Optimizing Multi-Node Cassandra Performance
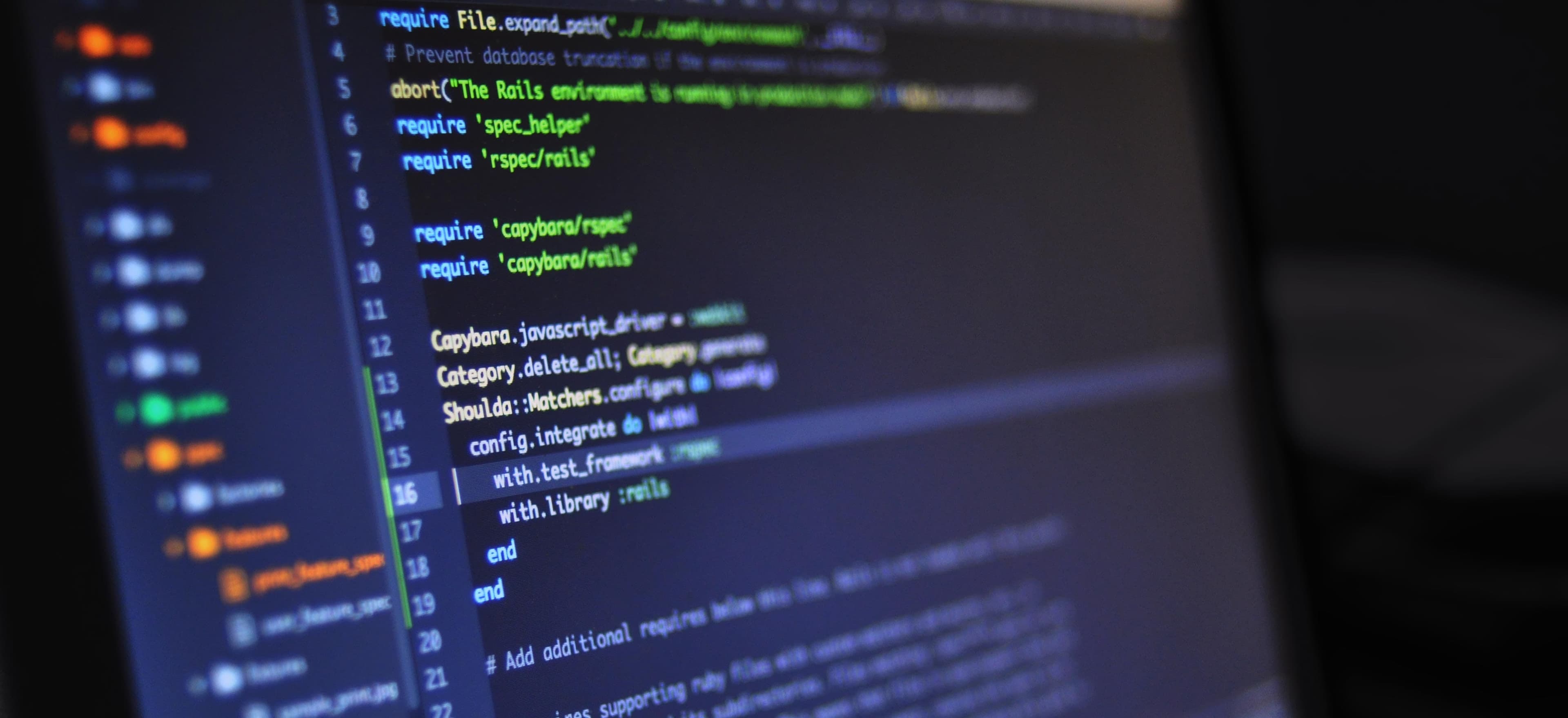
- Published on
Maximizing Multi-Node Cassandra Performance
In the world of distributed databases, performance is key. When dealing with large datasets spread across multiple nodes, optimizing performance becomes even more crucial. Apache Cassandra, with its distributed architecture, offers high availability and scalability. However, to truly maximize its performance in a multi-node setup, a combination of best practices and advanced tuning is required.
In this article, we will delve into various strategies and techniques for optimizing the performance of a multi-node Cassandra cluster. We will cover areas such as data modeling, consistency levels, compaction strategies, and tuning configuration parameters to achieve the best possible performance.
