Effective Software Version Control Strategies
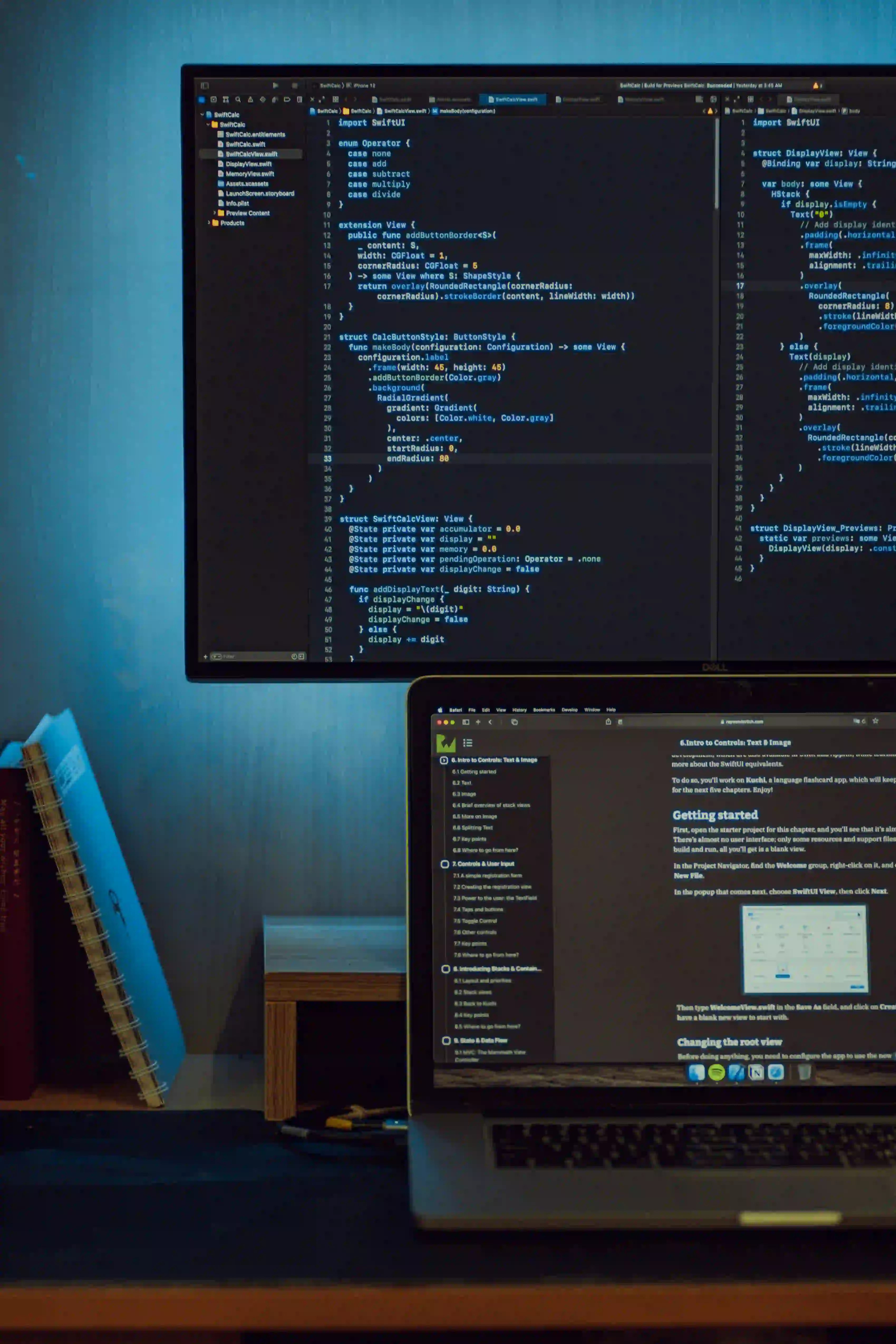
Ensuring Seamless Development with Effective Version Control in Java
Software development is an ever-evolving process that requires collaboration, organization, and precision. Version control is the backbone of this process, ensuring that developers can work concurrently without conflicts and have a steady record of changes. In this post, we'll delve into effective version control strategies for Java development, diving into essential practices and tools that streamline the development workflow.
