Optimizing Performance of RESTful Endpoints
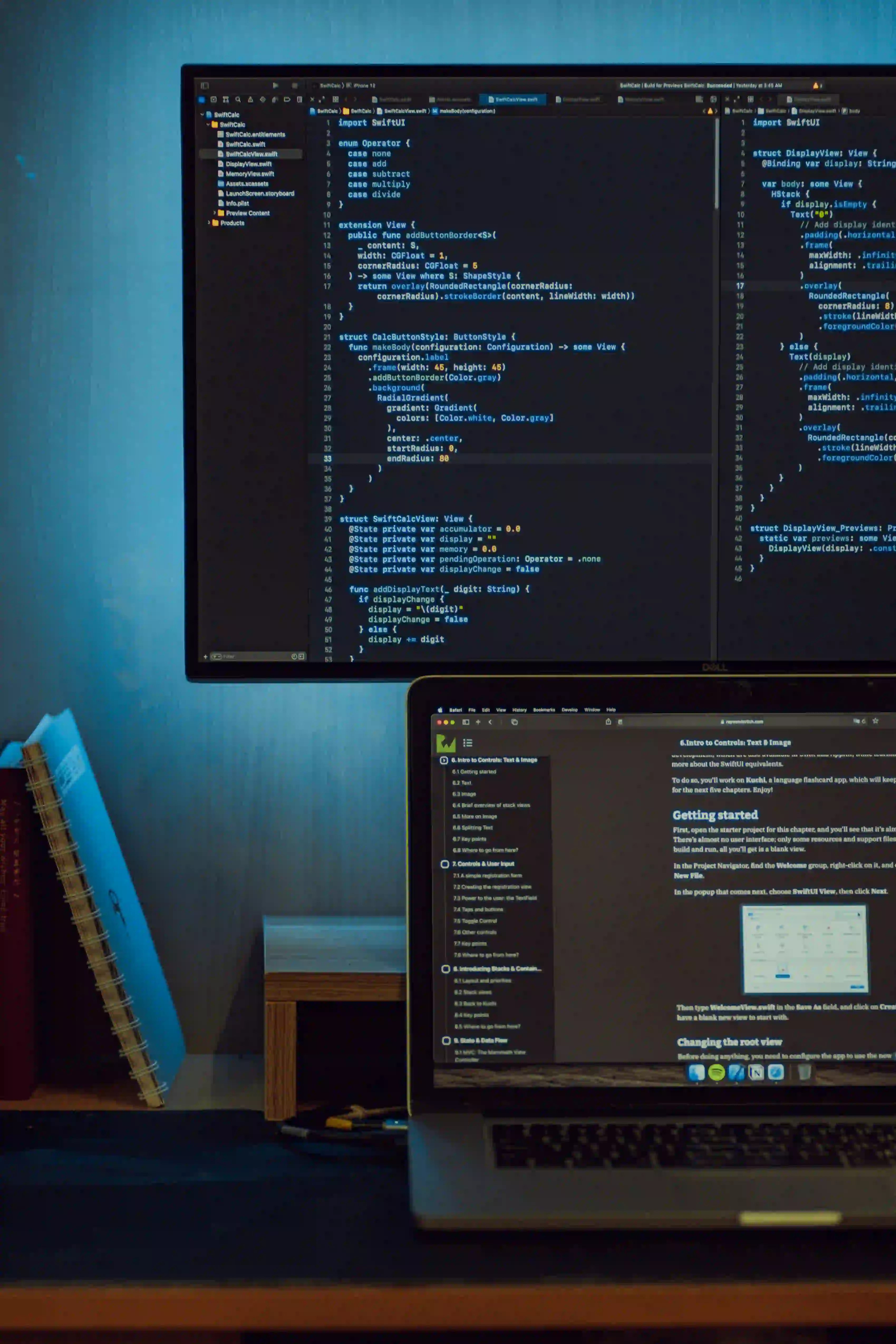
Optimizing Performance of RESTful Endpoints
In the world of web development, creating efficient and high-performing RESTful endpoints is crucial for delivering a seamless user experience. Performance optimization of RESTful endpoints is a multifaceted task that involves various aspects of system design, architecture, and code implementation.
