Efficient Utilization of Hibernate Pooled-Lo: A Hidden Gem
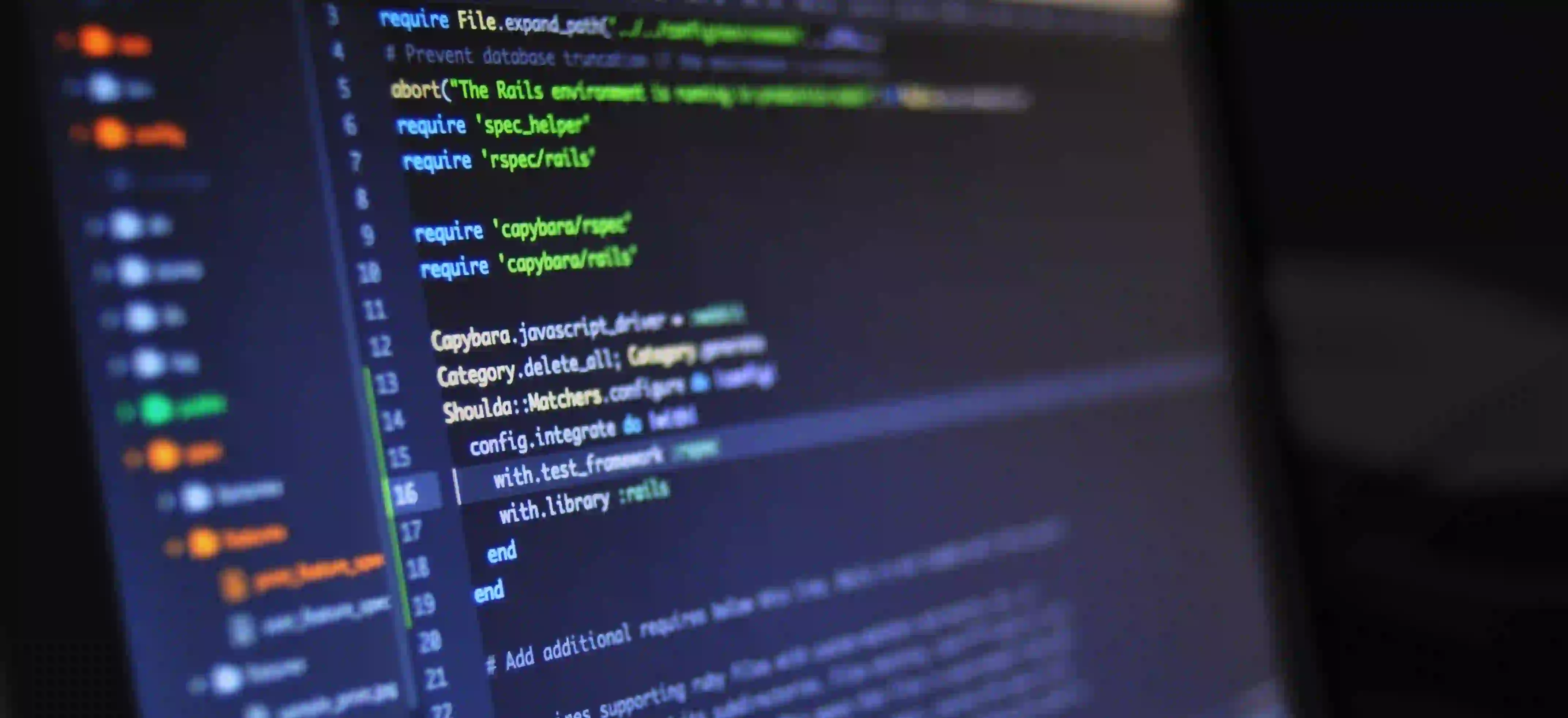
Efficient Utilization of Hibernate Pooled-Lo: A Hidden Gem
When it comes to Java programming and working with databases, Hibernate is a popular framework that simplifies the interaction with databases. One of the lesser-known features of Hibernate is the Pooled-Lo optimization strategy, which can significantly enhance database performance. In this article, we will explore how to efficiently utilize Hibernate Pooled-Lo to improve the performance of your Java applications.
