achieved by setting up a secure web server using libraries such as Jetty
or Netty
. By generating a self-signed certificate or obtaining an SSL certificate from a trusted CA, you can enable HTTPS for your Java-based API.
// Setting up a secure web server with Jetty
Server server = new Server(8443);
ServerConnector connector = new ServerConnector(server);
connector.setPort(8443);
HttpConfiguration https = new HttpConfiguration();
https.addCustomizer(new SecureRequestCustomizer());
SslContextFactory.Server sslContextFactory = new SslContextFactory.Server();
sslContextFactory.setKeyStorePath("/path/to/keystore");
sslContextFactory.setKeyStorePassword("keystorePassword");
sslContextFactory.setKeyManagerPassword("keyManagerPassword");
SslConnectionFactory connectionFactory = new SslConnectionFactory(sslContextFactory, "http/1.1");
ServerConnector sslConnector = new ServerConnector(server,
new SslConnectionFactory(sslContextFactory, HttpVersion.HTTP_1_1.asString()),
new HttpConnectionFactory(https));
sslConnector.setPort(8443);
server.setConnectors(new Connector[] { connector, sslConnector });
server.start();
By incorporating HTTPS into your Java API, you can ensure that all data transmitted over the network is encrypted, bolstering the security of your API.
Input Validation and Data Sanitization
Another critical aspect of API security is input validation and data sanitization. Unvalidated input can lead to various security vulnerabilities, including SQL injection, cross-site scripting (XSS), and other forms of attacks.
In Java, libraries such as OWASP Java HTML Sanitizer
provide powerful tools to sanitize and validate input, especially when dealing with untrusted data. By integrating the OWASP Java HTML Sanitizer library, you can effectively sanitize input and mitigate the risk of XSS attacks:
// Sanitizing untrusted input using OWASP Java HTML Sanitizer
String userInput = "<script>alert('XSS')</script>";
PolicyFactory policy = new HtmlPolicyBuilder().toFactory();
String sanitizedInput = policy.sanitize(userInput);
By sanitizing user input before processing it within your API, you can significantly reduce the potential attack surface and enhance the overall security posture of your application.
Keeping APIs Updated and Patched
Regularly updating and patching your API dependencies and frameworks is crucial for addressing security vulnerabilities and staying ahead of potential exploits. By keeping your Java libraries, frameworks, and dependencies up to date, you can ensure that any reported security vulnerabilities are promptly addressed.
Tools like Maven
and Gradle
make it easy to manage dependencies in Java and stay informed about the latest security patches for your API's dependencies. By regularly checking for updates and integrating them into your build process, you can minimize the risk of using outdated and vulnerable components in your API.
Leveraging API Gateways for Enhanced Security
API gateways act as a centralized entry point for all incoming and outgoing API traffic. They provide features such as rate limiting, authentication, authorization, and traffic encryption, serving as a protective barrier for your APIs.
In the Java ecosystem, API gateway solutions like Apigee
and WSO2 API Manager
offer comprehensive security features, including OAuth integration, threat protection, and policy enforcement. By integrating your Java API with an API gateway, you can offload security concerns to a dedicated infrastructure, allowing for centralized management and enhanced security capabilities.
Bringing It All Together
In the evolving landscape of API security, taking proactive measures to fortify your Java API is essential for safeguarding sensitive data, preventing security breaches, and maintaining the trust of your users and partners. By implementing robust authentication, securing communication with HTTPS, validating and sanitizing input, keeping dependencies up to date, and leveraging API gateways, you can maximize API security efficiencies without the need to hire additional resources.
As you continue to enhance the security of your Java API, consider conducting regular security assessments, staying informed about emerging threats, and evolving your security practices to stay ahead of potential risks. By prioritizing API security and adopting a proactive mindset, you can build and maintain secure, resilient, and reliable APIs that align with the highest standards of security and compliance.
API security is not only a technical imperative but also a business imperative, and by employing the best practices outlined in this post, you can fortify your Java APIs against a myriad of security threats, fostering trust and confidence among your users and stakeholders.
Start fortifying your API security today and stay ahead in the ever-changing landscape of cybersecurity. Remember, the best defense is a proactive one, and the security of your Java API is well worth the investment of time and resources.
This blog post discussed strategies and best practices for enhancing API security in Java without the need to hire additional resources. By implementing robust authentication, securing communication with HTTPS, input validation, and leveraging API gateways, you can fortify your API security efficiently . Regularly updating dependencies, staying informed about security patches, and conducting security assessments are crucial for maintaining the security of your Java API.