Java Development: Overcoming Legacy Code Challenges
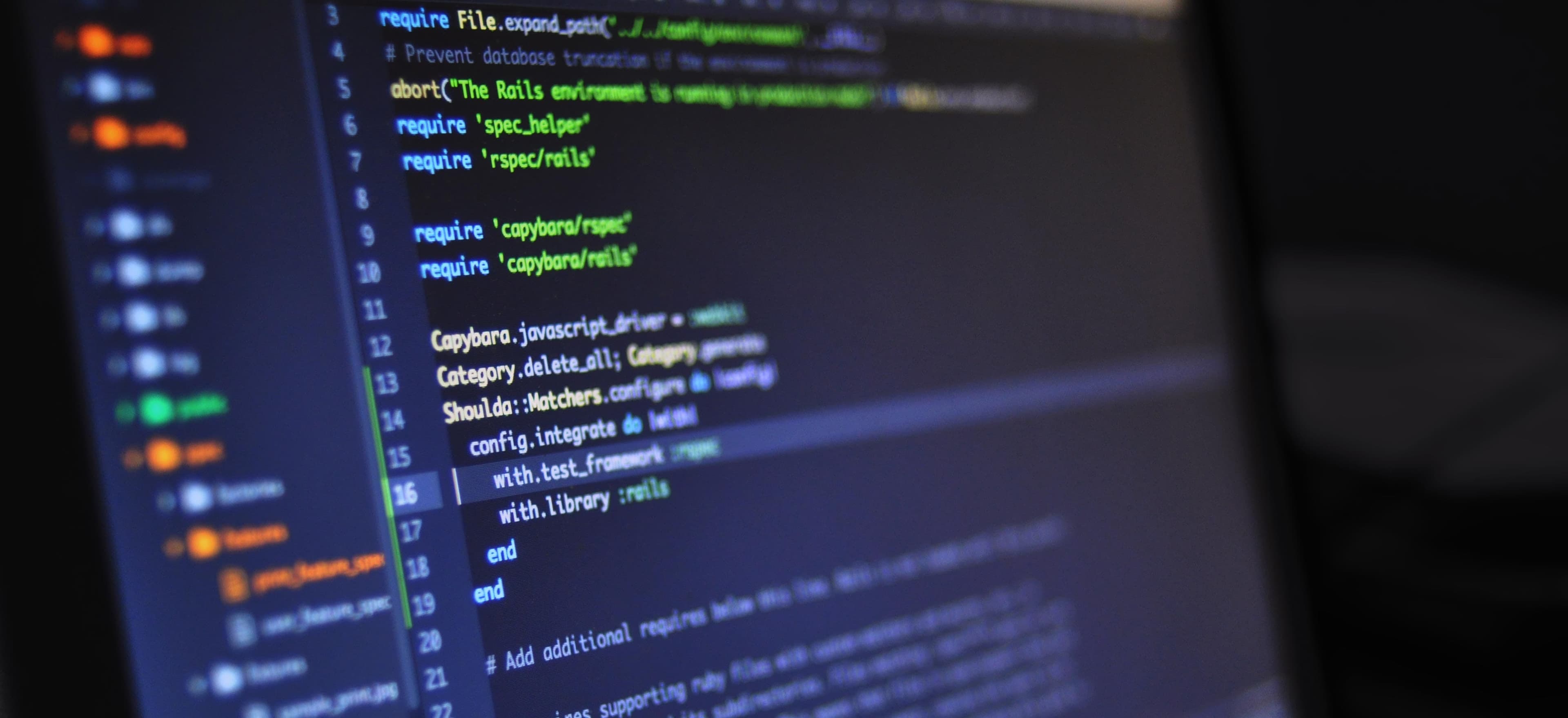
- Published on
Overcoming Legacy Code Challenges in Java Development
In the world of software development, dealing with legacy code is an inevitable reality. Legacy code can be a double-edged sword: on one hand, it represents the foundation of an application, but on the other hand, it can become a significant hurdle when trying to implement new features or fix bugs. In this article, we'll explore some of the common challenges of dealing with legacy code in Java development and discuss effective strategies for overcoming them.
