How to Efficiently Use Streams to Index Objects
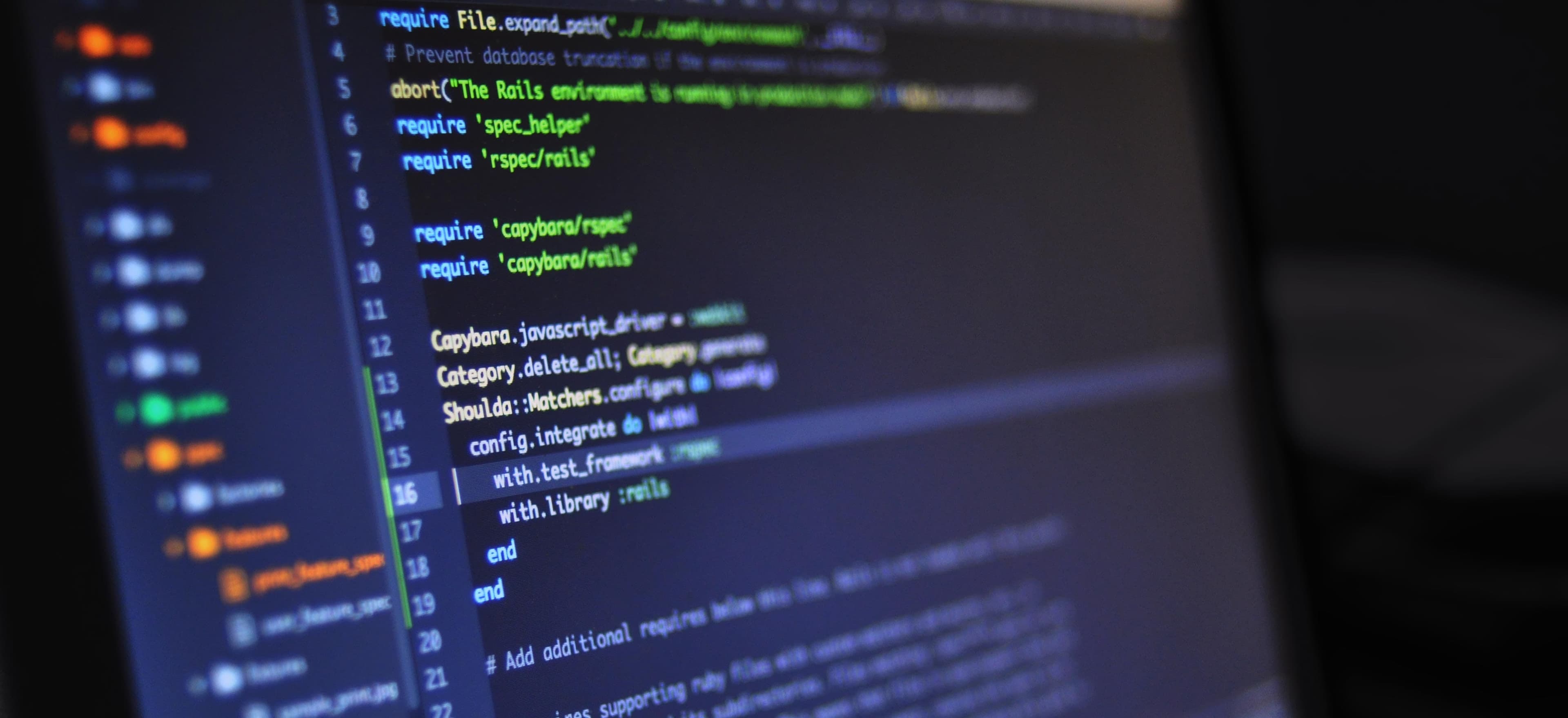
- Published on
Efficient Usage of Streams for Indexing Objects in Java
In Java, streams offer a powerful and efficient way to process and manipulate collections of objects. One common use case is indexing objects based on a specific property or attribute. This article will explore how to efficiently utilize streams for indexing objects in Java.
Understanding the Problem
Imagine you have a collection of objects, and you want to index them based on a specific attribute. For example, you have a list of Person
objects, and you want to index them based on their age. In traditional Java, you might iterate through the list and create a map where the keys are ages and the values are lists of Person
objects with that age. With streams, we can achieve this more efficiently and with cleaner, more concise code.
Using Streams to Index Objects
Let's dive into some code to see how we can efficiently use streams to index objects in Java. First, we'll define a simple Person
class:
public class Person {
private String name;
private int age;
// constructor, getters, and setters
}
Now, suppose we have a list of Person
objects and we want to index them based on their age. We can achieve this using Java streams and the Collectors.groupingBy
collector:
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<Person> people = Arrays.asList(
new Person("Alice", 30),
new Person("Bob", 25),
new Person("Charlie", 30)
);
Map<Integer, List<Person>> peopleByAge = people.stream()
.collect(Collectors.groupingBy(Person::getAge));
System.out.println(peopleByAge);
}
}
In this example, we're using the Collectors.groupingBy
collector to index the Person
objects based on their age. The resulting Map
will have the age as the key and a list of Person
objects with that age as the value.
Why Use Streams for Indexing Objects
Using streams for indexing objects offers several advantages:
- Conciseness: The code is more concise and expressive compared to traditional iterative approaches. This leads to improved readability and maintainability.
- Efficiency: Under the hood, streams leverage parallel processing and other optimizations for efficient operations on collections. This can lead to better performance, especially for large datasets.
- Flexibility: Streams offer a wide range of intermediate and terminal operations, allowing for complex data manipulation and indexing with minimal code.
Applying Stream Operations for Advanced Indexing
While the basic use of groupingBy
is powerful, streams offer even more advanced operations for indexing objects. For example, suppose we want to index the Person
objects based on their age, but we also want to filter out individuals under a certain age. We can achieve this using a combination of filtering
and groupingBy
collectors:
Map<Integer, List<Person>> peopleByAgeFiltered = people.stream()
.filter(person -> person.getAge() >= 30)
.collect(Collectors.groupingBy(Person::getAge));
In this example, we added a filter
operation before the groupingBy
collector to only include Person
objects with an age of 30 or higher in the index.
Key Takeaways
In conclusion, streams provide a powerful, efficient, and expressive way to index objects in Java. By leveraging collectors such as groupingBy
and combining stream operations, we can efficiently manipulate collections and create indices based on specific attributes. This approach leads to more readable, maintainable, and performant code.
The use of streams for indexing objects is a fundamental concept in modern Java development, and mastering these techniques can greatly enhance your ability to work with data in Java applications.
Incorporating stream operations like groupingBy
and filtering
enables you to handle complex indexing requirements with ease, making your code more robust and maintainable.
Overall, understanding and effectively utilizing streams for indexing objects is a valuable skill for any Java developer, and it can significantly improve the quality and efficiency of your code.
To delve even further into the world of Java streams and their application, you can explore the official Java documentation and additional resources available. Happy coding!