Managing Complex Method Parameters in Software Development
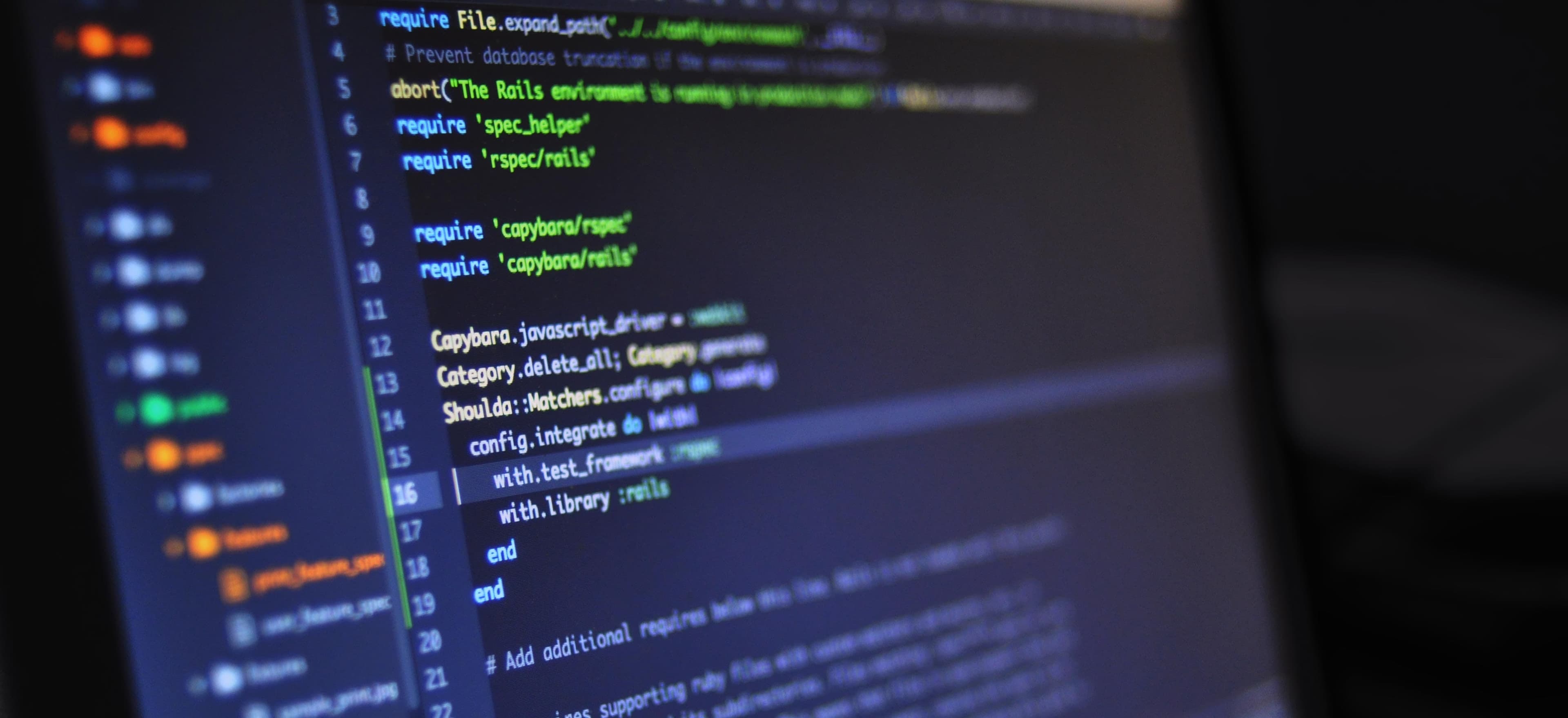
- Published on
Managing Complex Method Parameters in Software Development
When working on software development projects, it is common to encounter methods with complex parameter lists. Managing these complex method parameters effectively is crucial for writing maintainable, readable, and efficient code. In this article, we will explore best practices for handling complex method parameters in Java, including how to simplify parameter lists, improve readability, and enhance maintainability.
