Optimizing CRUD Operations with jOOQ and Spring
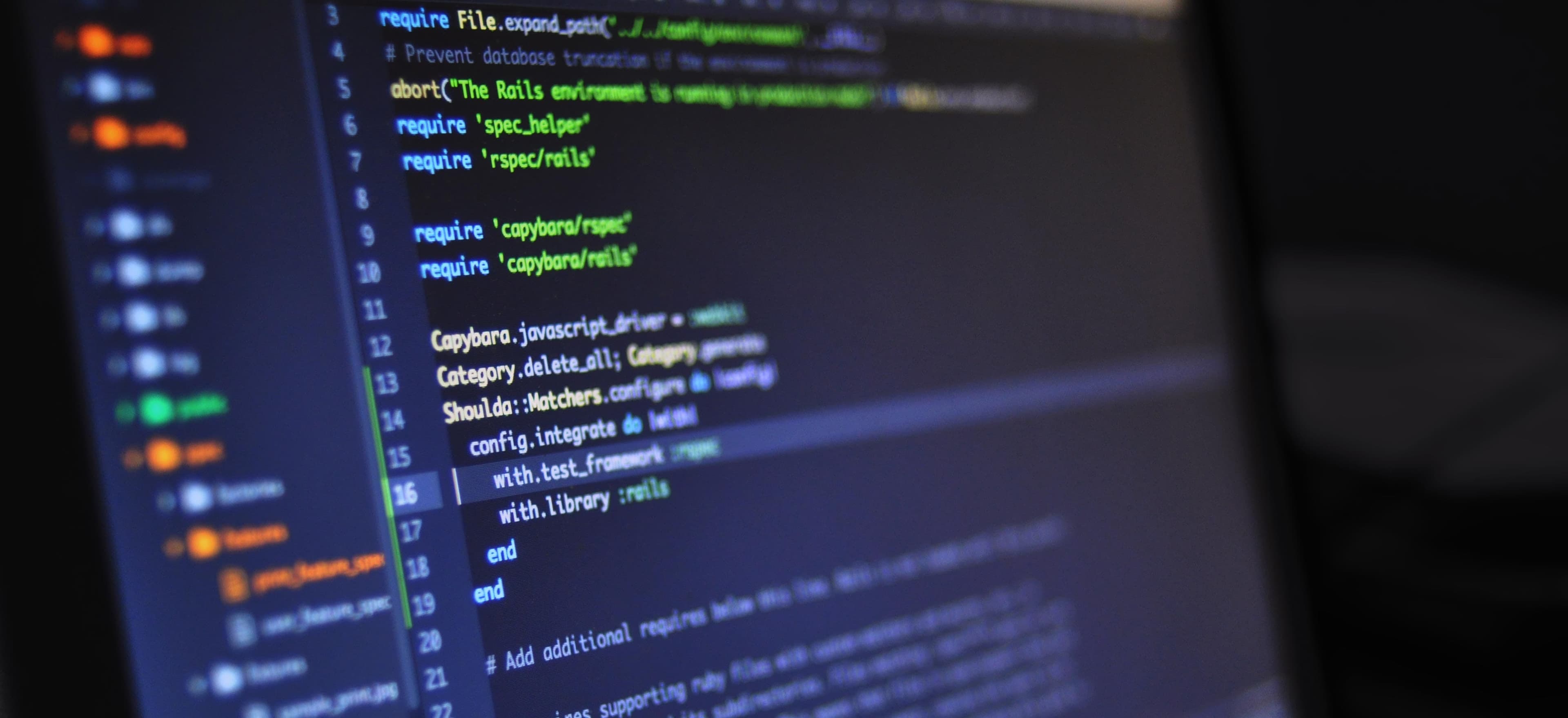
- Published on
Optimizing CRUD Operations with jOOQ and Spring
In the world of enterprise-level applications, optimizing the performance of CRUD (Create, Read, Update, Delete) operations is crucial for ensuring scalable and efficient data access. This blog post will explore how to optimize CRUD operations using the jOOQ library in conjunction with the Spring framework. By the end of this post, you will have a solid understanding of how to leverage jOOQ to streamline database access and improve the efficiency of your application's data operations.
What is jOOQ?
jOOQ is a Java library that provides a fluent API for building type-safe SQL queries. It allows developers to write database queries in a more natural and type-safe way, leveraging the power of Java's static typing and compiler checks. jOOQ supports a wide range of databases, including popular ones like MySQL, PostgreSQL, and Oracle.
