The Challenges of Self-Organizing Teams
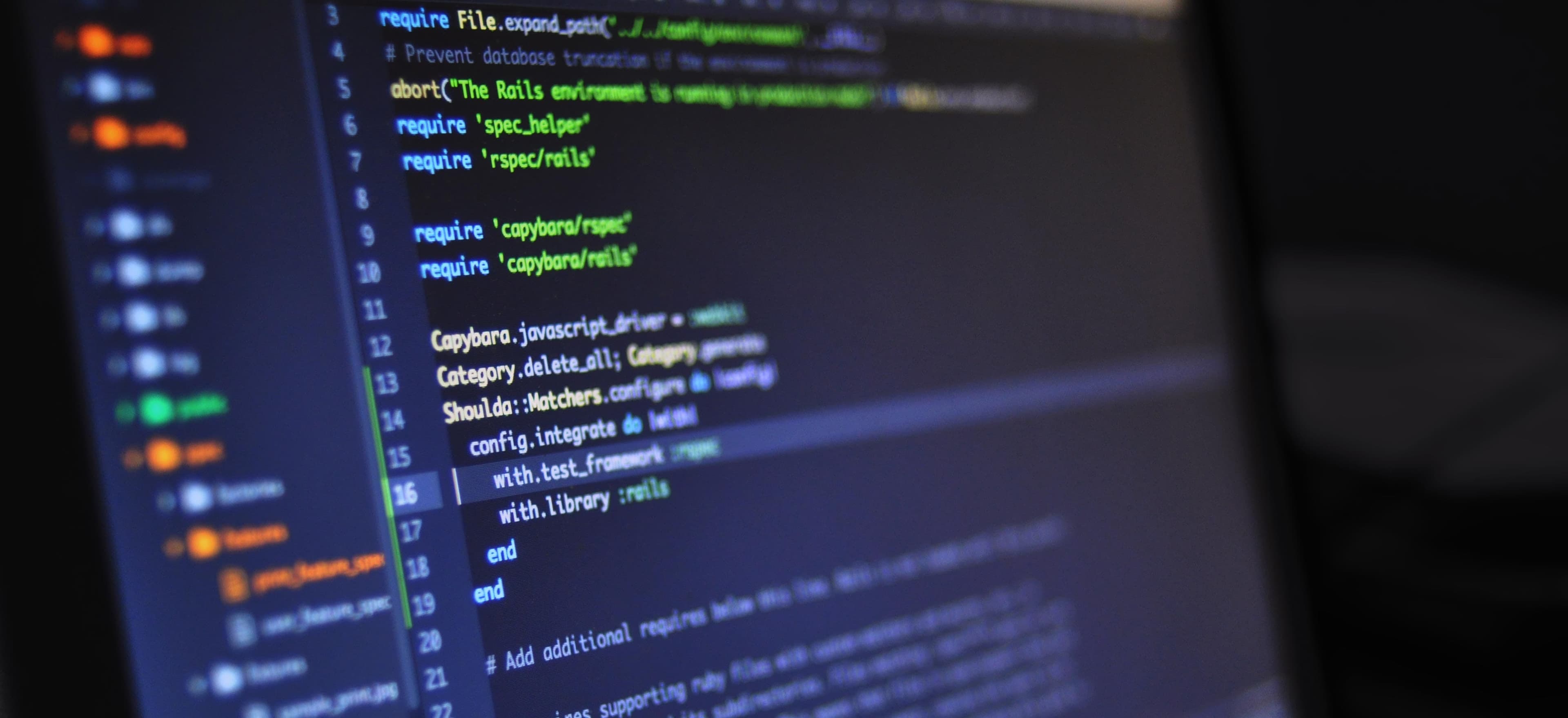
- Published on
The Challenges of Self-Organizing Teams
In the world of software development, self-organizing teams have gained prominence as a means to improve agility and creativity. These teams are characterized by their ability to make independent decisions, manage their own workload, and adapt to changing requirements. While this approach offers many benefits, it is not without its challenges. In this article, we will explore the unique difficulties faced by self-organizing teams and discuss how Java can be used to address them.
Understanding Self-Organizing Teams
Self-organizing teams are empowered to take ownership of their work and are responsible for making decisions to achieve their goals. This level of autonomy can lead to increased motivation, creativity, and accountability. However, it also introduces a set of challenges that traditional teams may not encounter.
Challenge 1: Lack of Guidance
Without a predefined structure, self-organizing teams may struggle with a lack of guidance. In traditional environments, there are often clear hierarchies and designated leaders who provide direction. In contrast, self-organizing teams must rely on shared decision-making and collaboration to set their own course.
Java Solution:
With Java, clear and consistent coding standards can help provide a level of guidance in the absence of a formal hierarchy. By establishing coding conventions and best practices, teams can ensure that all members are aligned in their approach, despite the absence of explicit directives.
// Example of coding standard in Java
public class MyClass {
private int myVariable; // Example of variable naming convention
public void myMethod() { // Example of method naming convention
// Method implementation
}
}
Challenge 2: Communication and Coordination
Effective communication and collaboration are essential for self-organizing teams. However, without a central authority dictating tasks and responsibilities, team members must proactively coordinate their efforts and stay informed about the progress of others.
Java Solution:
Using Java's multithreading capabilities, teams can develop concurrent and parallel programs to facilitate real-time communication and coordination. By leveraging constructs such as Thread
and ExecutorService
, teams can synchronize their activities and exchange information efficiently.
// Example of multithreading in Java
public class MyThread extends Thread {
public void run() {
// Thread execution logic
}
}
// Creating and starting a thread
MyThread thread = new MyThread();
thread.start();
Challenge 3: Adapting to Change
Self-organizing teams must be agile and adaptable to respond to changing requirements. In dynamic environments, this can be particularly challenging, as there may be limited mechanisms in place to manage and prioritize incoming changes.
Java Solution:
By embracing agile development methodologies supported by Java, such as Scrum or Extreme Programming, teams can implement iterative and incremental processes that embrace change. Additionally, integrating testing frameworks like JUnit can enable teams to confidently refactor and modify code without compromising its integrity.
// Example of using JUnit for testing in Java
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyTest {
@Test
public void myTestMethod() {
// Test logic and assertions
assertEquals(2, 1 + 1);
}
}
To Wrap Things Up
Self-organizing teams offer a unique approach to software development, enabling autonomy and flexibility. However, they also present specific challenges that require careful consideration and strategic solutions. By harnessing the capabilities of Java, teams can address these challenges effectively, ensuring that their self-organizing approach remains productive and sustainable in the long run.