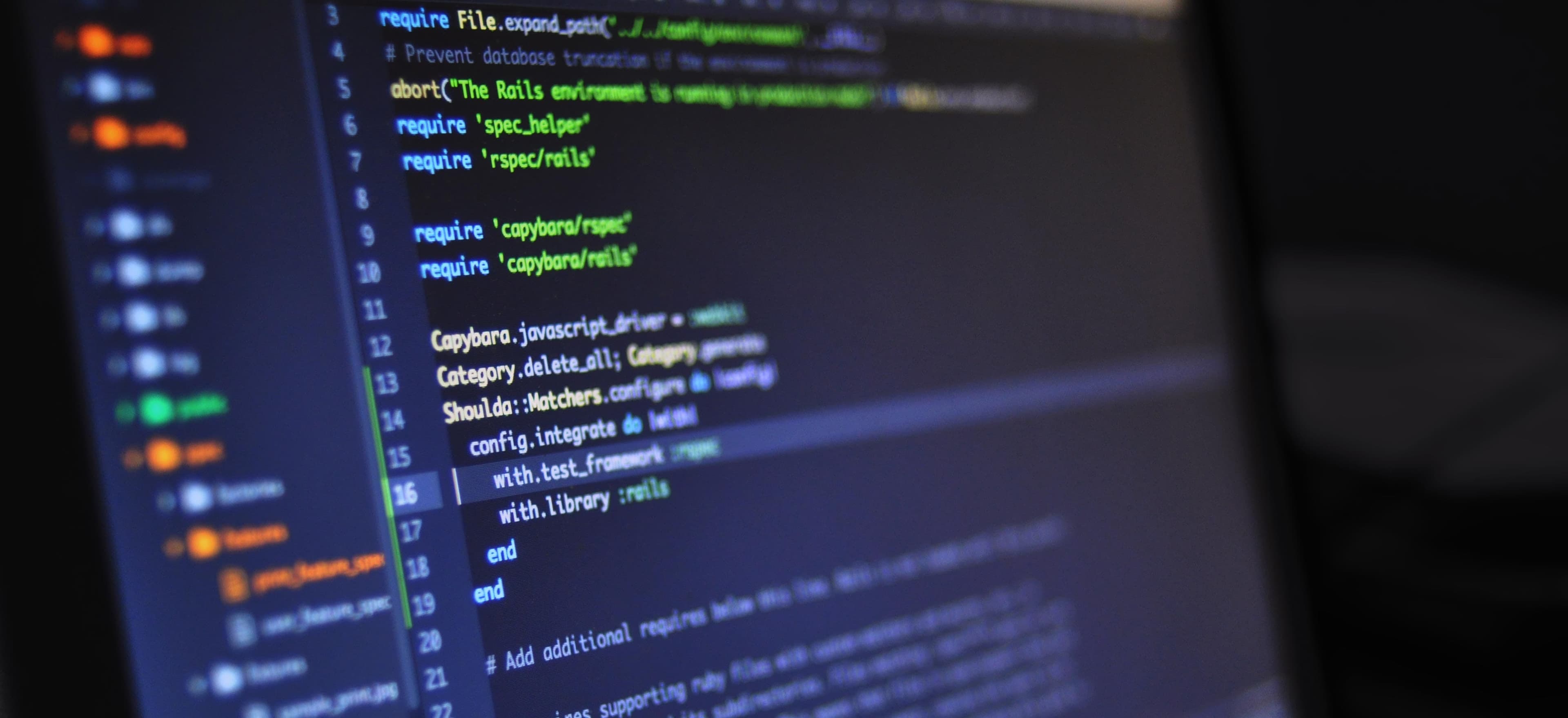
- Published on
Customizing RequestMappingHandlerMapping to Handle Specific Endpoints
In Java Spring applications, the RequestMappingHandlerMapping
is responsible for mapping HTTP requests to handler methods of controller classes. While this default behavior works well for most cases, there are scenarios where you may need to customize the mapping logic for specific endpoints. In this article, we'll explore how to extend and customize the RequestMappingHandlerMapping
to handle specific endpoints in a Java Spring application.
