Scaling Stream Processing with Apache Apex
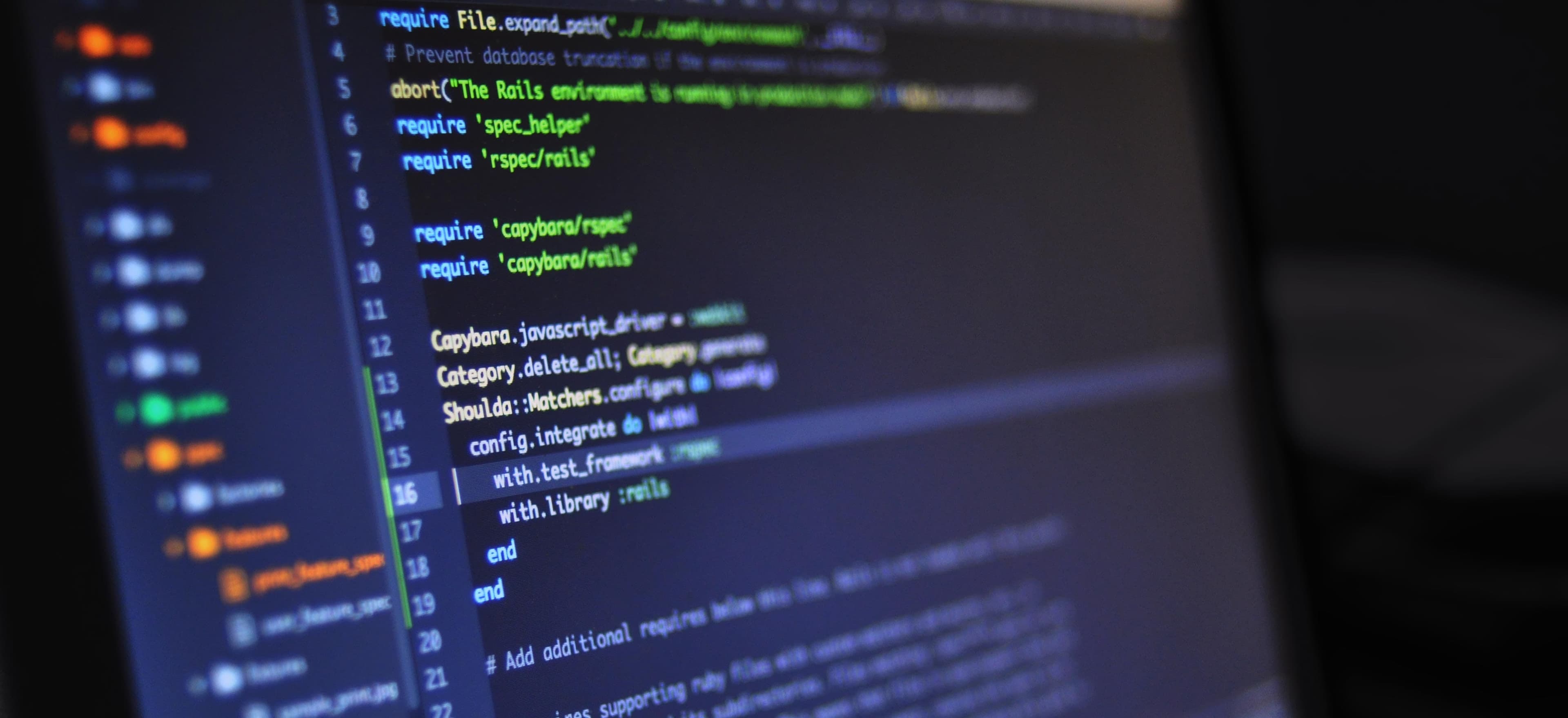
- Published on
Introduction
Stream processing has become an integral part of modern data architecture, allowing organizations to analyze real-time data and make decisions based on up-to-the-minute insights. Apache Apex is a powerful and scalable stream processing engine that provides high-performance, fault-tolerant, and exactly-once processing semantics. In this article, we'll explore the key aspects of Apache Apex, its scalability features, and how it enables organizations to handle large-scale stream data processing.
