Optimizing Instance Methods for Enums in Kotlin
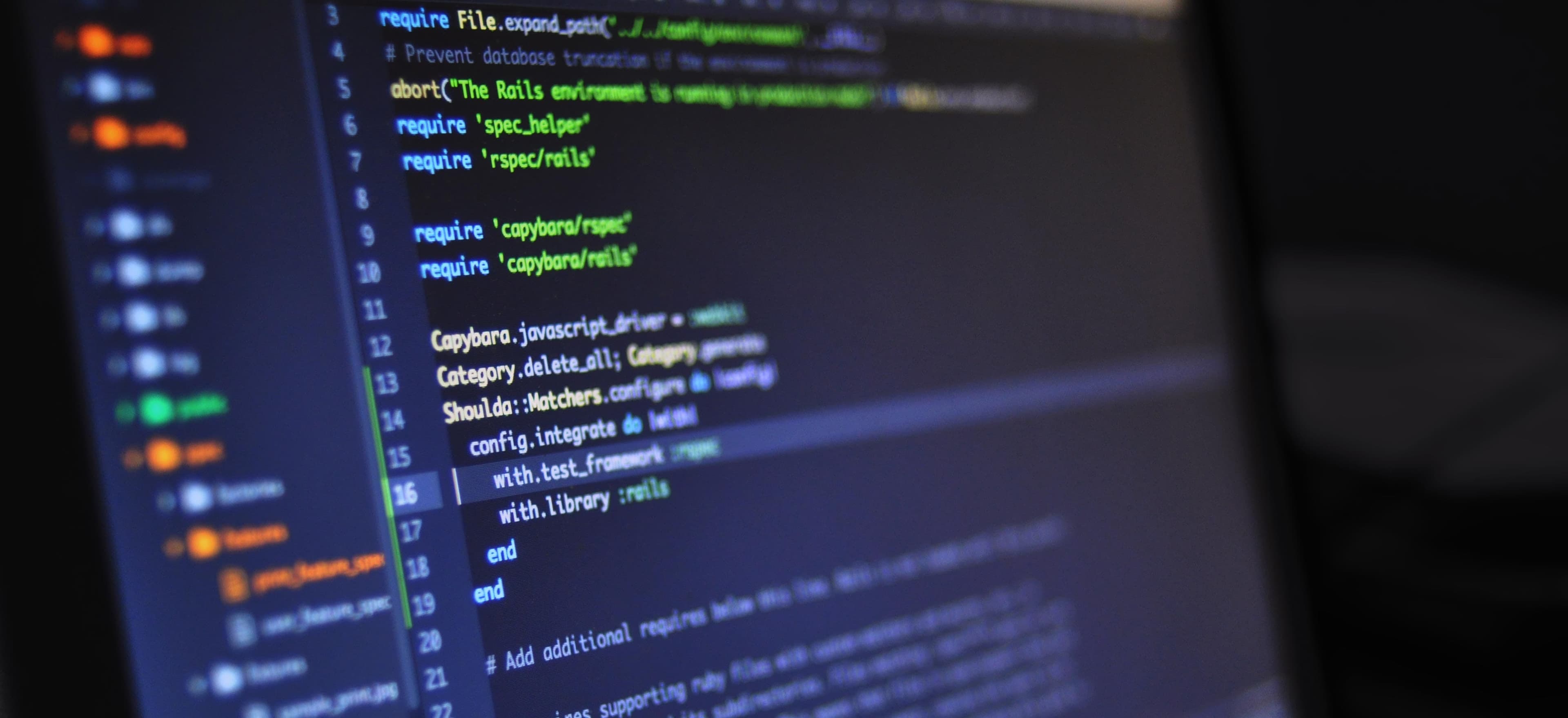
- Published on
Optimizing Instance Methods for Enums in Kotlin
When working with enums in Kotlin, it's essential to write efficient and optimized code. Enums are essentially classes in Kotlin, and like any other class, they can have instance methods. However, to ensure that these instance methods are optimized, there are some best practices and techniques that developers can employ. In this post, we will explore how to optimize instance methods for enums in Kotlin, ensuring that the code is not only clean and readable but also performs efficiently.
