Improving Hibernate Performance with Hazelcast Cache
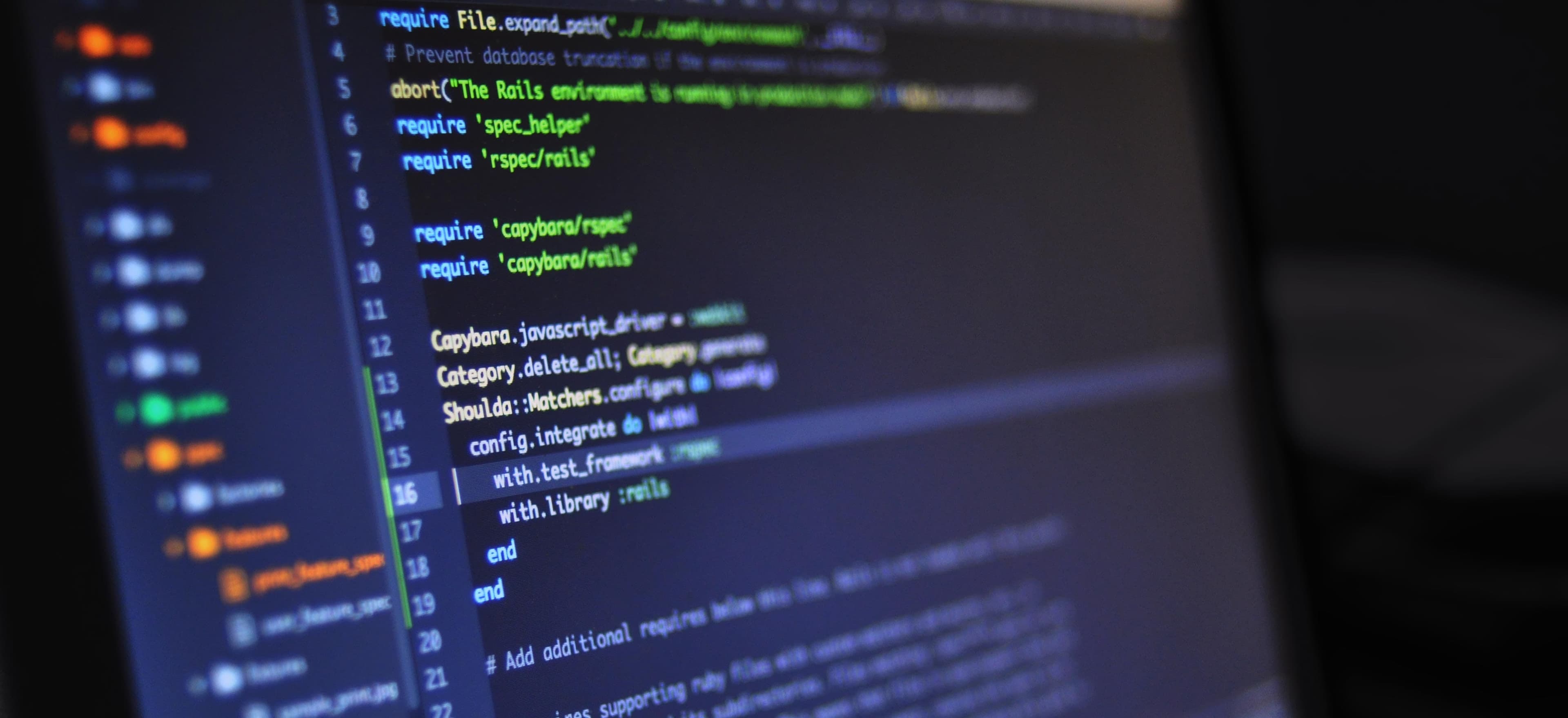
- Published on
Improving Hibernate Performance with Hazelcast Cache
When working with Java applications that utilize Hibernate for database access, you might encounter performance bottlenecks due to frequent database queries. This can lead to increased response times and decreased overall application performance. One effective solution to this problem is to integrate a distributed caching mechanism like Hazelcast with Hibernate. In this article, we will explore how to improve Hibernate performance by using Hazelcast as a second-level cache.
