Asynchronous Data Loading in Grails: Best Practices
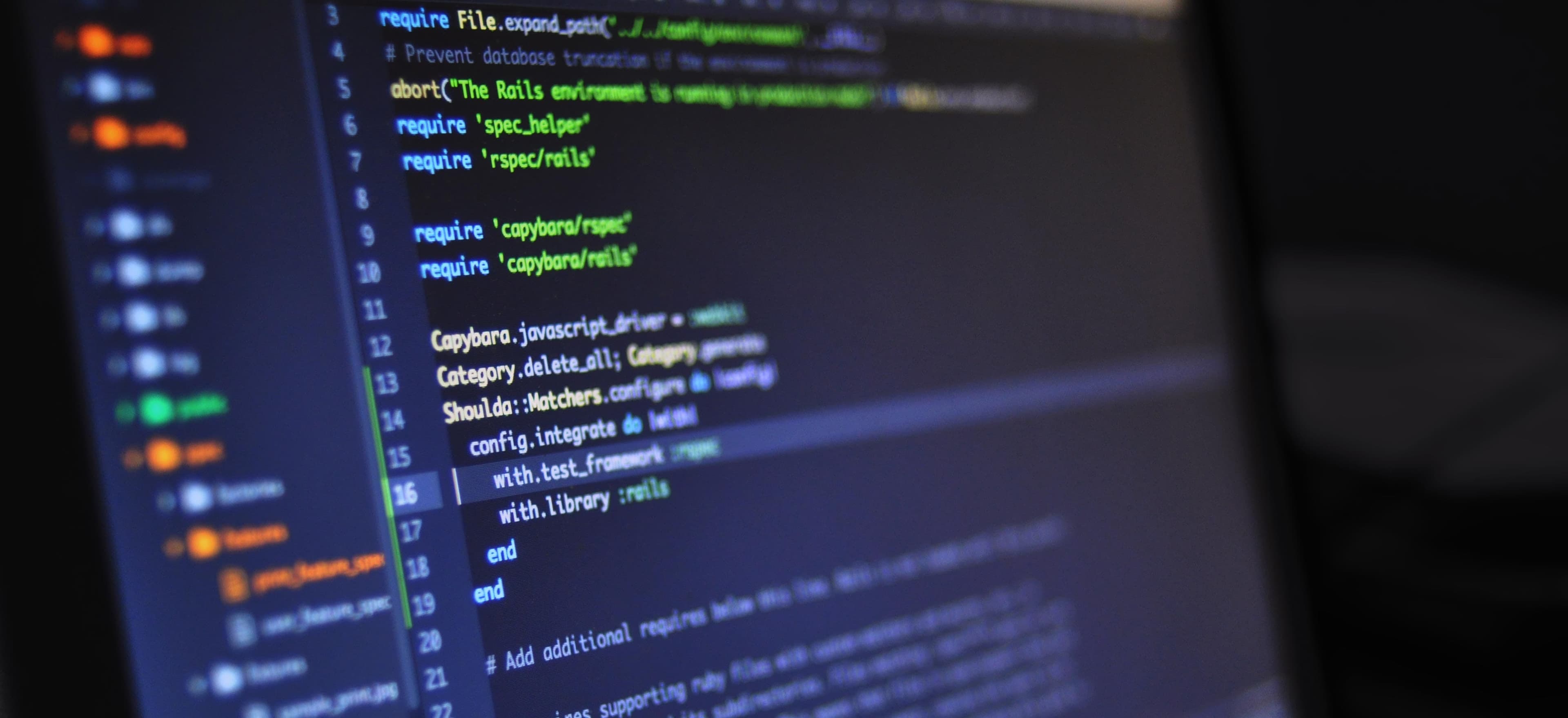
- Published on
Asynchronous Data Loading in Grails: Best Practices
In any web application, the loading and processing of data is an essential yet often time-consuming task. By default, Grails applications handle data loading synchronously, i.e., one task at a time in a sequential order. However, as an application scales or demands grow, synchronous data loading can lead to increased response times and decreased user experience. To alleviate this, Grails offers asynchronous data loading as a means to improve the performance and scalability of the application.
What is Asynchronous Data Loading?
Asynchronous data loading involves executing the data loading process independently of the main application thread, allowing the application to continue servicing other requests while the data is being loaded. This decoupling enables better performance as the application can handle more concurrent requests without being blocked by data loading tasks.
Why Use Asynchronous Data Loading in Grails?
- Improved Performance: Asynchronous loading prevents the main application thread from getting blocked, leading to faster response times and better user experience.
- Scalability: By being able to handle more concurrent requests, asynchronous loading contributes to the overall scalability of the application.
- Resource Utilization: It allows efficient utilization of system resources by allowing the application to perform other tasks while waiting for data to load.
Implementing Asynchronous Data Loading in Grails
Grails provides multiple ways to implement asynchronous data loading, leveraging features such as Promises and @Async annotations for methods.
Using Promises
import grails.async.Promise
Promise<List> loadDataAsync() {
return Promise.start {
// Perform asynchronous data loading here
// e.g., making a remote API call or querying a database
}
}
In this example, the Promise
class is used to wrap the asynchronous data loading process, allowing the main application thread to continue its operations while the data is being loaded in the background.
Using @Async Annotations
import org.springframework.scheduling.annotation.Async
@Async
void loadDataAsync() {
// Perform asynchronous data loading here
// e.g., making a remote API call or querying a database
}
By annotating a method with @Async
, Grails delegates the execution of the method to a separate thread, enabling asynchronous data loading without blocking the main application thread.
Best Practices for Asynchronous Data Loading in Grails
- Identify and Prioritize: Determine which parts of the application can benefit most from asynchronous loading and prioritize those areas.
- Handling Failures: Implement robust error handling and graceful degradation to handle failures in asynchronous data loading processes.
- Monitoring and Metrics: Utilize monitoring tools to track the performance and behavior of asynchronous data loading, enabling proactive maintenance and optimization.
Final Thoughts
Asynchronous data loading is a crucial technique for improving the performance and scalability of Grails applications. By leveraging asynchronous loading features, such as Promises and @Async annotations, developers can ensure that data loading processes do not hinder the responsiveness of the application. By following best practices and embracing asynchronous data loading, Grails applications can better handle increasing workloads while maintaining optimal performance.
For further information on asynchronous data loading in Grails, refer to the official Grails documentation.
Remember, optimizing data loading is just one aspect of building a high-performing web application, and there are various other optimization techniques to explore for crafting a robust and efficient system.