Reading and Understanding Code Comments for Error Indication
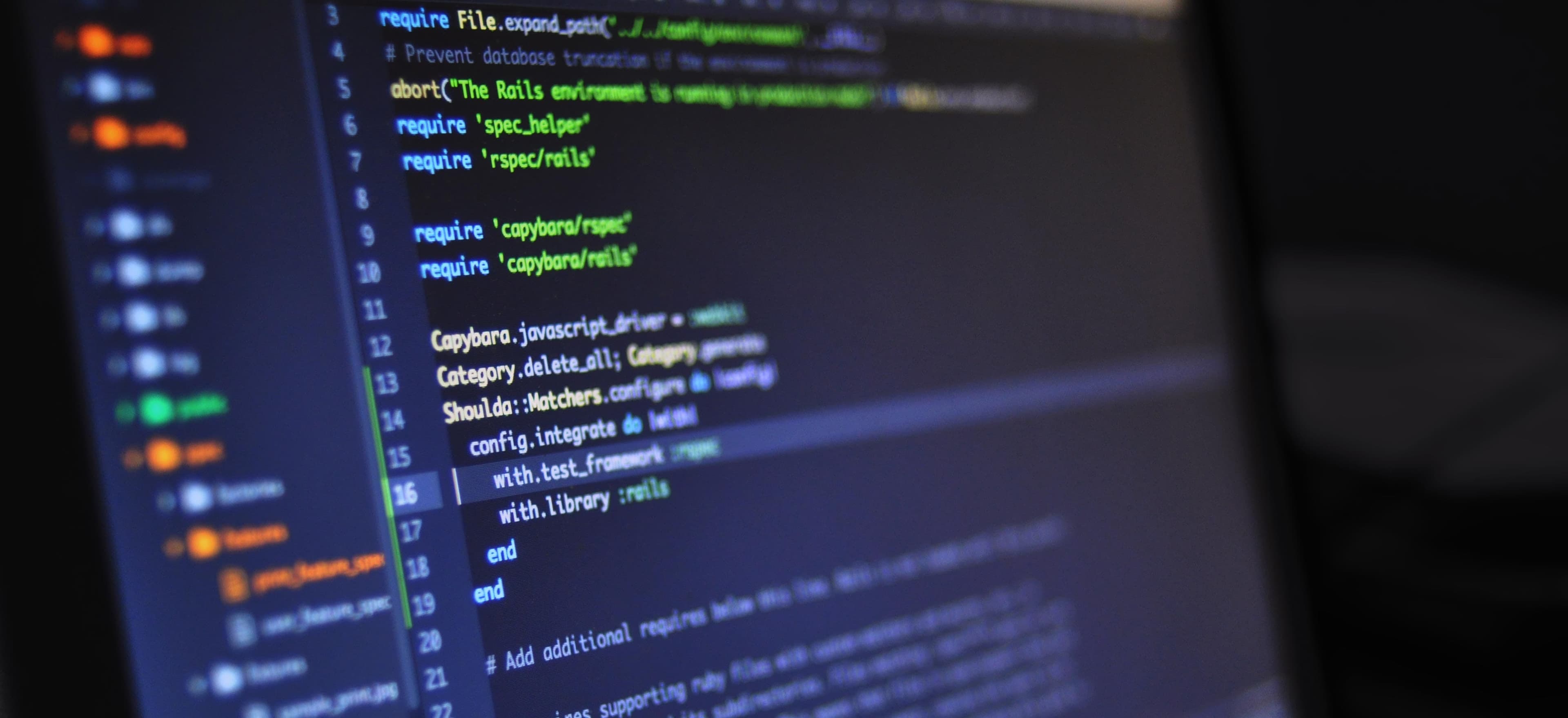
- Published on
Reading and Understanding Code Comments for Error Indication
In the world of programming, code comments play a crucial role in enhancing the readability and maintainability of code. They provide valuable insights into the logic and functionality of the code. However, there is an aspect of code comments that is often overlooked – their role in indicating potential errors or issues within the codebase.
