Handling Pop-up Dialogs in ADF: Best Practices
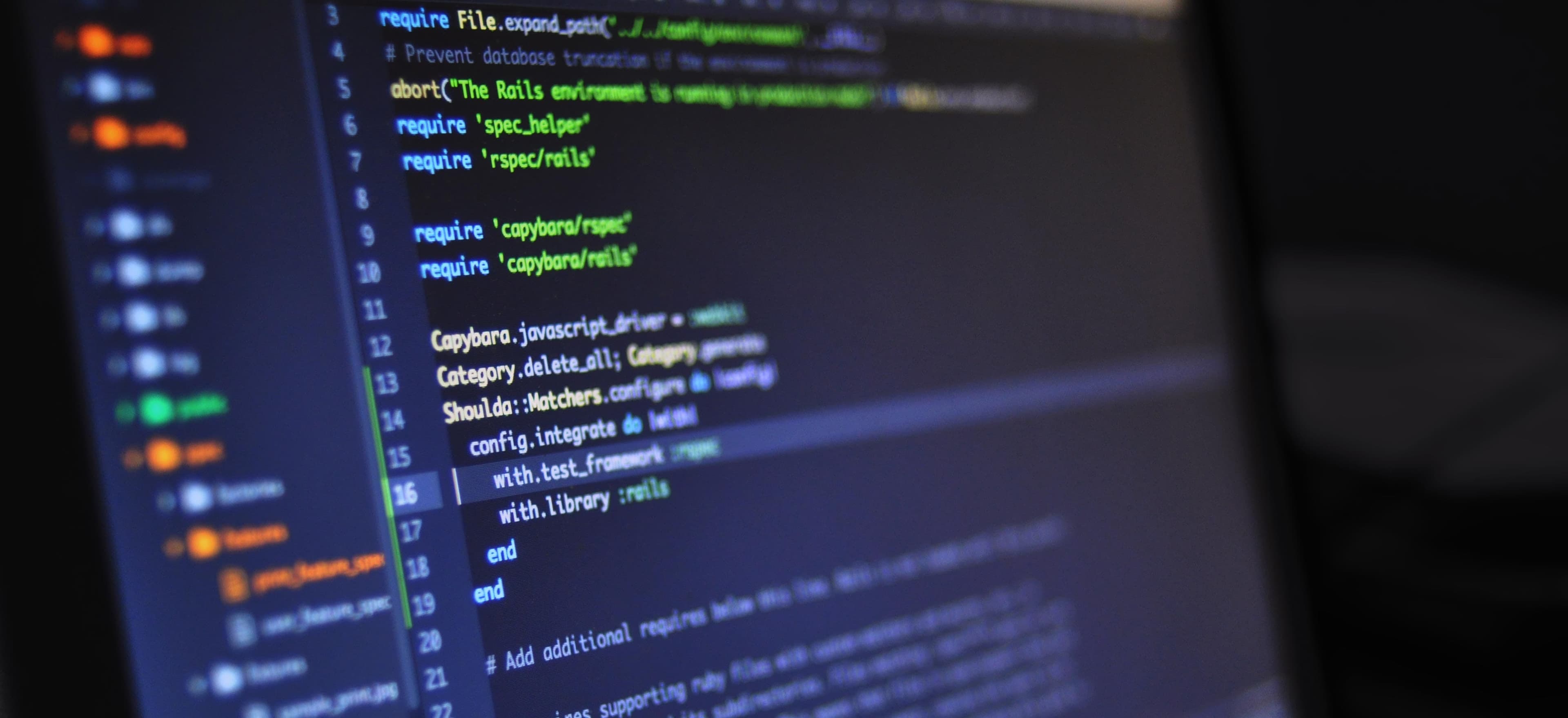
- Published on
Handling Pop-up Dialogs in ADF: Best Practices
Pop-up dialogs are a crucial component in modern web applications. They allow for a better user experience by displaying important information, receiving user input, or confirming actions without navigating away from the current page. In Oracle Application Development Framework (ADF), handling pop-up dialogs efficiently is essential for creating a seamless user interface.
In this article, we'll delve into the best practices for handling pop-up dialogs in ADF applications. We will cover the implementation of pop-up dialogs, managing their lifecycle, and ensuring a smooth user interaction. Let's dive in!
Adding Pop-up Dialogs to ADF Pages
When adding pop-up dialogs to ADF pages, it's important to consider the various ways in which users might interact with them. ADF provides the 'af:dialog' component to create pop-up dialogs. Here's an example of how to define a basic pop-up dialog in an ADF page:
<af:dialog id="popupDialog" title="Confirmation" modal="true">
<af:panelGroupLayout layout="vertical">
<af:outputText value="Are you sure you want to proceed?"/>
<af:button text="Yes" id="yesButton"/>
<af:button text="No" id="noButton"/>
</af:panelGroupLayout>
</af:dialog>
In this example, we've created a simple pop-up dialog with a title, a message, and two buttons for confirmation. The 'modal="true"' attribute ensures that the pop-up dialog blocks interactions with the rest of the page until it is closed.
Managing Pop-up Dialog Lifecycle
Once the pop-up dialog is added to the ADF page, it's important to manage its lifecycle effectively. This includes showing, hiding, and handling user interactions with the dialog. A common best practice is to use bean methods to control the pop-up dialog's behavior.
Here's an example of how to show the pop-up dialog using a bean method:
public void showPopupDialog(RichPopup popup){
if(popup != null){
AdfFacesContext.getCurrentInstance().addPartialTarget(popup);
popup.show(new RichPopup.PopupHints());
}
}
In this code snippet, the 'showPopupDialog' method takes a 'RichPopup' component as an argument and uses ADF Faces context to add the popup component to the current partial page rendering (PPR) target before showing the dialog.
Ensuring Smooth User Interaction
A key aspect of handling pop-up dialogs in ADF is to ensure a smooth and intuitive user interaction. This involves providing clear feedback, handling user input effectively, and managing the pop-up dialog's behavior based on user actions.
For example, when a user clicks the "Yes" or "No" button in the pop-up dialog, it's important to handle these actions appropriately. Here's an example of how to handle the "Yes" button click in a bean method:
public void handleConfirmation(){
// Perform the necessary action upon confirmation
// ...
// Close the pop-up dialog
RichPopup popup = getPopupDialog(); // Implement a method to retrieve the popup dialog component
if(popup != null){
popup.hide();
}
}
In this snippet, the 'handleConfirmation' method performs the necessary action upon confirmation (e.g., saving data, executing a process) and then hides the pop-up dialog.
Best Practices Summary
In summary, when handling pop-up dialogs in ADF, the following best practices should be considered:
- Use the 'af:dialog' component to create pop-up dialogs in ADF pages.
- Manage the pop-up dialog's lifecycle using bean methods and ADF Faces context.
- Ensure smooth user interaction by providing clear feedback, handling user input, and managing the dialog's behavior based on user actions.
By following these best practices, you can create a seamless and effective user experience when handling pop-up dialogs in ADF applications.
The Closing Argument
Pop-up dialogs are a fundamental part of modern web applications, and handling them efficiently is crucial for a positive user experience. In ADF, implementing pop-up dialogs and managing their lifecycle requires careful consideration of user interaction and feedback.
By following the best practices outlined in this article, you can ensure that pop-up dialogs in your ADF application provide a seamless and intuitive experience for users.
Do you have any experiences or additional best practices for handling pop-up dialogs in ADF? Share your thoughts in the comments below!
For more resources on Oracle ADF, visit the official Oracle ADF documentation.