Improving NullPointerException Messages in JDK 15
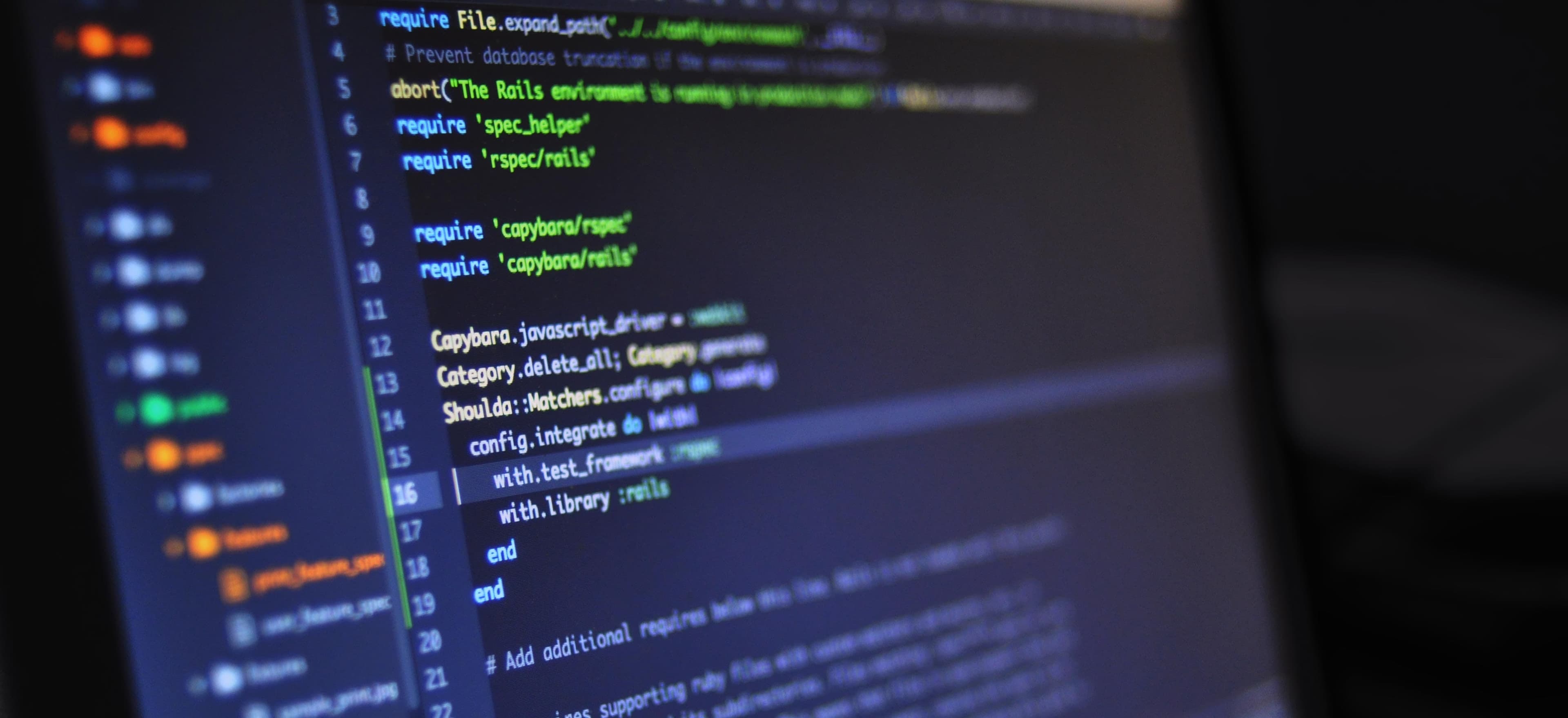
- Published on
Understanding and Leveraging the Enhanced NullPointerException Messages in Java JDK 15
In JDK 15, Java developers have been bestowed with an enhancement that may seem small but holds great potential in terms of debugging and improving code quality - the improved NullPointerException messages. NullPointerException is a ubiquitous exception in Java programming, often indicating a flaw in the code. With its vague error message, pinpointing the root cause of the issue becomes a daunting task.
