Maximizing Concurrency Control with Guava Striped Class
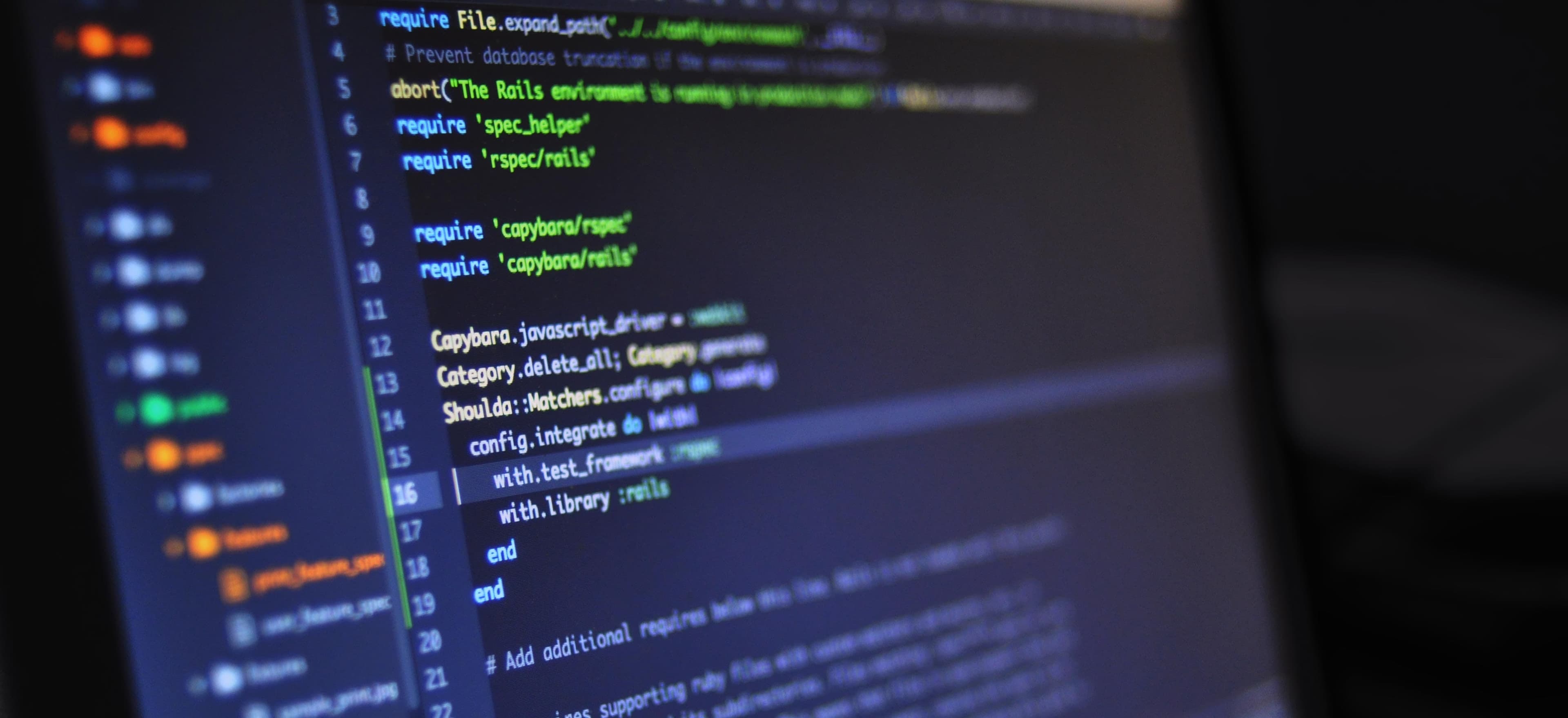
- Published on
Maximizing Concurrency Control with Guava Striped Class
In a multi-threaded environment, managing concurrency effectively is crucial for ensuring the stability and performance of your Java application. One powerful tool for achieving this is the Guava Striped class, which enables fine-grained concurrency control through the use of lock striping. In this blog post, we will explore the benefits of using Guava Striped and demonstrate how it can be leveraged to optimize concurrent data access in Java applications.
Understanding the Need for Concurrency Control
Concurrency issues arise when multiple threads attempt to access and modify shared resources simultaneously. Without proper synchronization mechanisms in place, these concurrent operations can lead to data corruption, race conditions, and inconsistent state within the application. To address these challenges, developers often resort to using locks or synchronization blocks to coordinate access to critical sections of code.
While locks can effectively prevent concurrent access to shared resources, they can also introduce performance bottlenecks and potential deadlocks. Additionally, using a single lock for a large set of resources can lead to unnecessary contention among threads, limiting the level of parallelism achievable in the application.
Introducing Guava Striped for Fine-Grained Concurrency Control
Guava, the popular open-source Java library developed by Google, provides a powerful utility class called Striped that offers a solution to the aforementioned challenges. The Striped class enables fine-grained concurrency control by partitioning the set of resources into a fixed number of stripes, each associated with its own lock. This approach minimizes contention and allows for greater parallelism by reducing the number of threads contending for the same lock.
Leveraging Guava Striped for Concurrent Data Access
Let's consider a scenario where multiple threads need to perform operations on a set of shared resources. We can use Guava Striped to create separate locks for different categories of resources, allowing concurrent access to be more finely controlled.
import com.google.common.util.concurrent.Striped;
import java.util.concurrent.locks.Lock;
// Create a Striped instance with a fixed number of stripes
Striped<Lock> lockStriped = Striped.lock(10);
// Obtain a lock based on a resource key
String resourceKey = "exampleKey";
Lock lock = lockStriped.get(resourceKey);
// Acquire the lock and perform the operation
lock.lock();
try {
// Perform the operation on the shared resource
// ...
} finally {
lock.unlock();
}
In the above code snippet, we create a Striped instance with 10 stripes, indicating that 10 separate locks will be used for controlling concurrent access. We then obtain a lock from the striped instance based on a unique resource key and perform the necessary operation within a synchronized block.
By using Striped, we ensure that concurrent operations on different resource keys are not unnecessarily blocked by each other, leading to improved parallelism and reduced contention among threads.
Benefits of Using Guava Striped
1. Improved Concurrency Control
Guava Striped offers a more granular approach to concurrency control compared to using a single, global lock. By partitioning resources into distinct lock stripes, it minimizes contention and allows for more efficient concurrent access.
2. Scalability and Performance
The use of fine-grained locks provided by Striped can lead to improved scalability and performance in multi-threaded applications. By reducing lock contention, it enables a higher degree of parallelism and throughput.
3. Reduced Risk of Deadlocks
The finer granularity of locks provided by Guava Striped reduces the likelihood of deadlocks occurring due to lock contention. This can lead to more robust and stable concurrent code.
4. Simple and Intuitive API
The API provided by Guava Striped is straightforward and easy to use, making it accessible for developers who need to implement fine-grained concurrency control without diving into low-level synchronization mechanisms.
Wrapping Up
In the context of concurrent data access in Java applications, Guava Striped provides an elegant solution for maximizing concurrency control while minimizing contention and the risk of deadlocks. By leveraging fine-grained locks through the Striped class, developers can ensure optimal performance and stability in multi-threaded environments.
To explore further, you can refer to the official Guava documentation for detailed information on using Guava Striped and its various configuration options.
In summary, Guava Striped stands as an invaluable tool for Java developers seeking to achieve effective concurrency control and optimize the performance of their multi-threaded applications. With its simple yet powerful API, Striped offers a pragmatic approach to addressing the complexities of concurrent data access, making it a valuable addition to any developer's toolkit.