Understanding the Challenges
1. Outdated Technology Stack
Legacy codebases often run on outdated technology stacks, making it challenging to incorporate modern development practices and tools. Upgrading the technology stack while ensuring backward compatibility can be a daunting task.
2. Poor Code Quality
Legacy codebases are notorious for their poor code quality. They may lack proper documentation, adhere to outdated coding standards, and feature tightly coupled components, making it difficult to understand and maintain.
3. Lack of Test Coverage
In many legacy systems, the absence of comprehensive test coverage poses a significant challenge. This makes it risky to introduce changes, as there's a higher probability of unintended side effects.
4. Unwieldy Dependencies
Legacy applications often rely on outdated or unsupported third-party libraries and dependencies. Incorporating new features may require working around these dependencies or finding suitable alternatives.
5. Resistance to Change
Legacy codebases are sometimes entrenched in their ways, making it challenging to introduce new development paradigms or refactor existing code without resistance from the existing codebase and/or team members.
Strategies for Overcoming Legacy Code Challenges
1. Refactoring
Refactoring the legacy code to adhere to modern coding standards and best practices can go a long way in improving its maintainability and extensibility. This can involve breaking down monolithic code into more modular components, eliminating redundant code, and improving the overall code structure.
// Example of refactoring: Extracting a method to eliminate code duplication
public void processOrder(Order order) {
validateOrder(order);
// Business logic for processing the order
}
private void validateOrder(Order order) {
// Validation logic for the order
}
The 'why' behind this refactoring is to improve code readability, maintainability, and reduce the risk of introducing bugs when validating orders.
2. Incremental Modernization
Instead of attempting a complete overhaul of the legacy codebase, adopting an incremental modernization approach can be more practical. This involves identifying and prioritizing critical areas for improvement and gradually introducing modern practices, such as dependency injection, unit testing, and design patterns.
3. Test-Driven Development (TDD)
Introducing a test-driven development approach can help in gradually increasing test coverage and ensuring that new changes do not introduce regressions. Writing unit tests for existing code and incorporating tests for new features can significantly improve the overall stability of the application.
// Example of test-driven development: Writing a test for a service method
@Test
public void testCalculateOrderTotal() {
Order order = // Create an order for testing
double expectedTotal = // Calculate the expected total
double actualTotal = orderService.calculateOrderTotal(order);
assertEquals(expectedTotal, actualTotal, 0.001);
}
The 'why' behind TDD is to ensure that new changes do not break existing functionality and to document the expected behavior of the code.
4. Dependency Management
Identifying and updating unwieldy dependencies is crucial for maintaining the security and stability of the application. Gradually migrating to modern, well-supported libraries and frameworks can reduce the technical debt associated with legacy dependencies.
5. Knowledge Transfer and Training
Encouraging knowledge transfer sessions and providing training on modern Java development practices can help in overcoming resistance to change. Collaborative code reviews and pair programming can also facilitate the adoption of new approaches within the team.
Closing Remarks
Dealing with legacy code in Java development requires a mix of strategic planning, technical acumen, and effective collaboration. By understanding and addressing the challenges posed by legacy code, developers can gradually transform a cumbersome codebase into a robust and maintainable application. While it may involve initial investment and patience, the payoff in terms of improved performance, security, and developer satisfaction can be significant.
In conclusion, embracing a proactive mindset, incorporating modern development practices, and fostering a culture of continuous improvement are all essential in overcoming legacy code challenges in Java development.
Remember, facing legacy code is not just about rewriting it, but rather about understanding it, respecting it, and gradually modernizing it for future success.
By adopting these strategies, Java developers can navigate the complexities of legacy code and pave the way for a more sustainable and adaptable codebase.
For more insights into modern Java development, check out Java's official documentation and Baeldung's comprehensive Java resources.
Checkout our other articles