The Role of Code Comments in Error Indication
Code comments can serve as effective indicators of potential errors, especially when they are used to explain complex or non-intuitive code segments. By carefully reading and understanding these comments, developers can preemptively identify and rectify potential issues, thereby reducing the likelihood of errors in the code.
Let’s delve into the key aspects of utilizing code comments for error indication, and how they can be leveraged to enhance code quality and maintainability.
Commenting Error-Prone Code Segments
When encountering complex or error-prone code segments, developers often incorporate detailed comments explaining the intricacies of the logic. Such comments not only aid in understanding the code but also serve as red flags for potential issues. They can explicitly highlight edge cases, known limitations, or assumptions that the code makes, thereby drawing attention to areas that require careful scrutiny.
Consider the following Java code snippet:
// Check if the list is not empty and contains at least 3 elements
if (list != null && list.size() >= 3) {
// Perform operations on the first three elements
// ...
} else {
// Handle the case when the list is either null or contains less than 3 elements
// ...
}
In this example, the comments provide clarity on the conditions being checked and the actions taken based on those conditions. They also indicate the potential for errors if assumptions about the list's state are not met.
Using Comments to Highlight Temporary Workarounds
Temporary workarounds or quick fixes are often implemented in code to address urgent issues. While these solutions may suffice in the short term, they can introduce potential risks or technical debt. Comments play a crucial role in documenting such workarounds and flagging them for future resolution.
// TODO: Temporary fix to handle null pointer exception, revisit for a more robust solution
String result = (str != null) ? str.toLowerCase() : "";
In this snippet, the comment indicates that the current approach is a temporary fix and prompts developers to revisit the code for a more robust and permanent solution, thus preventing complacency towards addressing potential issues.
Documenting Unresolved Issues
In a collaborative development environment, it is common to come across code segments that have unresolved issues or pending tasks. By using comments to document such instances, developers can keep track of incomplete or ongoing work, ensuring that these issues are not overlooked or forgotten.
// FIXME: Handle case when the data retrieval API returns inconsistent results
int processData(Data data) {
// Logic to process the data
// ...
}
The FIXME
comment serves as a clear indicator that there is an unresolved issue related to the consistency of data retrieved from an API, prompting developers to revisit and address it.
Best Practices for Writing Code Comments for Error Indication
Incorporating code comments for error indication should align with best practices to ensure they effectively serve their purpose. Here are some recommendations for writing informative and actionable comments to highlight potential issues within the codebase:
-
Be Specific and Clear: Comments should precisely state the nature of the potential error or issue, providing context for developers who encounter the code in the future.
-
Provide Rationale: Explain why a particular code segment is prone to errors or the reasons behind using a specific approach. This helps in understanding the underlying concerns and aids in formulating appropriate solutions.
-
Use Consistent Conventions: Follow a consistent and clear commenting style throughout the codebase to facilitate uniformity and ease of comprehension.
-
Avoid Redundancy: While it is important to document potential issues, refrain from over-commenting or stating the obvious, as it can clutter the code and dilute the significance of critical comments.
-
Update Comments Proactively: As the code evolves and issues are resolved, ensure that the associated comments are updated or removed accordingly to maintain their relevance and accuracy.
Key Takeaways
Code comments are not just aids for understanding the logic; they play a pivotal role in flagging potential errors and unresolved issues within the codebase. Developers should cultivate the habit of scrutinizing and leveraging code comments for error indication, thereby fortifying the robustness and reliability of their code.
By embracing best practices in writing informative and actionable comments, developers can establish a culture of proactive issue identification and resolution, ultimately contributing to the overall quality and maintainability of the code.
Incorporating code comments for error indication is a small yet impactful step towards fostering a more resilient and error-resistant codebase. It is a practice that empowers developers to preemptively tackle potential issues, thereby elevating the overall software development process to new levels of efficiency and reliability.
So, the next time you encounter a verbose comment in the code, remember that it might be more than just an explanation – it could be a valuable heads-up for an underlying issue waiting to be addressed.
Ensure you make the most out of code comments, not just for understanding, but also for error indication.