Scaling Neo4j with Heroku Add-ons
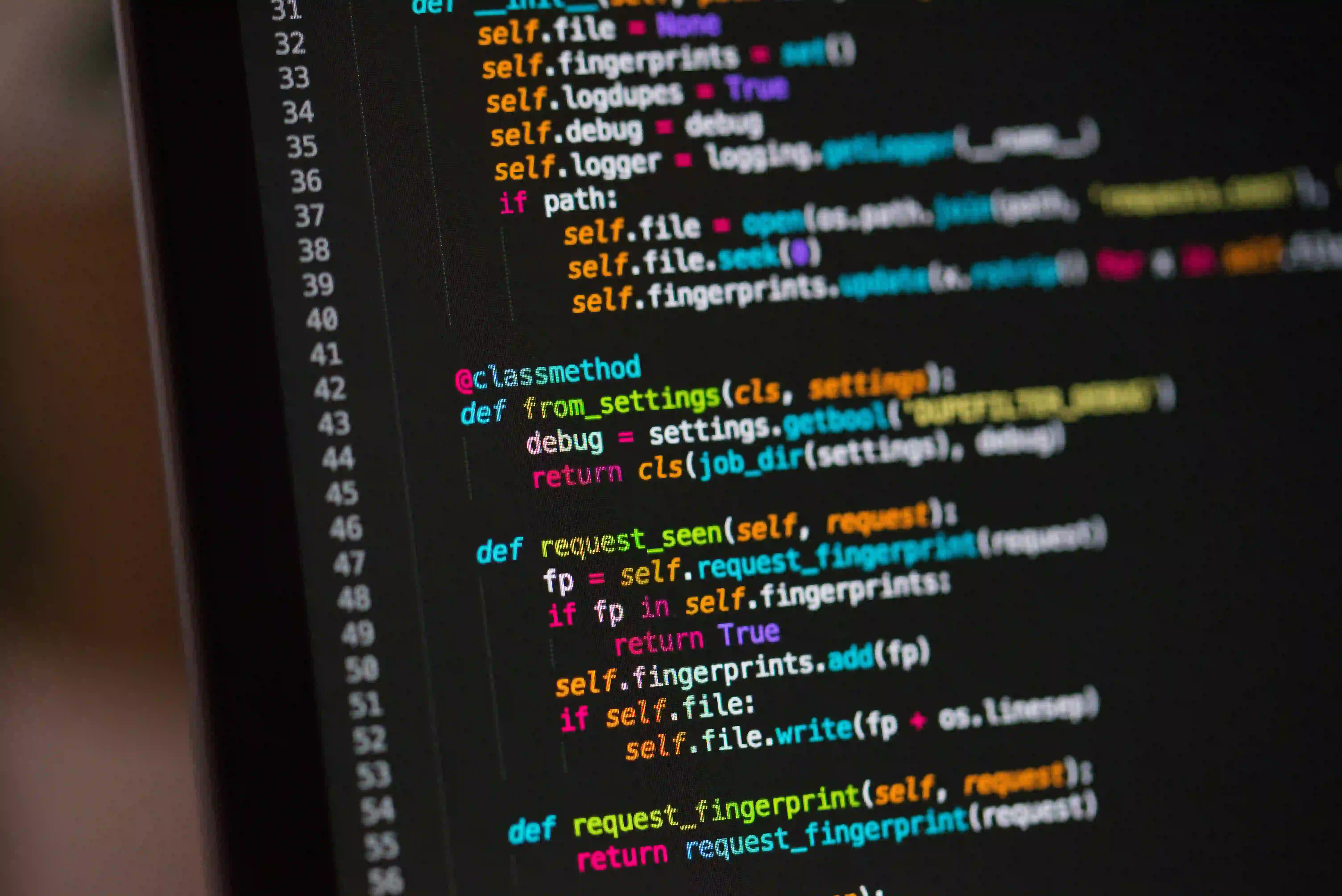
Scaling Neo4j with Heroku Add-ons
When it comes to managing and scaling databases in the cloud, Heroku can be a go-to platform for many developers due to its simplicity and flexibility. In this post, we will explore how to scale Neo4j, a popular graph database, using Heroku add-ons. We will discuss what Neo4j is, how to set it up on Heroku, and how to scale it using add-ons.
What is Neo4j?
Neo4j is a highly scalable native graph database that allows you to model, store, and query graph-structured data with ease. It is known for its flexible data model, powerful query language (Cypher), and strong support for real-time recommendations, fraud detection, and network impact analysis, among other applications.
Setting Up Neo4j on Heroku
Heroku provides an easy way to deploy and manage Neo4j using the official GrapheneDB add-on. To set up Neo4j on Heroku, you can follow these steps:
- Create a new Heroku app or use an existing one.
- Add the GrapheneDB add-on to your Heroku app.
- Configure the Neo4j connection settings provided by GrapheneDB.
Once you have set up Neo4j on Heroku, you can start using it for your applications. However, as your application grows, you may need to scale your Neo4j database to handle increased workload and storage requirements.
Scaling Neo4j with Heroku Add-ons
Heroku provides a range of add-ons that can help you scale Neo4j based on your specific needs. Here are some add-ons that can be useful for scaling Neo4j on Heroku:
1. Heroku Postgres
While Heroku Postgres is a relational database add-on, it can be used in conjunction with Neo4j to offload certain types of data or to perform specific types of queries that are better suited for a relational database. By using Heroku Postgres for such tasks, you can reduce the load on your Neo4j database, thereby effectively scaling it.
2. Redis
Redis is an in-memory data structure store that can be used as a cache or a message broker. By using Redis with Neo4j, you can offload frequently accessed or computationally intensive data from Neo4j, allowing it to handle other tasks more efficiently. Scaling using Redis can improve the overall performance of your Neo4j database.
3. Heroku Kafka
Heroku Kafka is a distributed streaming platform that can be used to build real-time data pipelines and streaming applications. By integrating Neo4j with Heroku Kafka, you can offload certain real-time data processing tasks from Neo4j, thereby improving its scalability and performance for other types of queries and operations.
Code Example: Using Redis to Scale Neo4j
import redis.clients.jedis.Jedis;
public class Neo4jRedisScaler {
private Jedis jedis;
public Neo4jRedisScaler(String host, int port) {
this.jedis = new Jedis(host, port);
}
public void offloadDataToRedis(String key, String data) {
jedis.set(key, data);
}
public String retrieveDataFromRedis(String key) {
return jedis.get(key);
}
}
In the above code example, we have a Neo4jRedisScaler
class that uses the Jedis library to connect to a Redis instance. We can use this class to offload data from Neo4j to Redis, thereby improving the scalability of our Neo4j database.
Final Considerations
Scaling a Neo4j database on Heroku can be achieved using various add-ons to offload specific tasks or types of data from Neo4j. By carefully selecting and integrating these add-ons, you can effectively improve the scalability and performance of your Neo4j database as your application grows.
In conclusion, Heroku provides a flexible and powerful platform for scaling Neo4j, allowing you to meet the demands of your graph database as your application evolves.
Remember, the key to successful scaling is to analyze your specific use case and data patterns to determine the most effective way to scale your Neo4j database using Heroku add-ons.
With the right approach and tools, you can ensure that your Neo4j database remains performant and responsive, even as your application experiences increased traffic and data volumes.