Data Modeling
Data modeling in Cassandra is quite different from traditional relational databases. It involves denormalization and designing tables based on queries. In a multi-node setup, efficient data modeling is critical for even data distribution and balanced query loads across nodes.
Partitioning and Clustering Keys
Choosing the right partition and clustering keys is fundamental for distributed query performance. Data distribution across nodes is determined by the partition key, while the clustering key dictates how data is sorted within a partition.
CREATE TABLE sensor_data (
sensor_id UUID,
event_time timestamp,
value double,
PRIMARY KEY (sensor_id, event_time)
);
In the above example, sensor_id
serves as the partition key, ensuring that data for each sensor is distributed evenly across the cluster. The event_time
column acts as the clustering key, organizing data within each partition based on time.
Avoiding Hotspots
Hotspots can occur when a disproportionately large amount of data is read from or written to a single partition, leading to performance bottlenecks. To mitigate hotspots, consider using composite partition keys to distribute data evenly.
Read and Write Path Optimization
Efficient read and write operations are crucial in a multi-node Cassandra setup. Tuning consistency levels and optimizing compaction strategies can significantly impact overall performance.
Consistency Levels
Cassandra offers tunable consistency, allowing developers to balance between data access consistency and performance. In a multi-node cluster, choosing the appropriate consistency level is essential for optimizing read and write operations.
// Read with LOCAL_QUORUM consistency level
ResultSet results = session.execute("SELECT * FROM sensor_data WHERE sensor_id = ?",
ConsistencyLevel.LOCAL_QUORUM, sensorId);
Using the LOCAL_QUORUM
consistency level ensures that read operations are performed locally on a quorum of replicas within the local data center, reducing cross-data center latency and improving performance.
Compaction Strategies
Compaction is the process of merging and purging SSTables to optimize disk space and read performance. In a multi-node Cassandra cluster, choosing the right compaction strategy can significantly impact read and write performance.
By utilizing the DateTieredCompactionStrategy
, outdated data can be efficiently removed, avoiding excessive disk space usage and improving read performance.
Tuning Configuration Parameters
Cassandra provides a plethora of configuration options for fine-tuning performance. In a multi-node setup, tweaking these parameters can make a substantial difference in overall cluster performance.
Memory Configuration
Proper memory allocation is vital for efficient read and write operations. Allocating an optimal heap size and configuring off-heap memory can drastically improve performance in a multi-node cluster.
# Recommended memory settings for a multi-node Cassandra cluster
MAX_HEAP_SIZE="8G"
HEAP_NEWSIZE="800M"
MAX_DIRECT_MEMORY="2G"
By allocating sufficient memory to the JVM heap and off-heap memory, you can reduce the frequency of garbage collection and improve overall performance.
Network Configuration
In a multi-node setup, network configuration plays a crucial role in inter-node communication. Configuring optimal settings for internode communication and encryption can enhance overall cluster performance.
# Configuring internode communication and encryption
listen_address: node1_ip
broadcast_address: node1_ip
rpc_address: 0.0.0.0
seed_provider:
- class_name: org.apache.cassandra.locator.SimpleSeedProvider
parameters:
- seeds: "node1_ip, node2_ip"
Properly configuring the listen_address
, broadcast_address
, and rpc_address
ensures efficient internode communication, reducing latency and improving performance in a multi-node setup.
The Last Word
Optimizing the performance of a multi-node Cassandra cluster requires a comprehensive approach, encompassing efficient data modeling, read and write path optimization, and meticulous tuning of configuration parameters. By following best practices and leveraging advanced tuning techniques, developers can achieve exceptional performance in their distributed Cassandra deployments.
In conclusion, a deep understanding of Cassandra's distributed architecture, combined with strategic optimization, is key to maximizing performance in a multi-node environment. By implementing the discussed strategies and techniques, developers can ensure that their Cassandra clusters perform at their peak, delivering efficient and scalable data operations.
For further reading on Apache Cassandra performance optimization, check out the official documentation and the insightful blog posts on DataStax Academy.
Remember, performance optimization is an ongoing process, and continuous monitoring and refinement are essential for sustaining peak performance in multi-node Cassandra clusters.