Generating Dynamic Tests in JUnit: How To Implement Flexible Test Suites
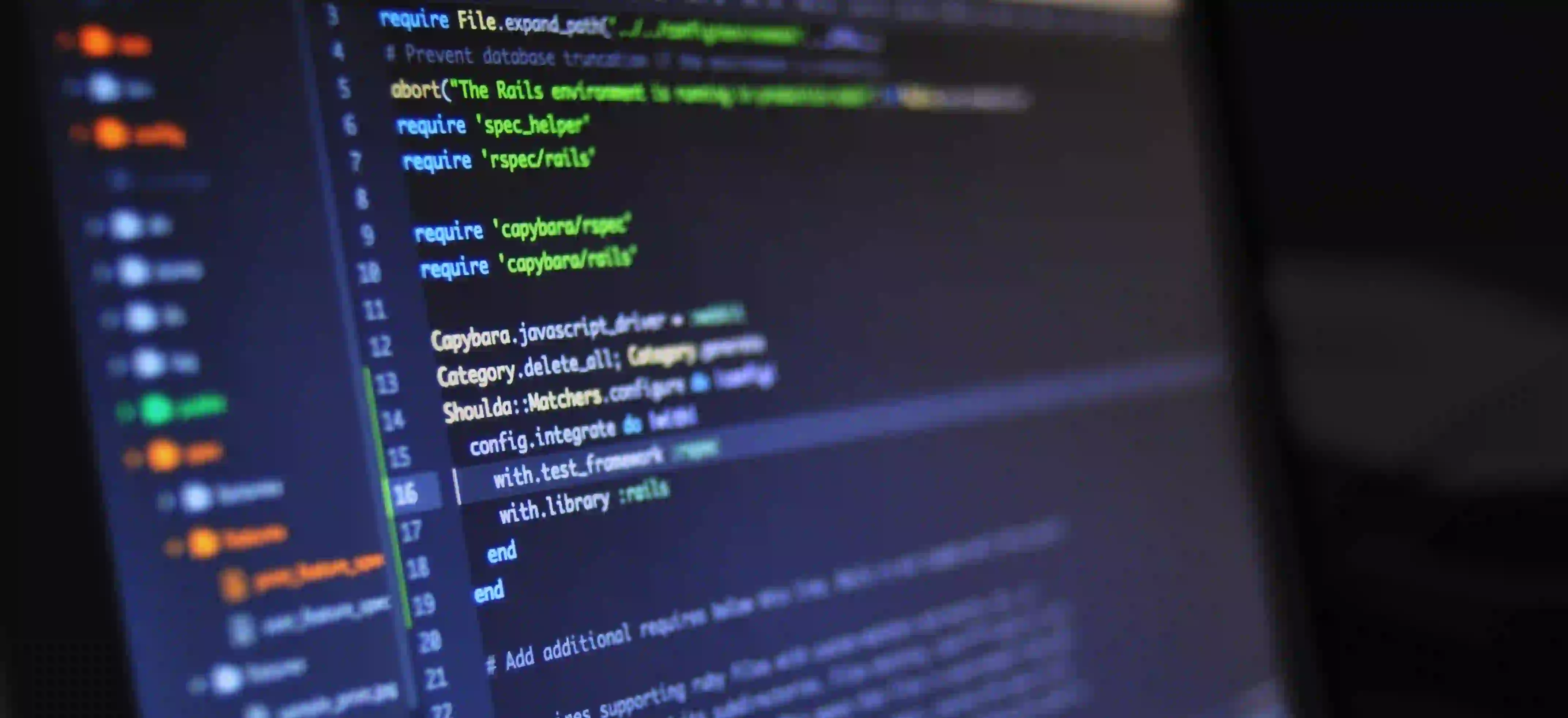
Generating Dynamic Tests in JUnit: How To Implement Flexible Test Suites
When working with JUnit, the popular testing framework for Java, there are scenarios where you need to generate tests dynamically based on certain conditions or data sets. This is where dynamic tests come into play, allowing you to generate tests at runtime rather than hardcoding them.
In this post, we will explore how to implement dynamic tests in JUnit to create flexible test suites that can adapt to changing requirements and input data.
What Are Dynamic Tests?
Dynamic tests in JUnit refer to the ability to create tests at runtime based on the input data or conditions. Unlike traditional tests that are predefined at compile time, dynamic tests allow for the generation of tests during execution.
Why Use Dynamic Tests?
