Optimizing Immutable Data: Handling Partial Updates
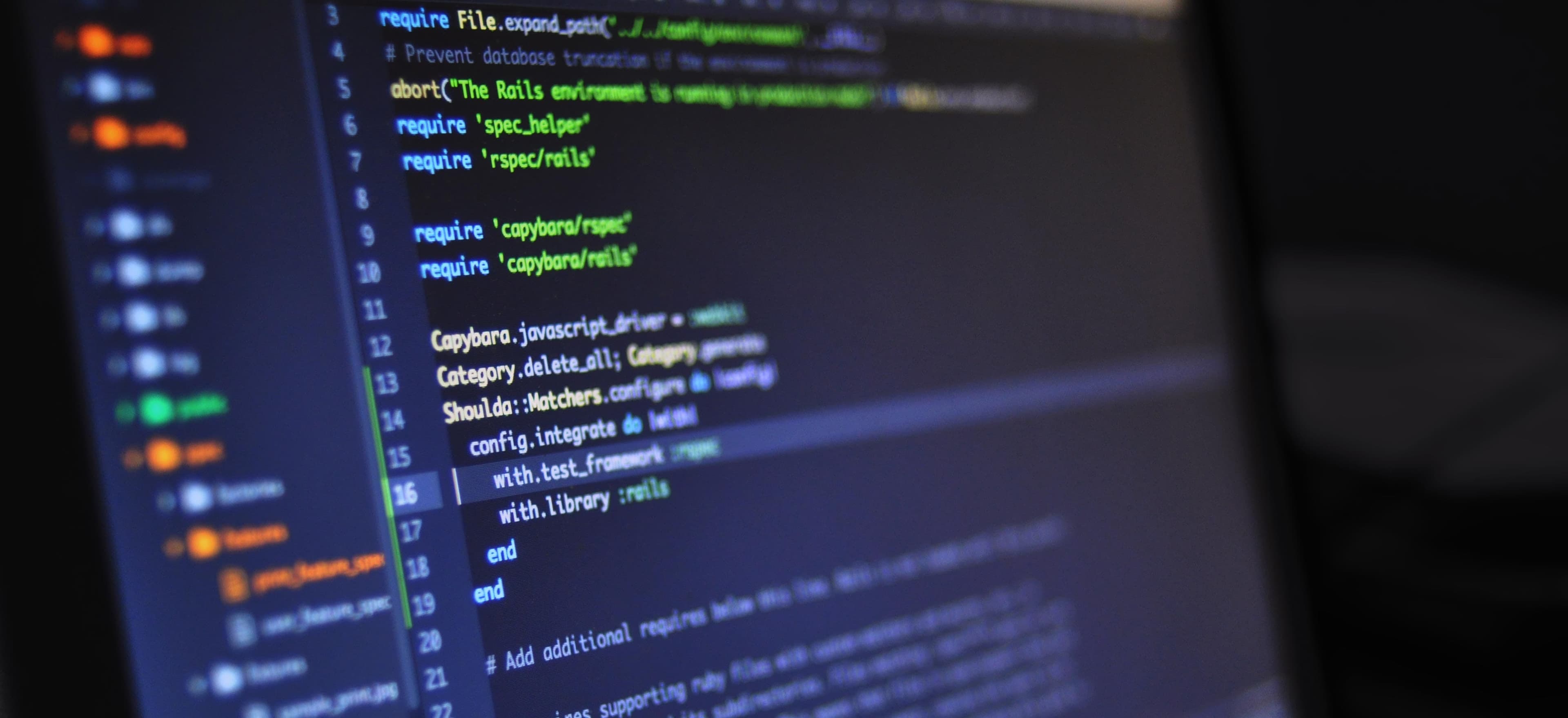
- Published on
Optimizing Immutable Data: Handling Partial Updates in Java
Immutable objects are a powerful concept in Java, offering numerous benefits such as thread safety, easy caching, and the ability to reason about code more effectively. However, when it comes to handling partial updates on immutable objects, things can get a bit tricky. In this blog post, we'll explore some strategies for optimizing the handling of partial updates on immutable data in Java, leveraging the power of the language to write concise, efficient, and maintainable code.
Understanding Immutable Data
Immutable objects are objects whose state cannot be modified after they are created. In Java, this is typically achieved by declaring all fields of an object as final
and initializing them via the constructor. Immutability plays a crucial role in writing reliable and maintainable code, as it eliminates entire classes of bugs related to mutable state.
Consider the following example of an immutable User
class:
public final class User {
private final String name;
private final int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In this example, once a User
object is created, its name
and age
cannot be modified. This simplicity and predictability are the hallmarks of immutable data.
Handling Partial Updates
Now, let's consider a scenario where we want to apply a partial update to our User
object – for instance, updating just the name while keeping the age unchanged. Since the fields of the User
class are declared as final
, we cannot directly modify them. In such cases, we need to create a new object with the updated values.
Using the Builder Pattern
One common approach to addressing partial updates on immutable objects is through the builder pattern. By creating a builder class within the immutable class, we can selectively update the object's fields while maintaining immutability. Here's how we can achieve this for the User
class:
public final class User {
private final String name;
private final int age;
private User(String name, int age) {
this.name = name;
this.age = age;
}
public static class Builder {
private String name;
private int age;
public Builder name(String name) {
this.name = name;
return this;
}
public Builder age(int age) {
this.age = age;
return this;
}
public User build() {
return new User(name, age);
}
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
With the builder pattern in place, we can easily apply partial updates to User
objects:
User originalUser = new User("Alice", 30);
User updatedUser = new User.Builder()
.name("Bob")
.age(originalUser.getAge())
.build();
Leveraging Lombok
Another approach to simplifying the creation of builder classes for immutable objects is to use Lombok. Lombok is a popular library that reduces boilerplate code by automatically generating getters, setters, constructors, and other utility methods.
By annotating the User
class with @Value
, we can instruct Lombok to generate an immutable class with a builder, facilitating the handling of partial updates without writing explicit builder code.
@Value
public class User {
String name;
int age;
}
This approach economizes the syntax, making it easy to work with partial updates:
User originalUser = new User("Alice", 30);
User updatedUser = originalUser.withName("Bob");
Final Thoughts
Handling partial updates on immutable data in Java requires a thoughtful approach to maintaining immutability while allowing for flexible object modification. By leveraging the builder pattern or using libraries like Lombok, we can simplify the process of updating immutable objects, ensuring that our code remains concise, clear, and efficient.
In conclusion, the key to optimizing the handling of partial updates on immutable data in Java lies in striking a balance between immutability and flexibility, ensuring that our code remains robust and easy to reason about.
For further reading on immutable objects and effective Java programming, check out the Java Immutable Objects Tutorial and the Effective Java by Joshua Bloch.
In this blog post, we delved into optimizing the handling of partial updates on immutable data in Java. We explored the concept of immutable data, how to handle partial updates using the builder pattern, and leveraging Lombok for simplifying this process. By adhering to these practices, you can enhance the maintainability and reliability of your Java code. If you've found this post helpful, please share it with your peers and consider implementing these strategies in your future Java projects.