The Frustration with NullPointerException
Consider the following scenario, wherein a NullPointerException is encountered:
String name = null;
int length = name.length();
Upon running the code, Java throws a NullPointerException with the message java.lang.NullPointerException
. This minimalist message provides no insight into which variable caused the exception or where the issue is located.
JDK 15 to the Rescue
Recognizing the need to elevate the precision of these error messages, the JDK 15 introduces an informative and discerning NullPointerException message: Cannot invoke "String.length()" because "name" is null
. This message pinpoints the exact operation (String.length()
) that caused the exception and the variable (name
) responsible for it.
Why It Matters
The enhanced NullPointerException message equips developers with valuable information, expediting the process of identifying and rectifying faulty code. By offering concise, clear, and actionable insights, it significantly diminishes debugging time and amplifies the overall development experience.
Utilizing the Enhanced NullPointerException Messages
Let's delve into a practical illustration to understand the significance of the enhanced NullPointerException messages and how to leverage them effectively.
Consider the following code snippet:
public class UserDetails {
private String name;
public UserDetails(String name) {
this.name = name;
}
public int getNameLength() {
return this.name.length();
}
public static void main(String[] args) {
UserDetails userDetails = new UserDetails(null);
System.out.println(userDetails.getNameLength());
}
}
In this scenario, without the enhanced NullPointerException messages (pre-JDK 15), the exception message would have been opaque and uninformative. However, with the improved exception message in JDK 15, the error message becomes elucidative and actionable. It clearly articulates the cause of the exception and the location of the issue, expediting the debugging process.
Leverage for Enhanced Debugging
By embracing the precise information offered by the enhanced NullPointerException messages, developers can swiftly locate and resolve issues, thereby streamlining the development cycle and bolstering code quality.
Compatibility and Adoption Considerations
It’s crucial to note that while JDK 15 offers this enhancement, older versions of Java will not provide the same level of NullPointerException message clarity. Hence, for projects targeting JDK 15 and above, this feature becomes a potent asset for refining code quality. However, for projects constrained to earlier JDK versions, the conventional vague NullPointerException messages persist.
Embracing Best Practices for NullPointerException Handling
While the improved NullPointerException messages undeniably offer significant assistance in debugging, it's imperative to adhere to best practices to circumvent NullPointerException scenarios altogether. Employing techniques such as defensive programming, null checks, and leveraging the Optional class can prevent the occurrence of NullPointerException, thereby fortifying the robustness of the codebase.
Defensive Programming
Embracing defensive programming practices, such as validating inputs, asserting preconditions, and employing defensive checks, aids in forestalling NullPointerException instances.
Leveraging Optional Class
The Optional class provides an elegant and concise way to handle potential null values, mitigating the risk of encountering NullPointerException. Its fluent API facilitates streamlined null checks and anticipatory actions, offering a proactive approach to null value handling.
In Conclusion, Here is What Matters
In conclusion, the enhanced NullPointerException messages in JDK 15 present a substantial advancement in fortifying the debugging process for Java developers. By imparting detailed and explicit information about the cause of the exception, it expedites issue resolution and bolsters code quality. While embracing this feature, it's imperative to amalgamate robust coding practices to preempt NullPointerException occurrences and reinforce the resilience of the codebase.
By leveraging both the enhanced NullPointerException messages and best practices in null value handling, Java developers can elevate their code quality, streamline debugging efforts, and fortify the overall development process.
We encourage you to explore the detailed documentation on NullPointerException and the robust features of JDK 15.
Invest in enhanced code quality and expedited debugging with the improved NullPointerException messages in JDK 15. Fortify your development process and empower your team with precision in error detection. Elevate your code, elevate your development.
With the enhanced NullPointerException messages in JDK 15, preempt errors, expedite resolutions, and fortify your code. Discover how this enhancement can revolutionize your development process.