Navigating SOAP Web Services in Modern Enterprise Integration
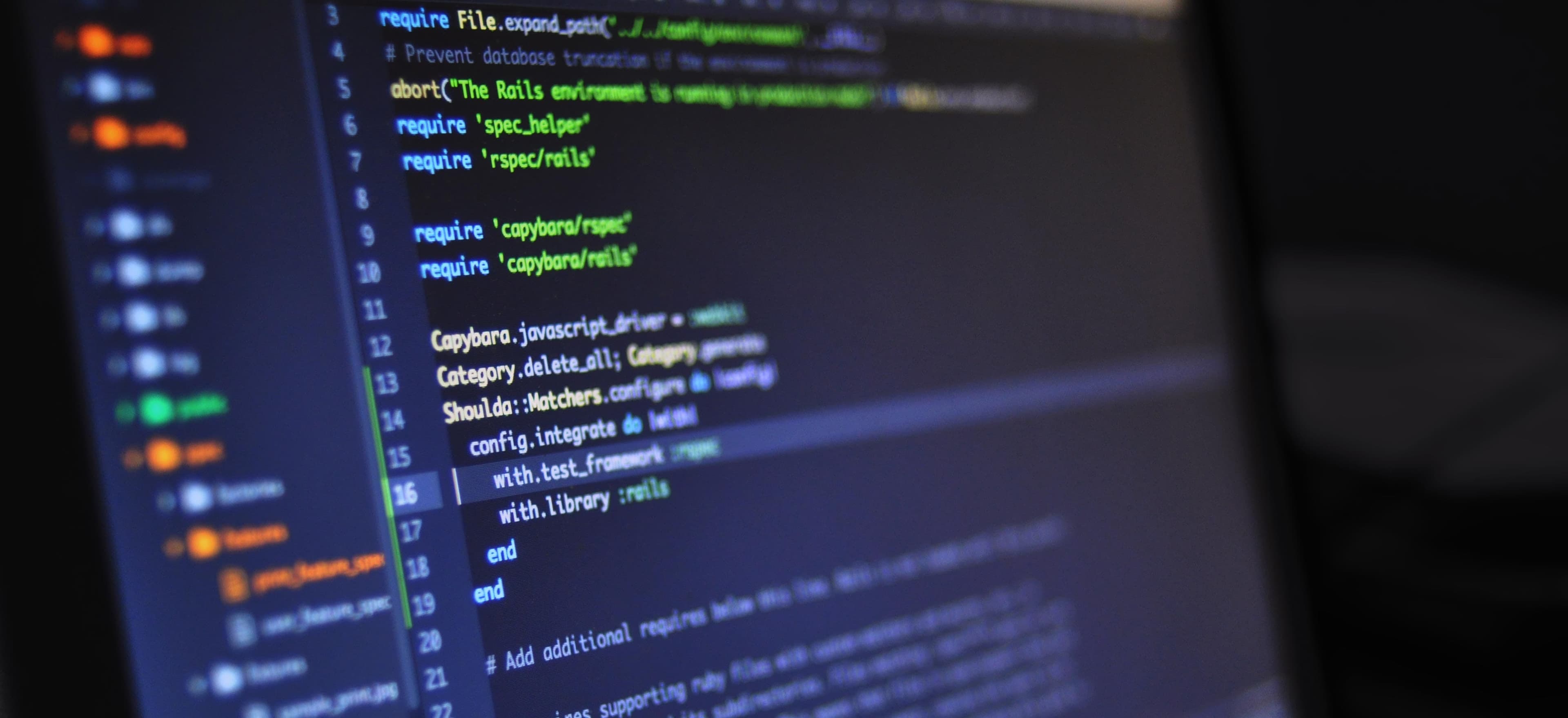
- Published on
Navigating SOAP Web Services in Modern Enterprise Integration
In the world of enterprise integration, there are various technologies that facilitate communication between different systems. One of the widely used technologies for web services is SOAP (Simple Object Access Protocol). In this blog post, we will delve into the world of SOAP web services, exploring what they are, how they work, and how they fit into modern enterprise integration.