Understanding Apache Apex
Apache Apex is an open-source platform for building and deploying big data stream and batch processing applications. It is designed to process large volumes of data with low latency and high throughput, making it suitable for a wide range of use cases, including real-time analytics, fraud detection, monitoring, and more.
Scalability in Apache Apex
Scalability is a crucial factor in stream processing, especially when dealing with massive volumes of data. Apache Apex offers scalable processing capabilities through its parallelism model and support for distributed computing.
Parallelism Model
Apache Apex allows for fine-grained control over the parallelism of operators within an application. This means that operators can be scaled independently based on the specific processing requirements, such as the volume and complexity of data being processed. By distributing the workload across multiple operators and parallel instances, Apache Apex can efficiently utilize resources and scale to handle high data throughput.
Data Partitioning
In addition to operator parallelism, Apache Apex provides support for data partitioning, allowing the distribution of data across multiple processing nodes. This enables efficient data locality and parallel processing, resulting in improved performance and scalability.
Fault Tolerance
Scalability is meaningless without fault tolerance. Apache Apex is designed to handle failures gracefully, ensuring that processing can continue without data loss. It achieves fault tolerance through mechanisms such as checkpointing and state management, which allow applications to recover from failures and resume processing seamlessly.
Building Scalable Applications with Apache Apex
Now, let's dive into a practical example to demonstrate how Apache Apex enables the development of scalable stream processing applications.
Example: Word Count Application
Let's consider a simple word count application designed to process a high volume of text data in real time. The goal is to count the occurrences of each word within the incoming stream of text data.
public static class Tokenizer implements FlatMapFunction<String, String> {
@Override
public void flatMap(String input, Collector<String> collector) {
String[] tokens = input.split("\\s+");
for (String token : tokens) {
collector.collect(token);
}
}
}
public static class WordCount implements WindowedOperator {
private Map<String, Integer> wordCounts = new HashMap<>();
@Override
public void processWindow(List<String> tuples) {
for (String word : tuples) {
wordCounts.put(word, wordCounts.getOrDefault(word, 0) + 1);
}
// Emit word counts to the output stream
// ...
}
}
In this example, we have two operators - Tokenizer
and WordCount
. The Tokenizer
operator splits the incoming text data into individual words, while the WordCount
operator calculates the word counts within a window of time.
By leveraging Apache Apex's parallelism model, we can scale the Tokenizer
and WordCount
operators based on the volume of incoming data and the processing requirements. Additionally, data partitioning can be applied to distribute the input data across multiple processing nodes, allowing for efficient parallel processing and improved scalability.
Integrating with Apache Apex Ecosystem
Apache Apex can be seamlessly integrated with other components of the big data ecosystem, such as Apache Hadoop, Apache Kafka, and Apache NiFi, allowing organizations to build end-to-end data processing pipelines that are highly scalable and performant.
Use Case: Real-Time Fraud Detection
In a real-time fraud detection system, Apache Apex can ingest streaming transaction data from sources such as Apache Kafka, perform complex event processing and analysis using its scalable processing capabilities, and then integrate with a fraud detection system to make instantaneous decisions.
In Conclusion, Here is What Matters
Apache Apex provides a robust and scalable platform for building and deploying stream processing applications. Its support for fine-grained parallelism, data partitioning, fault tolerance, and seamless integration with other big data components make it a top choice for organizations looking to tackle large-scale data processing challenges.
By harnessing the power of Apache Apex, organizations can unleash the potential of real-time data analysis, enabling them to make critical business decisions based on the freshest insights.
In conclusion, Apache Apex is well-positioned to meet the increasing demand for scalable stream processing in today's data-driven world, making it a valuable tool for organizations seeking to leverage the full potential of real-time data processing.
For more information on Apache Apex and stream processing, check out the official Apache Apex documentation and this in-depth article on stream processing.