Improving JavaFX2 Game Performance
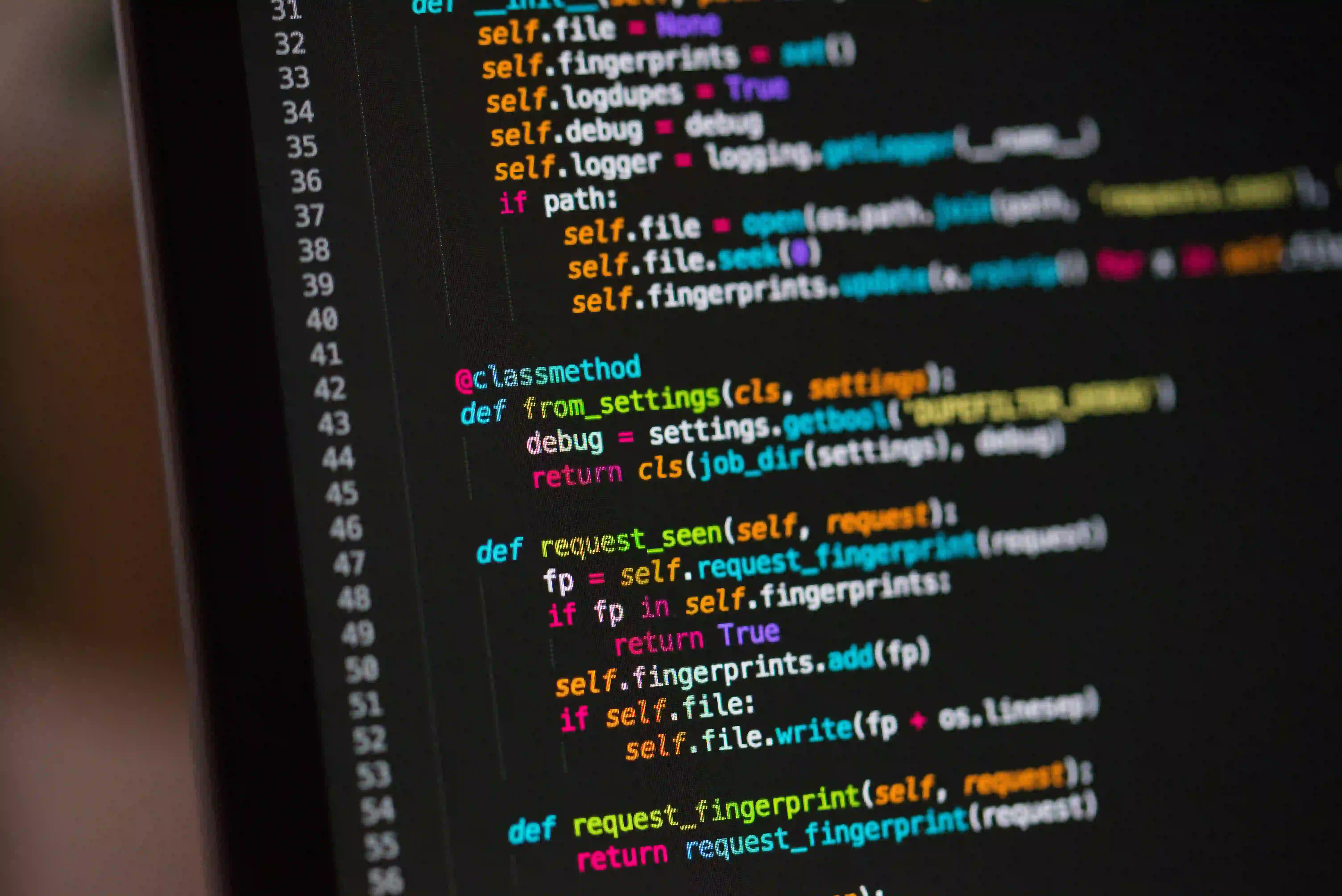
Tips for Improving JavaFX 2 Game Performance
JavaFX 2 provides a powerful platform for developing interactive and visually appealing games. However, as with any application, optimizing performance is crucial for delivering a smooth and enjoyable user experience. In this blog post, we will explore some tips and techniques for improving the performance of JavaFX 2 games.