Understanding Hibernate Pooled-Lo
Hibernate uses various identifier generation strategies to generate primary key values for entities. The Pooled-Lo strategy is a high-performance identifier generation strategy that combines the best aspects of both pooled and hi/lo strategies.
In the Pooled-Lo strategy, Hibernate pre-generates a pool of identifiers and assigns a range of these identifiers to each database transaction. This means that multiple database transactions can occur without needing to generate a new identifier for each transaction, leading to improved performance and reduced contention.
Implementing Hibernate Pooled-Lo
Let's delve into the implementation of Hibernate Pooled-Lo with a practical example. Suppose we have an Employee
entity with an auto-generated primary key. We can configure the Pooled-Lo strategy for this entity as follows:
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "employee_id_generator")
@GenericGenerator(
name = "employee_id_generator",
strategy = "org.hibernate.id.enhanced.PooledLo",
parameters = {
@Parameter(name = "initial_value", value = "1"),
@Parameter(name = "increment_size", value = "5")
}
)
@Column(name = "employee_id")
private Long id;
// Other entity properties and methods
}
In this example, we have used the @GenericGenerator
annotation to specify the Pooled-Lo strategy with the initial value and increment size parameters. The initial_value
parameter indicates the starting value for the identifier pool, while the increment_size
parameter specifies the number of identifiers to allocate at once.
Benefits of Using Hibernate Pooled-Lo
Reduced Database Round-Trips
By pre-allocating a pool of identifiers, Hibernate Pooled-Lo reduces the need for frequent database round-trips to generate new identifiers for each database transaction. This leads to improved performance, especially in scenarios with high transaction volumes.
Minimized Contention
With the Pooled-Lo strategy, multiple database transactions can operate concurrently without contending for new identifier generation. This concurrency-friendly approach reduces contention and enhances the scalability of the application.
Optimized Database Performance
The efficient utilization of identifiers through the Pooled-Lo strategy can contribute to optimized database performance by minimizing overhead related to identifier generation, thereby improving overall application responsiveness.
Best Practices for Hibernate Pooled-Lo
While using Hibernate Pooled-Lo can offer significant performance benefits, it is essential to follow best practices to maximize its effectiveness:
-
Optimized Increment Size: Carefully determine the increment size based on the expected transaction volume. A larger increment size can reduce contention but may lead to unused identifiers if transactions are infrequent.
-
Transaction Management: Ensure proper transaction management to make the most out of the pooled identifiers and avoid unnecessary exhaustion of the identifier pool.
-
Monitoring and Tuning: Regularly monitor database performance and tune the identifier pool configuration based on the application's workload to maintain optimal performance.
The Bottom Line
In conclusion, Hibernate Pooled-Lo is a hidden gem that can significantly enhance the performance of Java applications working with databases. By efficiently allocating and reusing identifiers, the Pooled-Lo strategy reduces database round-trips, minimizes contention, and optimizes database performance. When implemented with careful consideration of best practices, Hibernate Pooled-Lo can be a valuable asset in maximizing the scalability and responsiveness of your Java applications.
Incorporating Hibernate Pooled-Lo into your Hibernate configuration can be a game-changer for your Java applications. With the potential for reduced database round-trips, minimized contention, and optimized database performance, it's an optimization strategy worth exploring in your next project.
So, why not consider leveraging Hibernate Pooled-Lo for your next Java application to unlock its hidden potential and elevate performance to new heights?
To learn more about Hibernate Pooled-Lo, check out the official documentation here for detailed insights. Additionally, for further reference on database optimization strategies, consider this insightful article on improving database performance best practices.