1. Use Objects to Group Related Parameters
When a method has a long list of parameters, it can be challenging to remember the order and purpose of each parameter. To address this issue, consider using objects to group related parameters. This approach not only simplifies the method signature but also improves readability and maintainability.
public class Order {
private Customer customer;
private List<Product> products;
private boolean isExpressDelivery;
// Constructor, getters, and setters
}
public void placeOrder(Order order) {
// Method implementation
}
In the above example, we have created an Order
class to encapsulate related parameters such as customer
, products
, and isExpressDelivery
. By passing an Order
object to the placeOrder
method, we significantly reduce the number of parameters, leading to cleaner and more manageable code.
2. Consider Builder Pattern for Complex Object Creation
When dealing with complex objects that require a large number of parameters for construction, the builder pattern can be an effective solution. The builder pattern separates the construction of an object from its representation, allowing for fluent and readable code when creating complex objects.
public class OrderBuilder {
private Customer customer;
private List<Product> products;
private boolean isExpressDelivery;
public OrderBuilder withCustomer(Customer customer) {
this.customer = customer;
return this;
}
public OrderBuilder withProducts(List<Product> products) {
this.products = products;
return this;
}
public OrderBuilder withExpressDelivery(boolean isExpressDelivery) {
this.isExpressDelivery = isExpressDelivery;
return this;
}
public Order build() {
return new Order(this);
}
}
// Usage
Order order = new OrderBuilder()
.withCustomer(customer)
.withProducts(products)
.withExpressDelivery(true)
.build();
In this example, the OrderBuilder
class provides fluent methods for setting the different parameters of an Order
object. This approach simplifies the process of object creation and eliminates the need for constructors with numerous parameters.
3. Leverage Enums for Limited Choices
When a method requires parameters with a limited set of choices, using enums can enhance the readability and type safety of the code. Enums provide a way to define a set of constants, making the code more expressive and self-documenting.
public enum UserRole {
ADMIN,
USER,
GUEST
}
public void updateUserRole(User user, UserRole role) {
// Method implementation
}
By using the UserRole
enum in the updateUserRole
method, we ensure that only valid role choices can be passed as parameters, reducing the risk of runtime errors and making the code more predictable.
4. Use Default Parameters and Method Overloading
In Java, default parameters are not natively supported, but method overloading can be used to achieve similar behavior. When a method has multiple parameters with sensible default values, consider overloading the method to provide versions with fewer parameters, setting default values for those omitted.
public void sendMessage(String message) {
// Method implementation
}
public void sendMessage(String message, String recipient) {
// Method implementation
}
public void sendMessage(String message, String recipient, boolean isUrgent) {
// Method implementation
}
By overloading the sendMessage
method with different parameter combinations, we allow callers to use the method with varying levels of complexity, while still providing sensible defaults for omitted parameters.
5. Document Method Parameters Clearly
Regardless of the approach used to handle complex method parameters, clear and concise documentation is essential. When a method expects complex parameters or a combination of parameters, thorough documentation helps developers understand the purpose of each parameter and its expected behavior.
/**
* Processes the order and updates inventory.
*
* @param order the order to be processed
*/
public void processOrder(Order order) {
// Method implementation
}
By providing descriptive Javadoc comments for method parameters, developers can easily comprehend the intended use of the parameters, leading to more maintainable and error-free code.
A Final Look
In software development, managing complex method parameters is crucial for writing clean, maintainable code. By leveraging objects, the builder pattern, enums, method overloading, and clear documentation, developers can simplify parameter lists, improve readability, and enhance the maintainability of their code. These best practices not only make code easier to understand and maintain but also contribute to a more efficient and enjoyable development experience.
By applying these principles, developers can streamline the development process and build robust, scalable software systems.
In conclusion, handling complex method parameters effectively contributes to the overall quality and sustainability of the software. Using objects to group related parameters, employing the builder pattern for complex object creation, leveraging enums for limited choices, utilizing default parameters and method overloading, and documenting method parameters clearly are all valuable strategies for managing complex method parameters in Java.
References:
Now armed with these best practices, you can enhance the readability, maintainability, and efficiency of your code when dealing with complex method parameters in your Java projects.