Effective Strategies for Software Testing Success
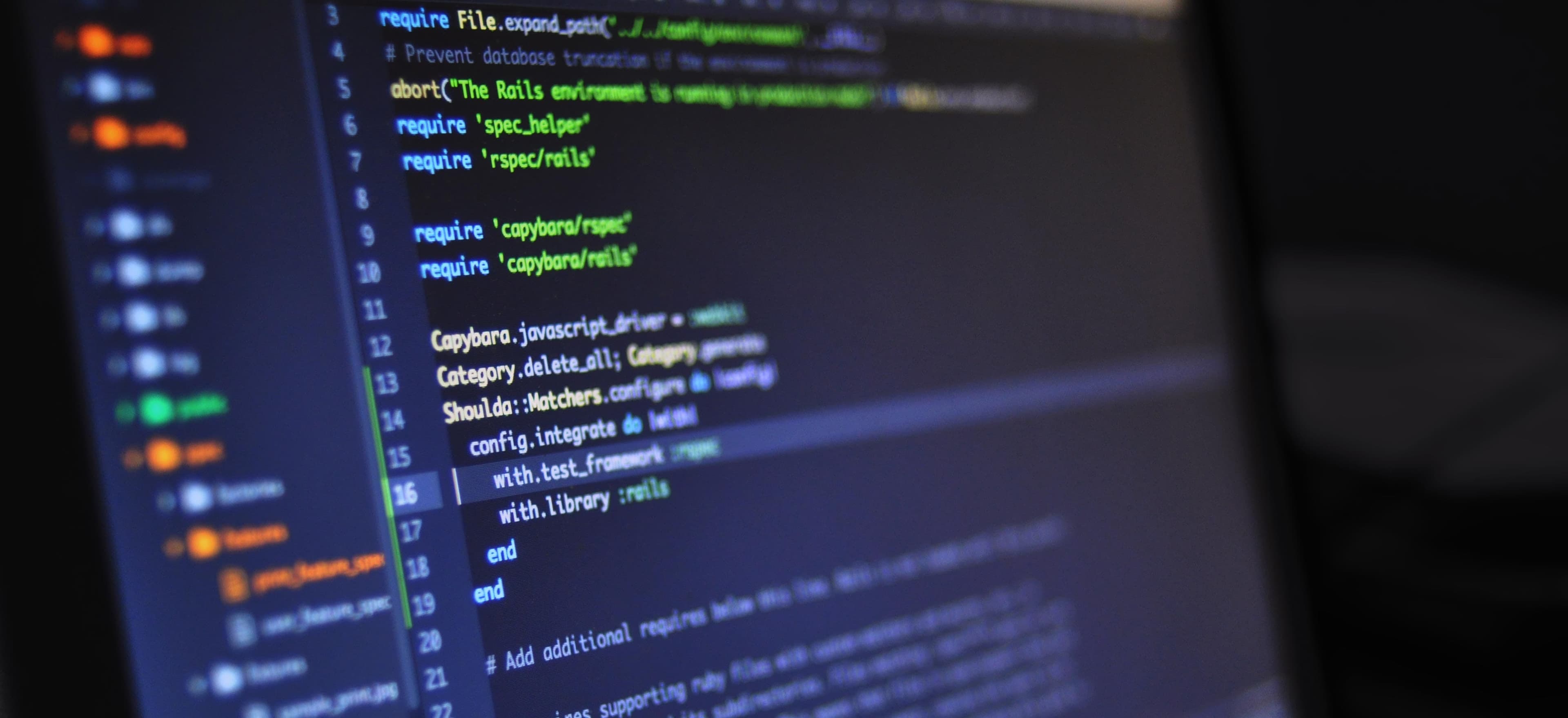
- Published on
Effective Strategies for Software Testing Success
In the world of Java development, software testing is a critical component of ensuring the quality and reliability of a product. Testing allows developers to identify and rectify defects early in the development lifecycle, ultimately leading to a more stable and robust software application. In this article, we will explore some effective strategies for achieving success in software testing within the context of Java development.