Utilizing Proper Data Structures
Efficient data structures are essential for optimizing the performance of RESTful endpoints. When dealing with large datasets, it's crucial to select the appropriate data structures to ensure fast data retrieval and manipulation. For example, choosing the right data structure (such as HashMap, ArrayList, or LinkedList) based on the specific use case can significantly impact endpoint performance.
// Example of utilizing HashMap for efficient data retrieval
Map<String, User> userMap = new HashMap<>();
// Populate userMap with data
User user = userMap.get(userId);
Using the right data structures not only improves endpoint performance but also enhances the overall scalability and maintainability of the system.
Employing Caching Mechanisms
Implementing caching mechanisms can greatly boost the performance of RESTful endpoints by reducing the need to repeatedly fetch data from the database or perform intensive computations. By caching frequently accessed data or computed results, the endpoint's response time can be significantly improved.
// Applying caching using caffeine library
LoadingCache<String, User> userCache = Caffeine.newBuilder()
.maximumSize(100)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build(key -> getUserFromDatabase(key));
Caching not only accelerates data retrieval but also reduces the load on backend systems, enhancing the overall system performance.
Asynchronous Processing
Utilizing asynchronous processing is instrumental in optimizing the performance of RESTful endpoints, especially when handling long-running tasks or concurrent operations. By offloading resource-intensive operations to background threads or utilizing asynchronous frameworks like CompletableFuture in Java, endpoints can continue to serve other requests without being blocked.
// Using CompletableFuture for asynchronous processing
CompletableFuture<User> futureUser = CompletableFuture.supplyAsync(() -> getUserFromDatabase(userId));
// Continue with other operations while the user data is being fetched asynchronously
Asynchronous processing ensures that endpoints remain responsive and efficient, even under heavy workloads or complex operations.
Efficient Database Querying
Efficient database querying is pivotal for enhancing the performance of RESTful endpoints. Utilizing proper indexing, query optimization, and employing database-specific optimizations can significantly reduce query execution time and improve overall endpoint responsiveness.
// Example of using JPA Criteria API for efficient query building
CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder();
CriteriaQuery<User> criteriaQuery = criteriaBuilder.createQuery(User.class);
// Build and execute optimized database queries
By fine-tuning database queries and leveraging database tools, the efficiency of RESTful endpoints can be greatly enhanced.
Minimizing Network Overhead
Minimizing network overhead is essential for optimizing the performance of RESTful endpoints. Techniques such as payload compression, HTTP/2 optimization, and reducing unnecessary data transfer can significantly improve endpoint responsiveness and reduce latency.
// Implementing payload compression using GZIP
@GZIP
@Path("/users")
public Response getUsers() {
// Fetch and return user data
}
By reducing network overhead, RESTful endpoints can deliver faster response times and improved performance, especially in scenarios with limited bandwidth or high network latency.
To Wrap Things Up
Optimizing the performance of RESTful endpoints is a multidimensional task that involves thoughtful consideration of data structures, caching mechanisms, asynchronous processing, database querying, and network optimization. By employing these optimization strategies, developers can ensure that their RESTful endpoints deliver exceptional performance, scalability, and responsiveness.
Optimizing the performance of RESTful endpoints goes beyond just writing code - it's about understanding system dynamics, data flow, and user expectations. By combining robust software engineering principles with a deep understanding of the underlying infrastructure, developers can create RESTful endpoints that not only perform well but also provide a seamless and efficient experience for end users.
For further insights into Java development and optimization, consider exploring Java Performance Optimization and Best Practices for Optimizing Java Code.
Remember, the goal is not merely to write endpoints that work, but to craft endpoints that shine in terms of speed, reliability, and scalability.