Dynamic tests can be particularly useful in scenarios where:
- Parameterized Testing: You need to run the same test logic with different input parameters.
- Conditional Testing: You want to generate tests based on certain conditions or criteria.
- Data-Driven Testing: You want to generate tests based on data sets.
By using dynamic tests, you can write more flexible and reusable test suites, reduce code duplication, and adapt your tests to changing requirements without modifying the test code.
Implementing Dynamic Tests in JUnit 5
JUnit 5 introduced a new feature called the @TestFactory
annotation, which allows for the dynamic generation of tests. Let's dive into how you can implement dynamic tests using @TestFactory
and explore some common use cases.
Setting Up the Project
Before we begin, make sure you have JUnit 5 configured in your Java project. You can add the following dependency to your pom.xml
if you use Maven:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
If you're using Gradle, add the following dependency to your build.gradle
:
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.8.1'
Creating Dynamic Tests with @TestFactory
To create dynamic tests in JUnit 5, you need to annotate a method with @TestFactory
and have it return a Stream
or Collection
of DynamicTest
instances. Each DynamicTest
represents a single dynamically generated test.
Let's consider an example where we want to test different implementations of a Calculator
interface. Instead of writing separate test methods for each implementation, we can use dynamic tests to generate tests for each implementation.
import org.junit.jupiter.api.*;
import java.util.stream.Stream;
public class CalculatorTest {
Calculator calculator;
@BeforeEach
void setUp() {
// Initialize the calculator instance
}
@TestFactory
Stream<DynamicTest> testAddOperationForImplementations() {
return Stream.of(
dynamicTest("Add operation test for implementation A", () -> {
// Test the add operation for implementation A
}),
dynamicTest("Add operation test for implementation B", () -> {
// Test the add operation for implementation B
}),
// Add more dynamic tests for additional implementations
);
}
// Other test methods...
}
In this example, the testAddOperationForImplementations
method returns a Stream
of DynamicTest
instances, each representing a test for a specific implementation of the Calculator
interface.
Common Use Cases for Dynamic Tests
Parameterized Testing
Dynamic tests can be used for parameterized testing, where you want to run the same test logic with different input parameters. This can be achieved by dynamically generating tests based on the input parameters.
@TestFactory
Stream<DynamicTest> testAddOperationWithParameters() {
List<Pair<Integer, Integer>> testCases = Arrays.asList(
Pair.of(2, 3),
Pair.of(5, 5),
// Add more test cases
);
return testCases.stream()
.map(pair -> dynamicTest("Add operation test with parameters " + pair.getFirst() + " and " + pair.getSecond(), () -> {
// Test the add operation with the given parameters
}));
}
Conditional Testing
You can use dynamic tests to generate tests based on certain conditions or criteria. For example, you may want to test different scenarios based on the environment or configuration settings.
@TestFactory
Stream<DynamicTest> testEnvironmentSpecificScenarios() {
if (isProductionEnvironment()) {
return Stream.of(
dynamicTest("Production-specific test 1", () -> {
// Test specific to production environment
}),
dynamicTest("Production-specific test 2", () -> {
// Another test specific to production environment
})
);
} else {
return Stream.of(
dynamicTest("Test for non-production environment", () -> {
// Test specific to non-production environment
})
);
}
}
Data-Driven Testing
Dynamic tests are well suited for data-driven testing, where you want to generate tests based on data sets. This can be useful when testing functionality with a variety of input data.
@TestFactory
Stream<DynamicTest> testDataDrivenTests() {
List<String> inputStrings = Arrays.asList("hello", "world", "foo", "bar");
return inputStrings.stream()
.map(input -> dynamicTest("Test for input: " + input, () -> {
// Test the functionality with the given input
}));
}
Running Dynamic Tests
When you run your JUnit tests, the dynamic tests will be generated and executed alongside the traditional tests. You will see the results for each dynamically generated test in the test report.
The Bottom Line
Dynamic tests in JUnit provide a powerful way to create flexible test suites that can adapt to changing requirements and input data. By leveraging the @TestFactory
annotation and DynamicTest
instances, you can write more reusable and adaptable tests, ultimately improving the maintainability of your test code.
In this post, we've explored how to implement dynamic tests in JUnit 5 and covered common use cases for dynamic testing, such as parameterized testing, conditional testing, and data-driven testing. By incorporating dynamic tests into your testing strategy, you can enhance the versatility and effectiveness of your test suites.