Understanding RequestMappingHandlerMapping
The RequestMappingHandlerMapping
is a core component of the Spring Framework that resolves method-level mappings to handler methods based on the @RequestMapping
annotations and other mapping annotations such as @GetMapping
, @PostMapping
, etc. It is responsible for determining which controller method should handle a particular HTTP request based on factors such as the request URL, request method, and request parameters.
Extending RequestMappingHandlerMapping
To customize the behavior of the RequestMappingHandlerMapping
, we can create a custom implementation that extends the RequestMappingHandlerMapping
class and overrides its methods as needed. This allows us to intercept the request mapping process and apply custom logic for specific endpoints.
Let's consider a scenario where we want to customize the request mapping logic for a specific endpoint, /customEndpoint
, and handle it differently than the standard mapping behavior. We can achieve this by creating a custom HandlerMapping
implementation.
import org.springframework.web.servlet.handler.RequestMappingHandlerMapping;
public class CustomRequestMappingHandlerMapping extends RequestMappingHandlerMapping {
@Override
protected HandlerMethod lookupHandlerMethod(String lookupPath, HttpServletRequest request) throws Exception {
if ("/customEndpoint".equals(lookupPath)) {
// Custom logic for handling the /customEndpoint
// Return the HandlerMethod for the custom handling
}
// Fallback to default behavior for other endpoints
return super.lookupHandlerMethod(lookupPath, request);
}
}
In the code snippet above, we extend the RequestMappingHandlerMapping
class and override the lookupHandlerMethod
method. Within this method, we can implement custom logic to handle the /customEndpoint
differently than the default behavior. For all other endpoints, we delegate to the default behavior by calling super.lookupHandlerMethod(lookupPath, request)
.
Registering Custom HandlerMapping
After creating our custom HandlerMapping
implementation, we need to register it with the Spring application context to ensure that it is used for request mapping.
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Bean
public CustomRequestMappingHandlerMapping customRequestMappingHandlerMapping() {
return new CustomRequestMappingHandlerMapping();
}
@Override
public void configurePathMatch(PathMatchConfigurer configurer) {
configurer.setUseRegisteredSuffixPatternMatch(true);
}
}
In the WebConfig
class above, we create a @Bean
for our custom CustomRequestMappingHandlerMapping
and override the configurePathMatch
method to configure path matching options. Spring will automatically detect and use our custom HandlerMapping
implementation when processing request mappings.
Applying Custom Logic
With our custom RequestMappingHandlerMapping
, we have the flexibility to apply any custom logic for specific endpoints. This could involve conditionally routing requests, applying custom security checks, or any other custom behavior required for the specific endpoint.
For example, we can implement custom authorization logic for the /customEndpoint
as follows:
@Override
protected HandlerMethod lookupHandlerMethod(String lookupPath, HttpServletRequest request) throws Exception {
if ("/customEndpoint".equals(lookupPath)) {
if (isAuthorized(request)) {
return // HandlerMethod for the custom handling
} else {
return super.lookupHandlerMethod("unauthorized", request);
}
}
return super.lookupHandlerMethod(lookupPath, request);
}
private boolean isAuthorized(HttpServletRequest request) {
// Custom authorization logic
}
In the code snippet above, we check if the request is for the /customEndpoint
and then perform a custom authorization check using the isAuthorized
method. Based on the authorization result, we return the appropriate HandlerMethod
for handling the request.
Key Takeaways
Customizing the RequestMappingHandlerMapping
provides a powerful way to handle specific endpoints differently within a Java Spring application. By extending the default behavior, we can apply custom logic to specific endpoints while retaining the standard mapping behavior for other endpoints. This flexibility allows us to address diverse requirements such as custom security checks, conditional routing, and specialized handling for specific endpoints.
In summary, by extending and customizing the RequestMappingHandlerMapping
, we can tailor the request mapping logic to meet the specific needs of our Java Spring application.
To dive deeper into the topic, you may find it helpful to explore the official Spring Framework documentation for more insights into request mapping and handler mapping in Spring MVC.
In conclusion, the ability to customize the RequestMappingHandlerMapping
in Java Spring applications equips developers with the flexibility to handle specific endpoints with tailored logic, contributing to the overall robustness and adaptability of the application.