Writing Testable Code
Writing testable code is the foundation of successful software testing. Testability is greatly influenced by the design and architecture of the codebase. In Java, adhering to principles such as SOLID (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) can significantly improve the testability of the code.
For instance, by following the Single Responsibility Principle, each class or module focuses on a single concern, making it easier to isolate and test. Utilizing interfaces and dependency injection, as advocated by the Dependency Inversion Principle, allows for easier substitution of dependencies with mock objects during testing.
Consider the following example of a class that violates the Single Responsibility Principle:
public class User {
public void createUser(String username, String password) {
// Business logic for creating a user
}
public void sendEmailConfirmation() {
// Business logic for sending email confirmation
}
}
In this scenario, the User
class is responsible for both user creation and email confirmation. To improve testability, it's better to separate these concerns into distinct classes or methods.
Leveraging Automated Testing
Automated testing plays a crucial role in ensuring the efficiency and reliability of the software development process. In Java, popular frameworks like JUnit and TestNG provide robust support for writing and executing automated tests. By incorporating automated testing into the development workflow, teams can rapidly detect regressions and integration issues, leading to faster feedback loops and higher overall code quality.
Let's consider a simple JUnit test case for a hypothetical Calculator
class:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 4);
assertEquals(7, result);
}
}
In this snippet, we define a test case for the add
method of the Calculator
class. The assertEquals
assertion validates whether the addition operation returns the expected result. Automated tests like this enable developers to validate the behavior of their code with minimal manual effort.
Embracing Test-Driven Development (TDD)
Test-Driven Development (TDD) is a software development approach that emphasizes writing tests before implementing the corresponding functionality. Following the "red-green-refactor" cycle, developers write a failing test, make it pass by writing the minimum amount of code, and then refactor the codebase while ensuring the tests remain green.
The benefits of TDD include improved test coverage, reduced defect rates, and a clearer understanding of the expected behavior of the code. In Java, tools like JUnit and Mockito complement TDD by enabling developers to create tests and mock dependencies effectively.
public class StringManipulator {
public String reverse(String input) {
return new StringBuilder(input).reverse().toString();
}
}
Here, we can drive the development of the reverse
method using TDD, starting with a failing test, implementing the method, and then refactoring as necessary.
Conducting Comprehensive Testing
Comprehensive testing involves encompassing various dimensions of testing, including unit, integration, end-to-end, and performance testing. Each of these testing layers plays a critical role in ensuring the quality and reliability of the software product.
In Java, frameworks like Selenium and JMeter facilitate end-to-end and performance testing, respectively. Integrating these tools into the testing pipeline enables thorough validation of the application's behavior and performance under various conditions.
@Test
public void testUserRegistration() {
// Perform user registration and assert the successful creation of the user account
}
An integration test like the one above validates the end-to-end behavior of the user registration process, including interactions with the database and external dependencies.
Continuous Integration and Continuous Testing
Continuous Integration (CI) and Continuous Testing are integral practices in modern software development. By integrating changes into a shared repository frequently and running automated tests, developers can quickly identify and rectify issues, maintaining a consistent and reliable codebase.
In the context of Java development, CI tools like Jenkins, GitLab CI, and CircleCI alongside testing tools such as JUnit and Mockito form a robust foundation for enforcing continuous integration and testing practices.
The Bottom Line
In the realm of Java development, effective software testing is paramount to building high-quality, resilient applications. By prioritizing testable code, leveraging automated testing, embracing TDD, conducting comprehensive testing, and embracing CI/CD, Java developers can establish a strong testing culture essential for success.
To further elevate your understanding of software testing in Java, consider exploring the detailed documentation of JUnit and TestNG.
Remember, in the world of software development, thorough testing isn't just a phase—it's a mindset. Cheers to building better, more reliable Java applications through the power of effective testing!