Understanding SOAP Web Services
SOAP is a protocol that enables the exchange of structured information in the implementation of web services. It relies on XML for message formatting and provides a way for applications to communicate over the internet. SOAP web services typically use HTTP or HTTPS as the communication protocol and define a set of rules for structuring messages.
When working with SOAP web services, it's essential to understand the core components:
- SOAP Envelope: Encapsulates the entire XML document.
- SOAP Header: Contains header information.
- SOAP Body: Carries the actual message.
- SOAP Fault: Used for reporting errors.
Integrating SOAP Web Services with Java
Java provides robust support for working with SOAP web services. The JAX-WS (Java API for XML Web Services) is the standard Java API for building and consuming SOAP web services.
Let's consider a scenario where we need to consume a SOAP web service in a Java application. We'll use the wsimport
tool, which is part of the JDK, to generate the necessary Java artifacts from the WSDL (Web Services Description Language) of the web service.
// Generated by wsimport
@WebServiceClient(name = "MyWebService", targetNamespace = "http://example.com/mywebservice", wsdlLocation = "http://example.com/mywebservice?wsdl")
public class MyWebService extends Service {
@WebEndpoint(name = "MyWebServicePort")
public MyWebServicePortType getMyWebServicePort() {
return super.getPort(new QName("http://example.com/mywebservice", "MyWebServicePort"), MyWebServicePortType.class);
}
}
In the code snippet above, we see the generated client stub for the SOAP web service. The wsimport
tool has produced the necessary Java classes based on the WSDL, making it easier to work with the web service.
Building a SOAP Web Service Client in Java
Now, let's dive into the process of building a SOAP web service client using JAX-WS in Java. We'll walk through the steps, starting from creating a client, consuming the web service, and handling the response.
Step 1: Create a Client
First, we need to create a client for the web service using the generated artifacts.
MyWebServiceClient {
public static void main(String[] args) {
MyWebService service = new MyWebService();
MyWebServicePortType port = service.getMyWebServicePort();
// Call methods on the port to consume the web service
}
}
Step 2: Consume the Web Service
Once we have the client and port, we can start making calls to the web service methods.
// Assuming there's a method called 'performAction' in the web service
String result = port.performAction(parameter1, parameter2);
Step 3: Handle the Response
We can now handle the response from the web service. This could involve processing the data or handling any potential errors.
System.out.println("Result: " + result);
Why SOAP Web Services?
As the technology landscape continues to evolve, with the rise of RESTful APIs and microservices, one might wonder about the relevance of SOAP web services. While REST has gained popularity for its simplicity and flexibility, there are still scenarios where SOAP shines:
-
Stronger Standards: SOAP provides a more comprehensive set of standards for security, transactions, and addressing, making it suitable for enterprise-level integrations where strict standards are a requirement.
-
Tooling and Support: Java and other languages offer mature tooling and support for SOAP web services, making it easier to work with these services in enterprise environments.
-
Legacy Systems Integration: Many legacy systems are built on SOAP web services, and they continue to play a critical role in enterprise systems. Knowing how to work with SOAP web services is invaluable in integrating with such systems.
Wrapping Up
In this blog post, we've taken a closer look at SOAP web services in the context of modern enterprise integration. We explored the fundamentals of SOAP, its integration with Java using JAX-WS, and the reasons why it still holds significance in certain integration scenarios.
While RESTful services have become ubiquitous, SOAP web services remain a crucial part of the enterprise integration landscape. Understanding both technologies equips developers with the knowledge to tackle a wide range of integration challenges, ensuring the smooth interoperability of diverse systems in the enterprise environment.
By staying informed about different web service protocols and technologies, developers can make well-informed decisions when it comes to choosing the right approach for a given integration scenario.
So, whether you're diving into the world of enterprise integration or expanding your knowledge of web services, having a solid understanding of SOAP and its integration with Java is a valuable asset in your developer toolkit. Happy integrating!