Understanding Hibernate Second-Level Caching
Hibernate is a powerful and popular Object-Relational Mapping (ORM) framework for Java. One of its key features is its support for second-level caching, which can significantly improve performance by reducing the number of times an application needs to hit the database. Second-level caching is particularly effective for read-heavy applications, where the same data is queried frequently.
Introducing Hazelcast
Hazelcast is an open-source, in-memory data grid that provides distributed data storage and computation. It can be used as a standalone caching solution or integrated with other frameworks like Hibernate to improve application performance.
Integrating Hibernate with Hazelcast
To integrate Hibernate with Hazelcast, you need to add the necessary dependencies to your project. Below is a Maven configuration that adds the Hibernate and Hazelcast dependencies to your project:
<dependencies>
<!-- Hibernate dependencies -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.18.Final</version>
</dependency>
<!-- Hazelcast dependencies -->
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast</artifactId>
<version>4.2.1</version>
</dependency>
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast-hibernate5</artifactId>
<version>2.1.0</version>
</dependency>
</dependencies>
Once the dependencies are added, you can configure Hibernate to use Hazelcast as the second-level cache provider. Below is an example of Hibernate configuration in hibernate.cfg.xml
:
<property name="hibernate.cache.region.factory_class">com.hazelcast.hibernate.HazelcastCacheRegionFactory</property>
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.use_query_cache">true</property>
In this configuration, we configure Hibernate to use Hazelcast as the second-level cache provider and enable both the second-level cache and query cache.
Understanding the Benefits of Hazelcast Cache for Hibernate
Integrating Hazelcast with Hibernate brings several benefits:
1. Reduced Database Load
Hazelcast provides an in-memory cache that can store frequently accessed data. By caching database entities in memory, it reduces the number of database queries, leading to a reduced database load and improved application performance.
2. Scalability
Hazelcast is a distributed caching solution, meaning it can be easily scaled by adding more nodes to the cluster. This enables the cache to handle larger datasets and more concurrent access, further enhancing the performance of the application.
3. Fault Tolerance
Hazelcast provides built-in support for high availability and fault tolerance. In case a node in the cluster goes down, the data is automatically rebalanced across the remaining nodes, ensuring continuous access to cached data.
Best Practices for Using Hazelcast Cache with Hibernate
While integrating Hazelcast with Hibernate can bring significant performance improvements, it's essential to follow best practices to ensure effective and efficient usage of the cache.
1. Identify Cacheable Entities
Identify the entities in your Hibernate application that are read frequently and can benefit from caching. Not all entities need to be cached, so focus on those that are frequently accessed and not frequently updated.
2. Proper Cache Configuration
Configure the cache settings appropriately based on the access patterns and data size. This includes setting eviction policies, time-to-live, and maximum size of entries to be cached. These settings ensure that the cache stays efficient and doesn't consume excessive memory.
3. Monitor and Tune the Cache
Monitor the cache usage and performance to identify any bottlenecks or inefficiencies. Tune the cache configuration based on the observed behavior, such as adjusting eviction policies or adding more nodes to the cluster for better distribution.
To Wrap Things Up
Integrating Hazelcast with Hibernate as a second-level cache can greatly enhance the performance of your Java applications by reducing database load, improving scalability, and providing fault tolerance. By following best practices for using the cache effectively, you can ensure that your application benefits from the performance gains offered by this powerful combination.
In conclusion, if you're looking to boost the performance of your Hibernate-based Java application, consider leveraging the capabilities of Hazelcast to introduce a robust and efficient caching layer.
Give it a try and experience the performance improvements firsthand!
External Links
- Hibernate Official Website
- Hazelcast Official Website
- Hazelcast Documentation
- Hazelcast Hibernate Integration Documentation
- Hibernate Caching Strategies
Now that you're equipped with the knowledge of improving Hibernate performance with Hazelcast cache, go ahead and optimize the performance of your applications!