Synchronizing Different Methods on Same Object
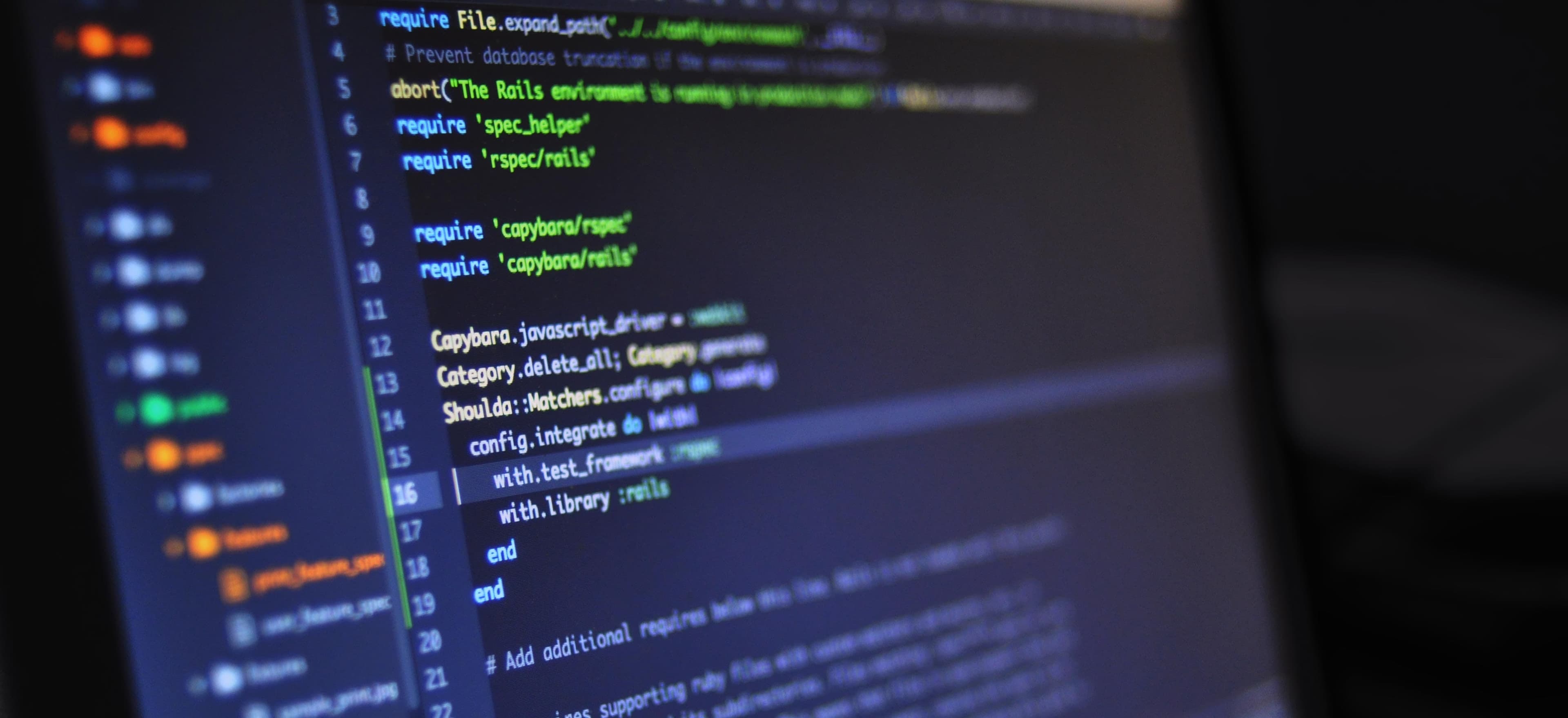
- Published on
In Java, synchronization is a process of controlling the access of multiple threads to shared resources. It plays a critical role in preventing data inconsistency that arises when multiple threads modify the same object concurrently. Synchronization ensures that only one thread can access the shared resource at a time. However, when different methods within the same object need to be synchronized, a crucial consideration is that the synchronized
keyword locks the entire object, not just a specific method. Let's delve into how to synchronize different methods on the same object in Java.
Why Synchronize Different Methods on the Same Object?
Synchronization is indispensable in multithreaded programming to maintain data consistency and avoid conflicts when multiple threads access shared resources. However, in some scenarios, it becomes necessary to synchronize only specific methods and not the entire object. For instance, consider a class with multiple methods and some of them modify shared data while others do not. Synchronizing the entire object when it is not imperative can lead to performance degradation. Therefore, it is essential to synchronize only the necessary methods to ensure thread safety without causing unnecessary contention.
Synchronizing Methods in Java
In Java, two ways can be employed to synchronize different methods of the same object - using the synchronized
keyword and using explicit locks provided by java.util.concurrent.locks.Lock
.
Using the synchronized
Keyword
The synchronized
keyword can be used to synchronize methods and code blocks. When a method is declared as synchronized
, Java ensures that only one thread can execute it at a time for a particular object. Here’s an example illustrating the use of the synchronized
keyword to synchronize different methods of the same object.
public class SynchronizedMethodsExample {
private int count = 0;
public synchronized void increment() {
count++;
}
public void commonMethod() {
// Non-synchronized method
}
public synchronized void decrement() {
count--;
}
}
In the above example, the increment()
and decrement()
methods are synchronized, ensuring that only one thread can execute either of them at a time. However, the commonMethod()
remains unsynchronized, allowing concurrent access by multiple threads.
Using ReentrantLock
Another approach to synchronize different methods on the same object is by using java.util.concurrent.locks.ReentrantLock
. Unlike the synchronized
keyword, ReentrantLock
provides more flexibility and features like the ability to create fair locks, try locking, and lock interruption. Here’s an example demonstrating the use of ReentrantLock
to synchronize different methods.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class SynchronizedMethodsExample {
private int count = 0;
private Lock lock = new ReentrantLock();
public void increment() {
lock.lock();
try {
count++;
} finally {
lock.unlock();
}
}
public void commonMethod() {
// Non-synchronized method
}
public void decrement() {
lock.lock();
try {
count--;
} finally {
lock.unlock();
}
}
}
In the above example, the ReentrantLock
is used to synchronize the increment()
and decrement()
methods while leaving commonMethod()
unsynchronized.
The Bottom Line
In Java, synchronizing different methods on the same object is crucial for ensuring thread safety and data consistency in multithreaded applications. The choice between using the synchronized
keyword and ReentrantLock
depends on the specific requirements of the application. While synchronized
keyword provides simplicity and ease of use, ReentrantLock
offers more advanced features and greater control. It's essential to analyze the trade-offs and choose the synchronization mechanism that best fits the application's needs.
Overall, understanding the various approaches to synchronizing methods in Java empowers developers to write efficient and thread-safe code, thereby eliminating potential data inconsistencies and conflicts in multithreaded environments. By employing synchronization effectively, Java developers can ensure the reliability and correctness of their applications in the face of concurrent access to shared resources.