Mastering Java Syntax and Best Practices
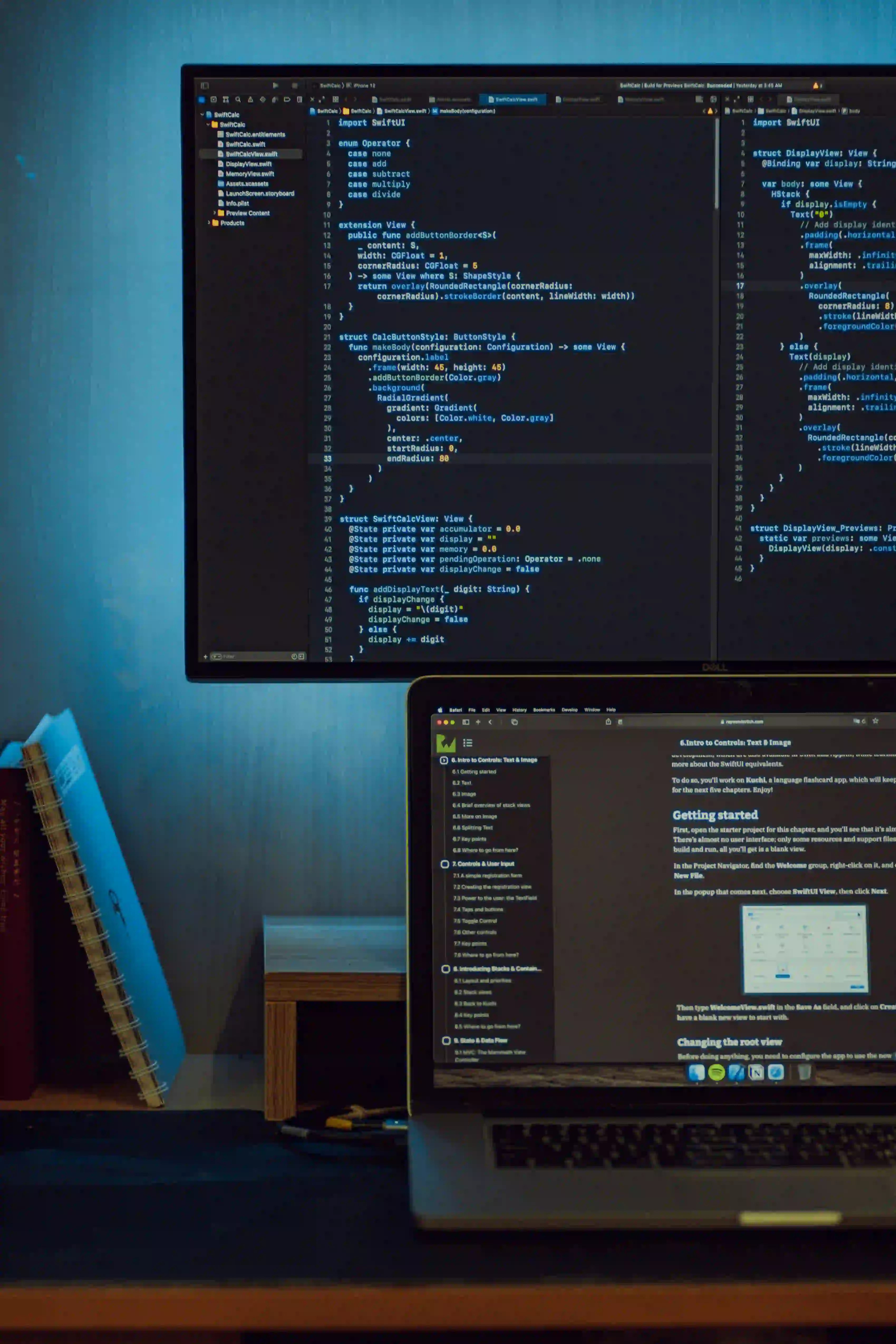
Mastering Java Syntax and Best Practices
When it comes to Java programming, mastering the syntax and best practices is crucial for writing efficient, readable, and maintainable code. Whether you're a beginner or an experienced developer, understanding the nuances of Java syntax and following best practices can significantly enhance your coding skills. In this article, we'll delve into the essential aspects of Java syntax and explore some best practices to help you level up your Java programming.
1. Understanding Java Syntax
Variables and Data Types
In Java, every variable must be declared with a specific data type. Primitive data types such as int
, double
, boolean
, etc., are used to store simple values. On the other hand, reference data types like String
, arrays, and classes are used to store complex objects. It's essential to choose the appropriate data type based on the nature of the data you want to store.
// Variable declaration and initialization
int age = 25;
String name = "John Doe";
double salary = 55000.50;
Control Flow Statements
Java provides several control flow statements such as if-else
, switch
, for
, while
, and do-while
to dictate the flow of the program. It's crucial to use these statements judiciously to control the program's execution flow based on various conditions.
// If-else statement
int marks = 85;
if (marks >= 75) {
System.out.println("Excellent!");
} else {
System.out.println("Keep it up!");
}
Methods and Classes
Methods are the building blocks of Java programs, encapsulating a set of actions to be performed. Classes, on the other hand, act as blueprints for creating objects. Understanding how to define methods and classes and how they interact with each other is fundamental to Java programming.
// Method definition
public int add(int a, int b) {
return a + b;
}
// Class definition
public class Person {
String name;
int age;
public void displayInfo() {
System.out.println("Name: " + name + ", Age: " + age);
}
}
2. Best Practices in Java Programming
Use Descriptive and Meaningful Names
When naming variables, methods, classes, and packages, use descriptive and meaningful names that convey the purpose or intent. This enhances code readability and makes it easier for other developers to understand the functionality.
Follow Code Conventions
Adhering to Java code conventions, such as naming conventions, indentation, and formatting, makes the code more consistent and understandable. It's recommended to follow the conventions specified in the Java Code Conventions for better code maintainability.
Avoid Magic Numbers and Strings
Avoid using hard-coded constants, also known as magic numbers and strings, directly in the code. Instead, define them as named constants using final
keyword to improve code modifiability and readability.
public static final int MAX_ATTEMPTS = 3;
public static final String WELCOME_MESSAGE = "Welcome to our application!";
Exception Handling
Proper exception handling is essential for writing robust and reliable Java code. Always handle exceptions at an appropriate level and throw meaningful exceptions with clear error messages to aid in debugging and troubleshooting.
try {
// Code that may throw an exception
} catch (IOException e) {
// Exception handling and error logging
}
Use Generics and Collections
Utilize Java generics and collections to write type-safe and efficient code when dealing with data structures such as lists, maps, and sets. Generics enable you to define classes, interfaces, and methods that operate on objects of various types parameterized at compile time.
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
Final Thoughts
Mastering Java syntax and following best practices not only improves the quality of your code but also builds a strong foundation for your programming skills. By understanding the intricacies of Java syntax and embracing best practices, you can write clean, maintainable, and efficient code that aligns with industry standards.
In conclusion, honing your skills in Java syntax and best practices is an ongoing process that requires practice, dedication, and continuous learning. As you continue to refine your Java programming skills, remember to keep abreast of the latest developments and trends in the Java ecosystem for a well-rounded proficiency.
Now, armed with a solid understanding of Java syntax and best practices, go forth and write impeccable Java code!
Remember, Rome wasn't built in a day, and mastery of Java syntax and best practices takes time and effort. But the journey is rewarding, and the destination is mastery!