Handling Dynamic Routing with Zuul 2
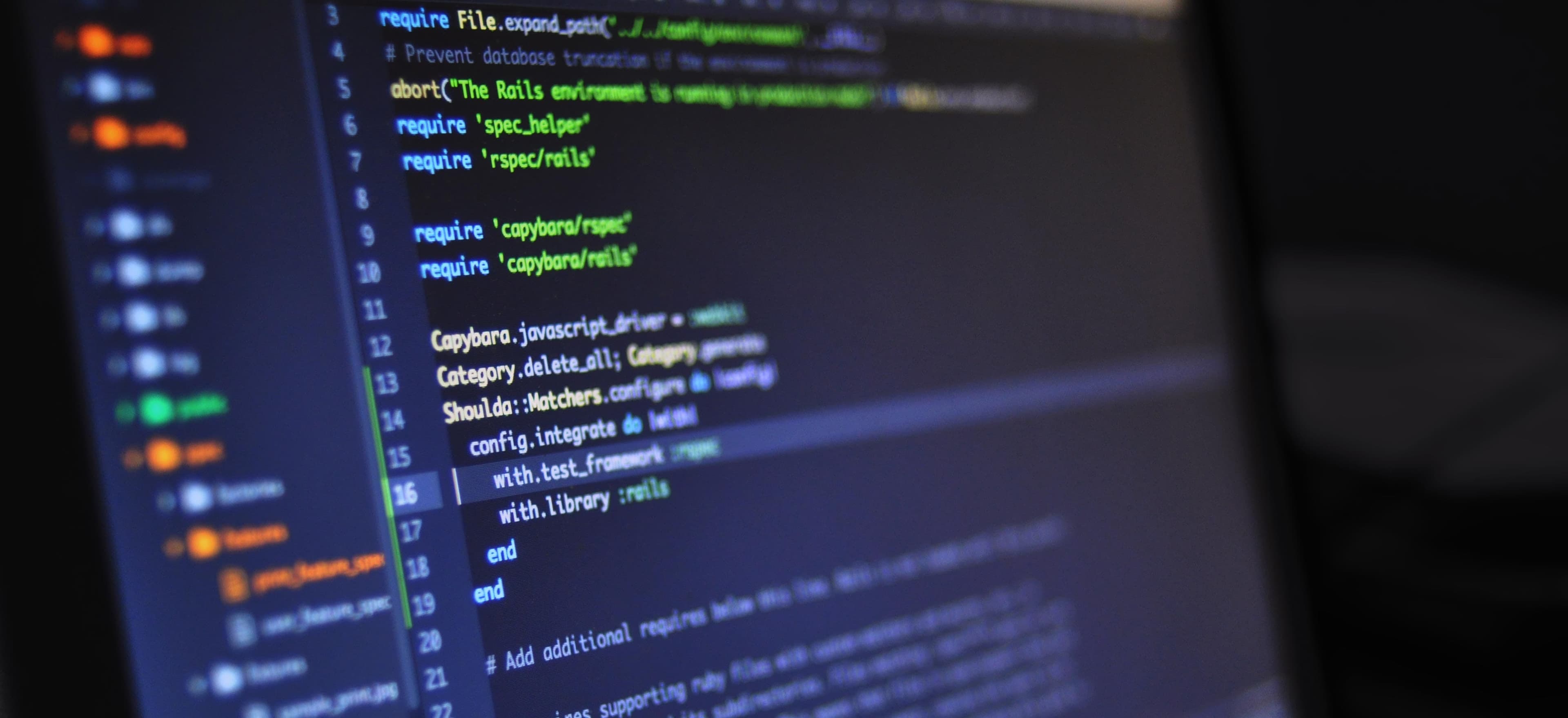
- Published on
Understanding Dynamic Routing in Zuul 2
When it comes to building microservices architecture, handling dynamic routing is a crucial aspect. Zuul 2, which acts as a gateway service, plays a significant role in this scenario. In this article, we will explore the concept of dynamic routing and how it can be effectively managed in Zuul 2.
What is Dynamic Routing?
Dynamic routing refers to the process of routing incoming requests to different backend services based on certain conditions or configurations. This allows for flexibility in managing traffic and providing services without the need to hardcode specific routes for each service.
Zuul 2: The Gateway Service
Zuul 2 is a dynamic routing and load balancing solution that sits at the edge of your microservices architecture. It acts as a gateway, receiving all incoming requests and routing them to the appropriate backend services. Zuul 2 provides the flexibility to dynamically reroute requests based on various factors such as URL patterns, headers, or any other custom criteria.
Dynamic Routing with Zuul 2
Setting up Dynamic Routing Rules
In Zuul 2, dynamic routing rules are defined using a configuration file or a configuration service. These rules specify the conditions under which a request should be routed to a particular service.
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("service-name", r -> r.path("/service/**")
.uri("http://service-host:service-port"))
.build();
}
In the above example, we define a custom RouteLocator
bean to configure dynamic routing for requests that match the "/service/**" pattern. Any requests matching this pattern will be routed to the "service-host" at "service-port". This setup allows for easy management of routing rules without the need for modifying source code.
Dynamic Routing Based on Headers
Dynamic routing can also be based on request headers. For instance, you might want to route requests to different versions of a service based on the version specified in the request header.
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("v1-service", r -> r.path("/v1/**")
.filters(f -> f.addRequestHeader("X-Version", "v1"))
.uri("http://service-host:v1-service-port"))
.route("v2-service", r -> r.path("/v2/**")
.filters(f -> f.addRequestHeader("X-Version", "v2"))
.uri("http://service-host:v2-service-port"))
.build();
}
In this example, requests matching "/v1/" pattern will have the header "X-Version" set to "v1" and will be routed to the corresponding service. Similarly, requests matching "/v2/" pattern will have the header "X-Version" set to "v2" and will be routed accordingly.
Dynamic Routing Based on Custom Criteria
Zuul 2 allows for defining custom criteria for dynamic routing using filters and predicates. This enables more sophisticated routing decisions based on various factors such as request parameters, cookies, or any other custom conditions.
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("custom-route", r -> r.predicate(new CustomPredicate())
.uri("http://custom-service-host:custom-service-port"))
.build();
}
In this example, we define a custom predicate CustomPredicate
to determine the routing based on some custom criteria. This approach provides the flexibility to make routing decisions based on complex conditions specific to your application.
The Bottom Line
Dynamic routing in Zuul 2 offers a powerful way to manage traffic within a microservices architecture. By leveraging dynamic routing rules, based on URL patterns, headers, or custom criteria, Zuul 2 allows for flexible and efficient routing of requests to the respective backend services.
With its ability to dynamically reroute requests, Zuul 2 simplifies the process of managing traffic and ensures a smooth flow of requests within a microservices ecosystem.
In summary, mastering dynamic routing in Zuul 2 is essential for optimizing the performance and scalability of a microservices architecture, and it offers a robust solution for efficient request routing in a dynamic environment.
For more information on Zuul 2 and dynamic routing, you can refer to the official documentation here.
Remember, dynamic routing is a powerful tool, and understanding how to effectively implement it in Zuul 2 can significantly enhance the performance and scalability of your microservices architecture.