Why Version Control Matters in Java Development
Without version control, Java projects can easily spiral into chaos. Version control systems provide a structured approach to managing code changes, allowing developers to:
- Track modifications and revert to previous versions.
- Collaborate seamlessly with team members.
- Maintain a clean and organized codebase.
Key Version Control Systems for Java
Git
Git has gained significant traction among Java developers due to its distributed architecture, speed, and flexibility. It enables efficient branching and merging, essential for concurrent Java development. Platforms like GitHub, GitLab, and Bitbucket provide robust hosting and collaboration features, making Git the go-to choice for Java projects.
Subversion
Subversion, also known as SVN, is a centralized version control system that remains prevalent in enterprise environments. While not as lightweight or speed-oriented as Git, SVN provides strong support for versioned directories and files, making it a viable option for Java projects that require strict access control and centralized management.
Best Practices for Version Control in Java Projects
1. Utilize Branching for Feature Isolation
Branching is a fundamental concept in version control, enabling developers to work on features or fixes without impacting the main codebase. In Java development, feature branches ensure that new functionalities can be developed independently and merged seamlessly once complete.
// Create a new feature branch
git checkout -b new-feature
// Make changes, commit, and push branch
git add .
git commit -m "Implement new feature"
git push origin new-feature
This approach prevents conflicts and maintains a clean history of changes, enhancing the overall stability of the Java codebase.
2. Continuous Integration with Version Control
Integrating version control with continuous integration (CI) pipelines streamlines the development process. Tools like Jenkins, CircleCI, and Travis CI can automatically trigger builds and tests upon code changes, ensuring that Java projects remain consistently functional and error-free. By integrating version control with CI, Java developers can catch and rectify issues early in the development lifecycle.
3. Meaningful Commit Messages
Clear, descriptive commit messages are pivotal for comprehending the evolution of a Java codebase. Meaningful commit messages should succinctly explain the purpose of the changes and provide context for future developers who may need to understand the rationale behind specific modifications.
git commit -m "Refactor user authentication logic to use JWT tokens"
By adhering to this practice, Java developers can ensure that all changes are transparent and comprehensible, fostering better collaboration and knowledge transfer within the team.
4. Code Reviews and Pull Requests
Code reviews are instrumental in maintaining code quality and knowledge sharing within a Java development team. By utilizing pull requests, developers can thoroughly scrutinize each other's code, identify potential issues, and suggest improvements before changes are merged into the main branch.
// Create a pull request via command line or Git hosting platform
git pull-request -m "Implement new feature X"
This collaborative approach enhances the overall robustness and maintainability of Java codebases while facilitating collective learning within the team.
5. Version Control for Configuration and Build Files
Version controlling configuration files, build scripts, and dependency specifications is indispensable for Java projects to ensure consistency across development environments and deployments. By managing these files within version control, Java developers can mitigate discrepancies and simplify the setup and replication of project environments.
The Closing Argument
Version control is a foundational aspect of modern software development, playing a pivotal role in the agility, collaboration, and reliability of Java projects. By embracing best practices and leveraging robust version control systems like Git and Subversion, Java developers can fortify their development workflows, mitigate risks, and ensure the seamless evolution of their codebases.
Implementing version control best practices, integrations, and collaborative processes empowers Java development teams to sustainably deliver high-quality software, ensuring that every line of code contributes to an exemplary end product. Striving for excellence in version control is, in essence, the gateway to streamlined, efficient, and harmonious Java development.
By incorporating the outlined version control strategies into Java projects, developers can navigate the intricacies of code evolution with finesse, ensuring that each iteration reflects the team's collective ingenuity and dedication to crafting exceptional software solutions.
In the dynamic realm of Java development, version control stands as an unwavering compass, guiding teams through the vast expanse of code changes, collaborative endeavors, and software evolution. With the right version control strategies in place, Java projects can soar to new heights, unfettered by the chaos of unmanaged modifications, and instead, propelled by the synergy of organized, purposeful development.