Setting Up jOOQ with Spring
To begin using jOOQ with Spring, you'll first need to set up your project to include the jOOQ library and its required dependencies. You can add jOOQ to your project using Maven or Gradle, depending on your build tool of choice.
Maven Dependency
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq</artifactId>
<version>{latest_version}</version>
</dependency>
Gradle Dependency
implementation 'org.jooq:jooq:{latest_version}'
Additionally, you'll need to configure a jOOQ code generator to generate Java classes from your database schema. This can be achieved using the jOOQ code generation tool. Once configured, the tool will generate classes representing your database tables, records, and other database objects.
Optimizing CRUD Operations with jOOQ
1. Writing Type-Safe Queries
One of the primary advantages of using jOOQ is its support for writing type-safe SQL queries. This means that you can leverage the compile-time checks provided by Java to ensure that your queries are syntactically correct and refer to valid database objects. Let's take a look at an example of how jOOQ enables type-safe querying:
// Using jOOQ to select records from the "books" table
Result<Record> result = create.select()
.from(BOOKS)
.where(BOOKS.PUBLISHED_IN.eq(2021))
.fetch();
In this example, BOOKS
is a generated class representing the "books" table in the database. We are using the eq
method to create a type-safe condition that checks if the PUBLISHED_IN
column is equal to 2021. Notice how the IDE can provide auto-completion and type-checking for database objects and queries, making the code more reliable and less error-prone.
2. Batch Operations
jOOQ provides built-in support for batch operations, allowing you to execute multiple CRUD operations in a single database round-trip. This can significantly improve the performance of bulk data operations. Let's see how jOOQ enables batch operations:
// Using jOOQ to insert multiple records into the "books" table in a single batch
create.batch(
create.insertInto(BOOKS, BOOKS.TITLE, BOOKS.AUTHOR, BOOKS.PUBLISHED_IN)
.values("Clean Code", "Robert C. Martin", 2008),
create.insertInto(BOOKS, BOOKS.TITLE, BOOKS.AUTHOR, BOOKS.PUBLISHED_IN)
.values("Design Patterns", "Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides", 1994)
).execute();
In this example, we are using the batch
method to execute multiple insert operations in a single batch, minimizing the overhead of individual database round-trips.
3. Using Query DSL for Dynamic Queries
jOOQ's Query DSL (Domain-Specific Language) provides a powerful and expressive way to build dynamic queries. This is especially useful for constructing queries based on varying criteria at runtime. Let's take a look at how jOOQ's Query DSL facilitates the construction of dynamic queries:
// Using jOOQ's Query DSL to build a dynamic query with conditional filtering
SelectQuery<Record> dynamicQuery = create.selectQuery();
dynamicQuery.addSelect().from(BOOKS);
if (searchCriteria.getAuthor() != null) {
dynamicQuery.addConditions(BOOKS.AUTHOR.eq(searchCriteria.getAuthor()));
}
if (searchCriteria.getPublishedAfter() != null) {
dynamicQuery.addConditions(BOOKS.PUBLISHED_IN.greaterOrEqual(searchCriteria.getPublishedAfter()));
}
Result<Record> result = dynamicQuery.fetch();
In this example, we are dynamically adding conditions to the query based on the search criteria provided at runtime. This illustrates how jOOQ's Query DSL allows for the construction of flexible and dynamic queries without sacrificing type safety.
4. Fetching Strategies for Performance Optimization
jOOQ provides fetching strategies that allow you to fine-tune the behavior of fetching records from the database. By selecting the appropriate fetching strategy, you can optimize the performance of data retrieval operations. Let's consider an example of employing fetching strategies:
// Using jOOQ's fetching strategy to optimize the fetching behavior
List<Book> books = create.selectFrom(BOOKS)
.where(BOOKS.PUBLISHED_IN.eq(2021))
.fetchInto(Book.class);
In this example, we are using the fetchInto
method with a custom POJO class to fetch records from the database. jOOQ allows you to control the fetching behavior, such as whether to fetch data eagerly or lazily, to minimize the impact on performance.
Closing the Chapter
In this blog post, we've explored how jOOQ can be leveraged to optimize CRUD operations within a Spring application. By harnessing jOOQ's type-safe querying, batch operations, Query DSL, and fetching strategies, you can enhance the performance and efficiency of your application's data access layer. Employing these techniques will not only result in improved database interactions but also contribute to the overall scalability and responsiveness of your application.
To delve deeper into jOOQ and Spring integration, I recommend checking out the official jOOQ documentation and Spring Framework reference guide. These comprehensive resources provide valuable insights into maximizing the potential of jOOQ within the Spring ecosystem. Happy coding!