1. Use Hardware Acceleration
JavaFX 2 provides hardware acceleration support, which can significantly boost the performance of graphical operations. By default, JavaFX 2 applications are hardware accelerated, but it's essential to ensure that hardware acceleration is indeed enabled.
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
// Ensure hardware acceleration is enabled
System.out.println("Is hardware acceleration enabled: " +
Platform.isSupported(ConditionalFeature.SCENE3D));
}
}
2. Optimize Scene Graph
The scene graph in JavaFX 2 represents the visual elements of the application. To improve performance, it's important to optimize the scene graph by reducing the number of nodes and updating only the necessary parts when changes occur.
// Example of optimizing scene graph
Group root = new Group();
for (int i = 0; i < 1000; i++) {
Rectangle rect = new Rectangle(10, 10);
rect.setLayoutX(Math.random() * 800);
rect.setLayoutY(Math.random() * 600);
root.getChildren().add(rect);
}
In the above example, instead of adding each rectangle to the scene graph individually, we added them to a group and then added the group to the scene. This reduces the number of nodes in the scene graph, leading to improved performance.
3. Use Cached Nodes
JavaFX 2 provides the ability to cache nodes, which can significantly reduce the rendering overhead, especially for complex or frequently used graphical elements.
// Caching a Node
ImageView imageView = new ImageView("path_to_image.jpg");
imageView.setCache(true);
By caching the node, JavaFX creates a bitmap with the rendered content, and subsequent rendering operations use this cached bitmap instead of re-rendering the node.
4. Leverage JavaFX Animation API
The JavaFX Animation API provides powerful capabilities for creating fluid animations. When developing games, it's crucial to leverage this API to create smooth and responsive animations.
// Example of using JavaFX Animation API
TranslateTransition transition = new TranslateTransition(Duration.seconds(1), node);
transition.setToX(100);
transition.setAutoReverse(true);
transition.setCycleCount(Animation.INDEFINITE);
transition.play();
In the above example, we use the TranslateTransition to create a smooth animation that moves a node horizontally over one second. By utilizing the Animation API, we can achieve better performance and visual appeal in our games.
5. Optimize Image Loading
Loading images in a game can be a performance-intensive task, especially when dealing with a large number of images. It's vital to optimize image loading by using the appropriate image formats and caching strategies.
// Optimizing image loading
Image image = new Image("path_to_image.jpg", true);
ImageView imageView = new ImageView(image);
In the above code, we use the Image constructor that takes a boolean flag indicating whether to load the image in the background. This can prevent UI blocking during image loading.
6. Implement Efficient Event Handling
Efficient event handling is crucial for game performance. JavaFX 2 provides various event handling mechanisms, and it's essential to implement them efficiently to minimize overhead.
// Efficient event handling
node.setOnMouseClicked(event -> {
// Handle mouse click event
});
By using lambda expressions or event delegation, we can efficiently handle events without introducing unnecessary overhead.
7. Profiling and Optimization
Profiling tools such as Java Mission Control and VisualVM can help identify performance bottlenecks in JavaFX 2 applications. By profiling the application, developers can pinpoint areas that need optimization and make informed decisions to improve performance.
In Conclusion, Here is What Matters
Optimizing the performance of JavaFX 2 games is essential for delivering a seamless and immersive gaming experience. By leveraging hardware acceleration, optimizing the scene graph, using cached nodes, utilizing the Animation API, optimizing image loading, implementing efficient event handling, and employing profiling and optimization tools, developers can significantly enhance the performance of their JavaFX 2 games.
In conclusion, optimizing JavaFX 2 game performance is a multifaceted process that involves leveraging the platform's capabilities and implementing best practices to deliver high-performance, visually appealing games.
By following these tips and techniques, developers can unlock the full potential of JavaFX 2 for game development and provide users with engaging and responsive gaming experiences.
For further exploration of JavaFX 2 game development and performance optimization, visit JavaFX documentation and Java performance tuning tips.