Understanding Enums in Kotlin
In Kotlin, enums are used to model a fixed set of options. They are defined using the enum
keyword and can contain properties and methods. Each enum constant is an object, and they are separated by commas. Enums in Kotlin can also have constructors, as well as initialize and anonymous classes.
enum class Direction {
NORTH,
SOUTH,
EAST,
WEST;
fun isCardinalDirection(): Boolean {
return this == NORTH || this == SOUTH || this == EAST || this == WEST
}
}
In the above example, we have defined an enum class Direction
with four constants representing the cardinal directions. We have also defined an instance method isCardinalDirection
to check if the direction is a cardinal direction.
Optimizing Instance Methods
Now that we understand the basics of enums in Kotlin, let's delve into optimizing instance methods for enums.
Use when
Expression for Concise Code
When dealing with enums, it's common to use a when
expression to branch the code based on the enum constant. This can lead to a more concise and readable code compared to using multiple if
statements.
enum class Direction {
NORTH,
SOUTH,
EAST,
WEST;
fun isCardinalDirection(): Boolean {
return when (this) {
NORTH, SOUTH, EAST, WEST -> true
else -> false
}
}
}
Using a when
expression makes it clear that we are branching based on the enum constant values. This approach not only makes the code more readable but also can improve performance due to its optimized implementation in Kotlin.
Leverage const
Keyword for Performance
In Kotlin, the const
keyword can be used to make the enum constant values compile-time constants. This can lead to improved performance as it enables the compiler to optimize the code by substituting the constant values directly where they are used.
enum class Direction {
NORTH,
SOUTH,
EAST,
WEST;
companion object {
private const val NUM_CARDINAL_DIRECTIONS = 4
}
fun isCardinalDirection(): Boolean {
return when (this) {
NORTH, SOUTH, EAST, WEST -> true
else -> false
}
}
}
In the above example, we have defined a const
property NUM_CARDINAL_DIRECTIONS
within the companion object. Using const
ensures that the value is known at compile time, potentially resulting in better performance when the code is executed.
Consider Using sealed
Classes for Exhaustive Checks
If you need to perform exhaustive checks on enum constants, consider using sealed
classes. Sealed classes in Kotlin are restricted hierarchies, which can be used to perform exhaustive checks without needing an else
branch. This can lead to better-optimized code, especially when the sealed class is used in combination with when
expressions.
sealed class Direction {
object NORTH : Direction()
object SOUTH : Direction()
object EAST : Direction()
object WEST : Direction()
fun isCardinalDirection(): Boolean {
return when (this) {
NORTH, SOUTH, EAST, WEST -> true
}
}
}
In the above example, we have defined a sealed class Direction
with four object instances representing the cardinal directions. When using sealed
classes, the use of when
expressions for exhaustive checks can result in optimized code, as the compiler knows that all possible subclasses are covered.
A Final Look
Optimizing instance methods for enums in Kotlin is key to writing efficient and maintainable code. By leveraging techniques such as using when
expressions, const
keyword, and sealed
classes, developers can ensure that their enum instance methods are not only optimized but also adhere to best practices. Writing optimized code for enums can lead to improved performance and a more robust codebase overall. By following these best practices, developers can ensure that their Kotlin enums perform efficiently while remaining concise and readable.
In conclusion, optimizing instance methods for enums in Kotlin is not just about improving performance, but also about writing clean, maintainable, and efficient code.
Remember, optimizing code is not about premature optimization, but about thoughtful and strategic improvements that result in better-performing, more maintainable code.
Happy coding!
Note: While the examples in this post are in Kotlin, the optimization principles can often be applied to other languages with enum support.
For further reading on enums in Kotlin, you can check the official Kotlin documentation.
That’s a wrap for this post! If you have any questions or additional tips for optimizing enum instance methods in Kotlin, feel free to share in the comments.